#React
Wordle game using ReactJS
Wordle is a word puzzle game where players guess a hidden word within a limited number of tries.
Prerequisites:
Basic knowledge of JavaScript and Rea...
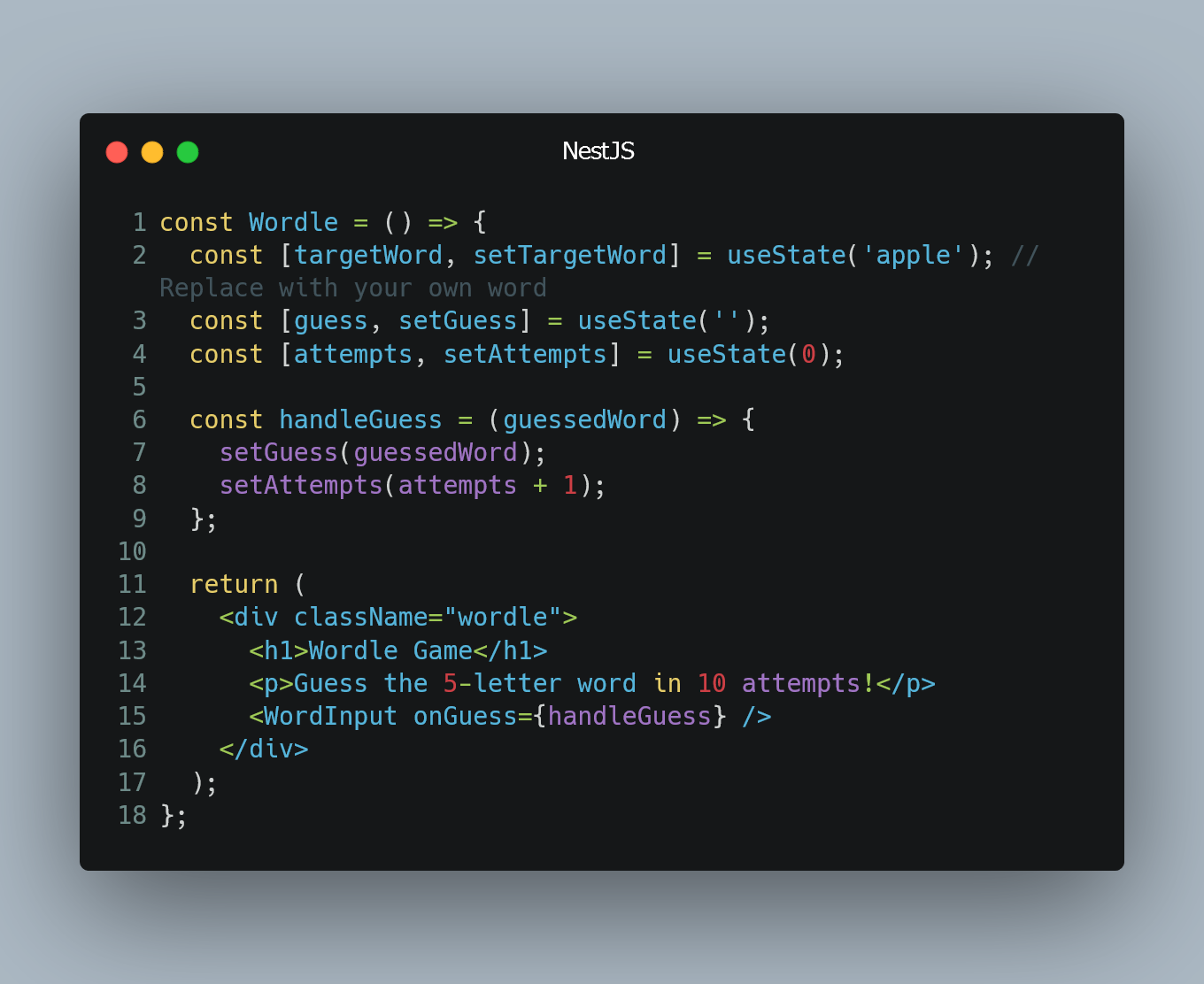
Adding Multiple Classes to a Component
In React, you can apply multiple CSS classes to a component to style it according to your design requirements.
#1. Using String Concatenation
One of t...
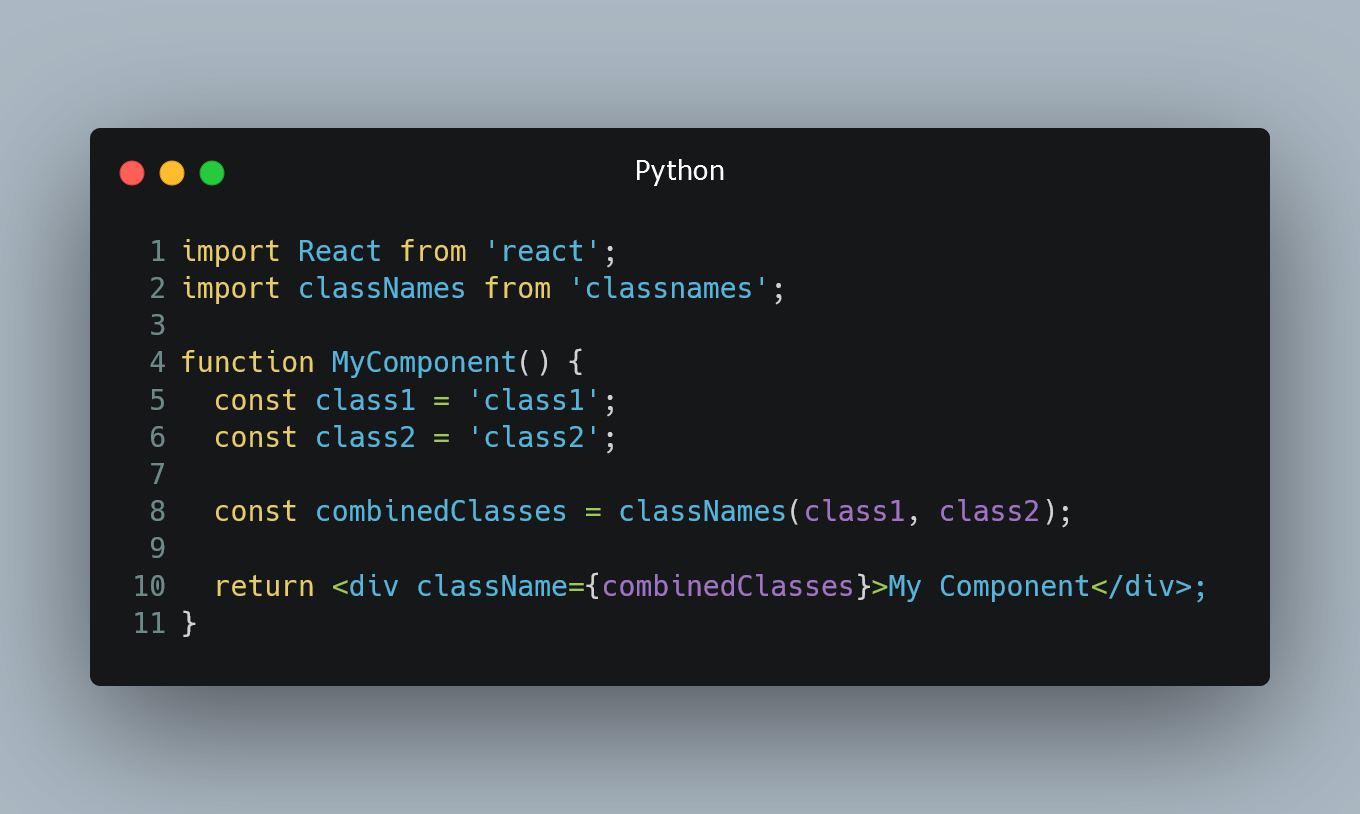
Programmatically Navigate Using React Router
React Router is a popular library for handling routing in React applications. It allows you to navigate between different components or pages based on...
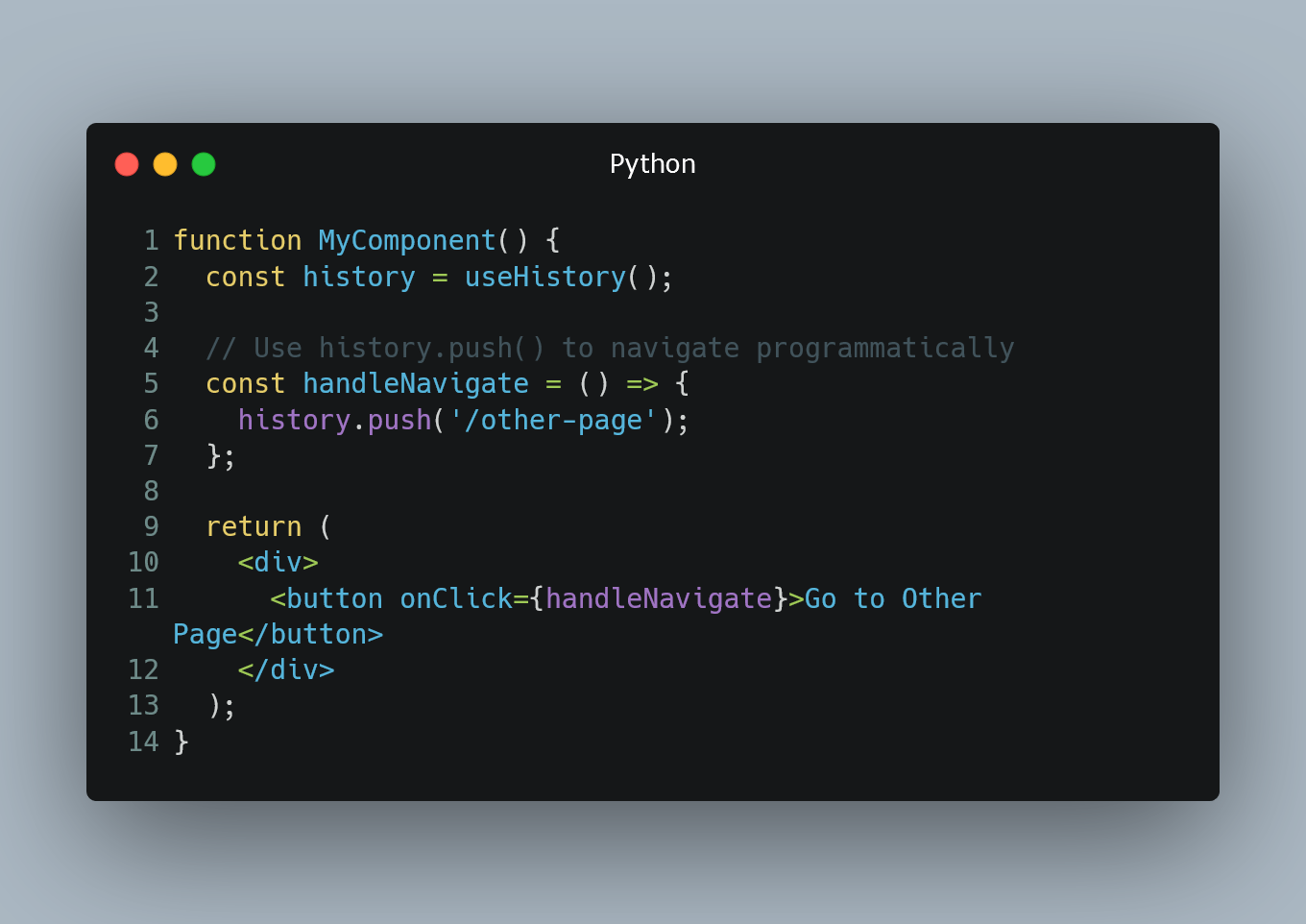
Understanding Unique Keys for Array Children
In React, when rendering arrays of elements as children, it is essential to provide a unique key prop for each child.
#1. Why Keys are Necessary
Keys...
Loop Inside React JSX
In React, you can use loops to dynamically render repetitive elements or content within JSX (JavaScript XML).
#1. Using map() to Loop
One of the most...
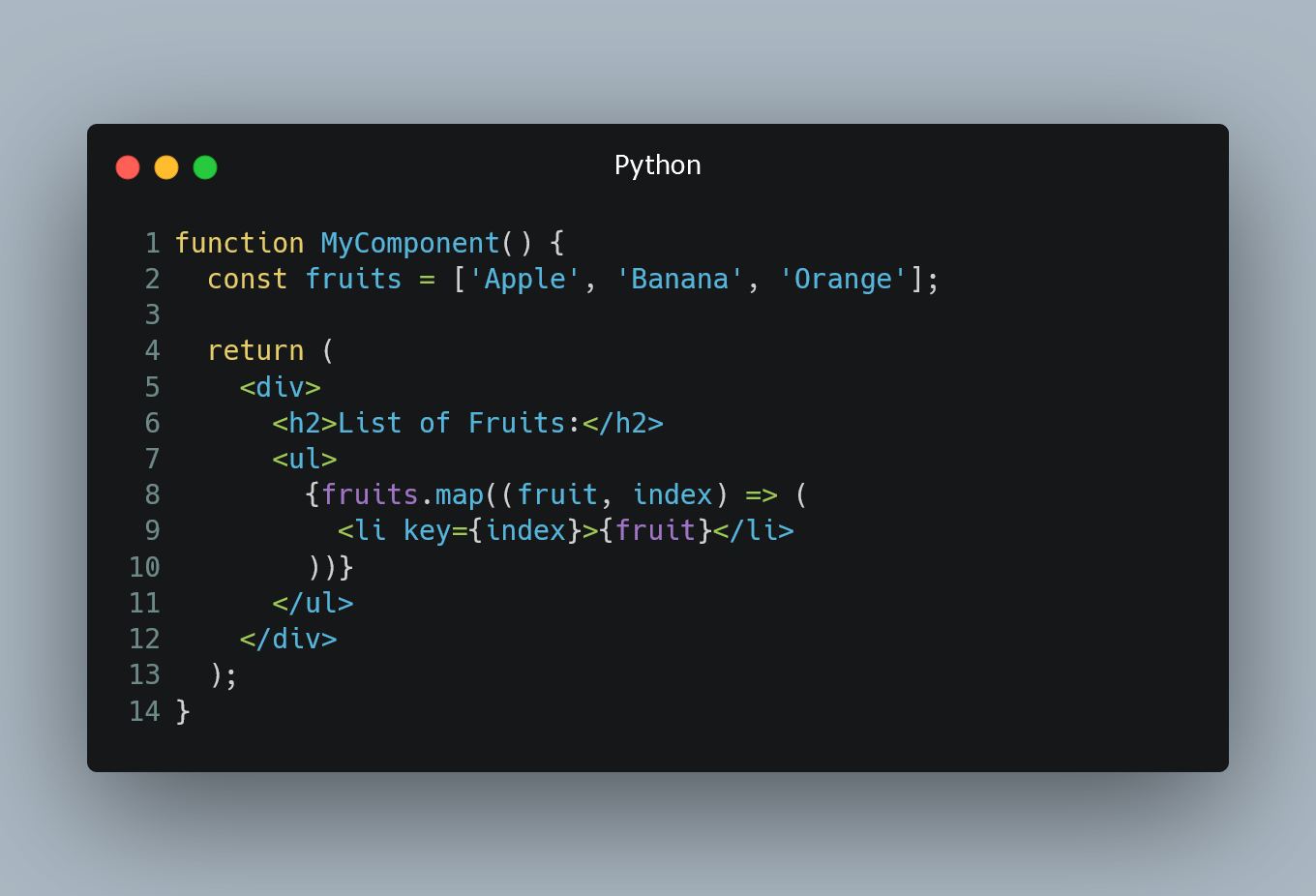
Show or Hide Element
In React, you can dynamically show or hide elements based on certain conditions.
#1. Using Conditional Rendering with JavaScript if statement
One of t...
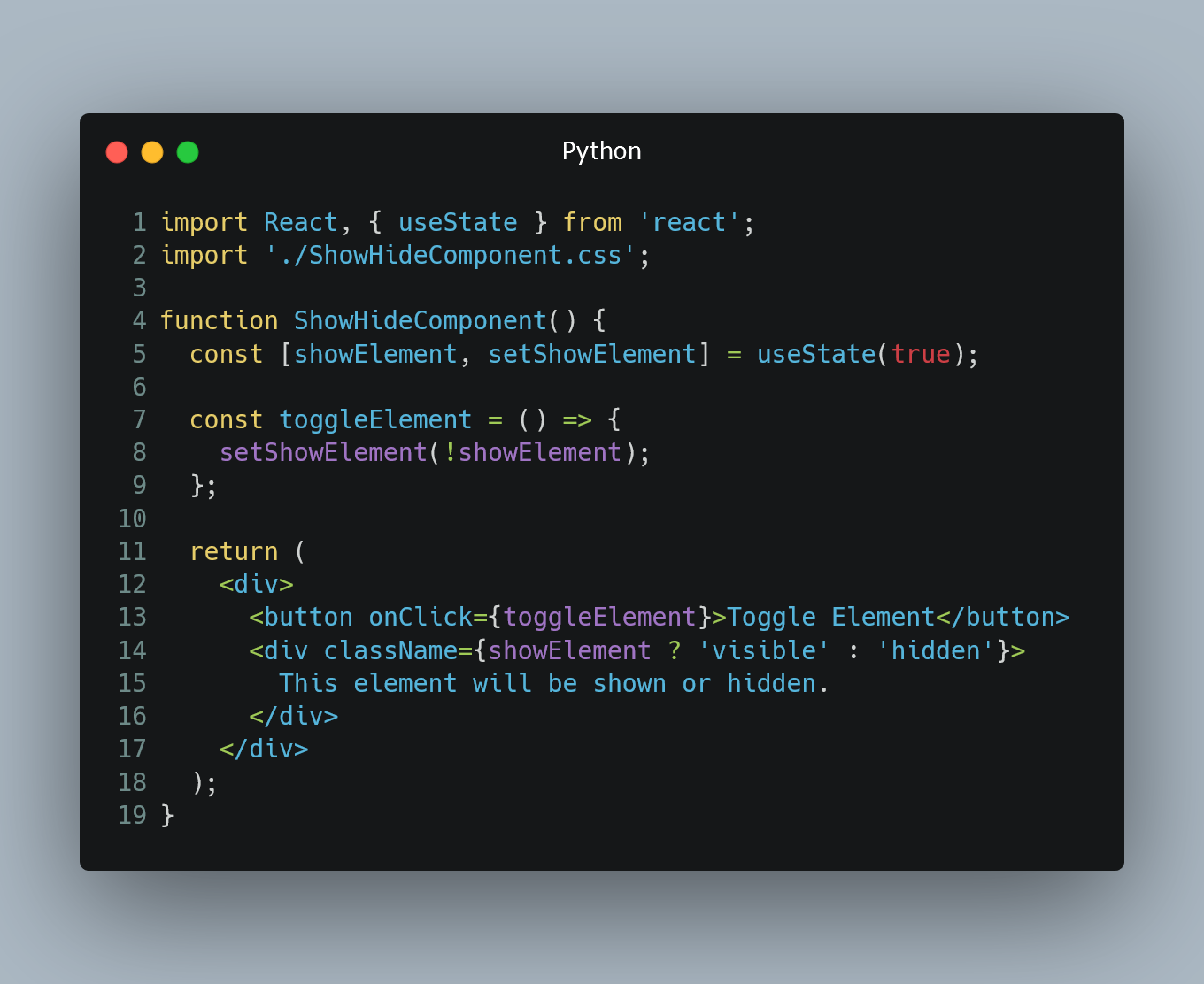
Forcing a React Component to Rerender Without Calling setState
In React, components rerender automatically when their state or props change. However, there may be cases where you want to force a component to reren...
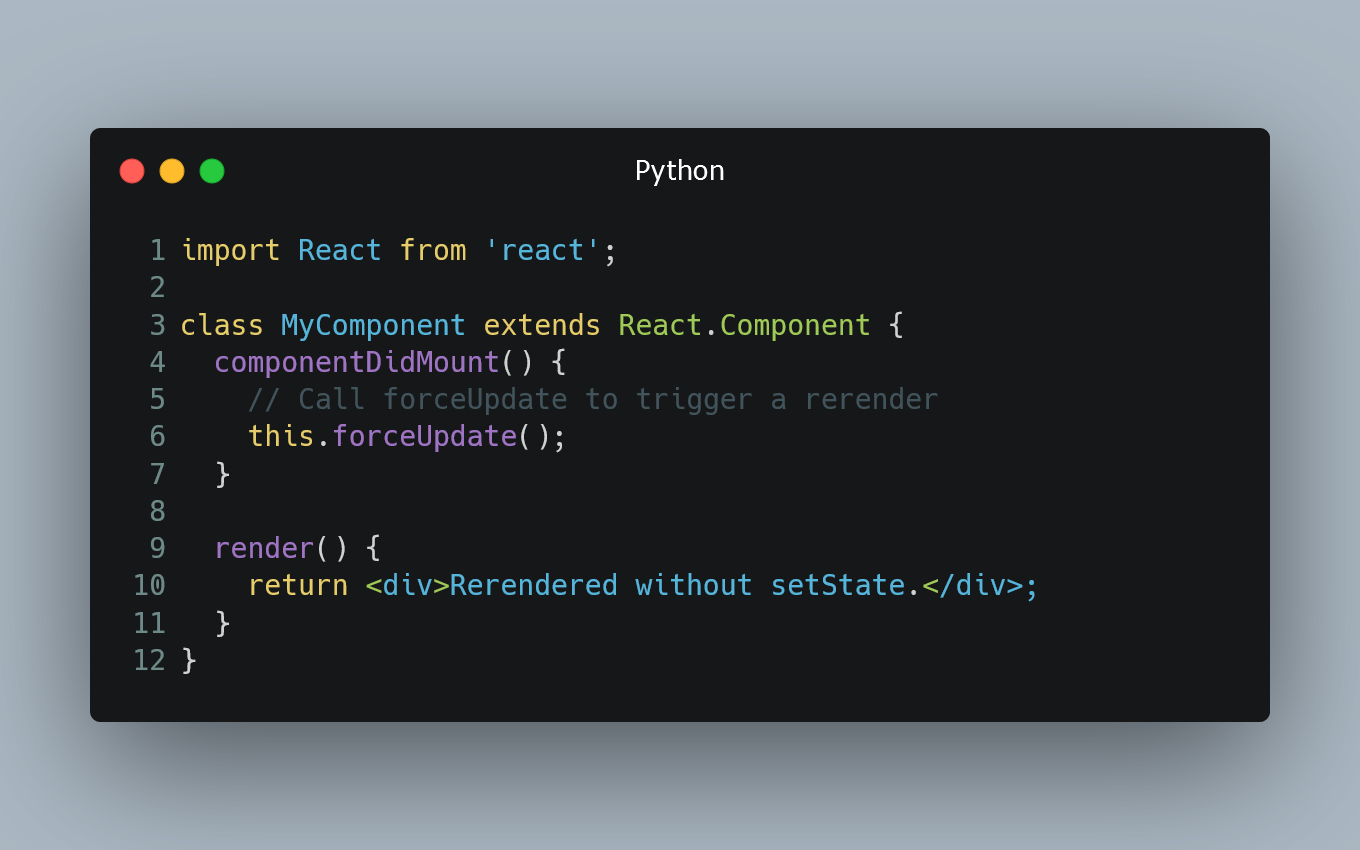
Call Child Method from Parent
In React, it is possible for a parent component to call a method defined in a child component.
#1. Use Ref to Access Child Component
To call a child m...
Getting Parameter Value from Query String
In web development, query strings are used to pass data in the URL. These query strings can contain parameters and their values.
#1. Using URLSearchPa...
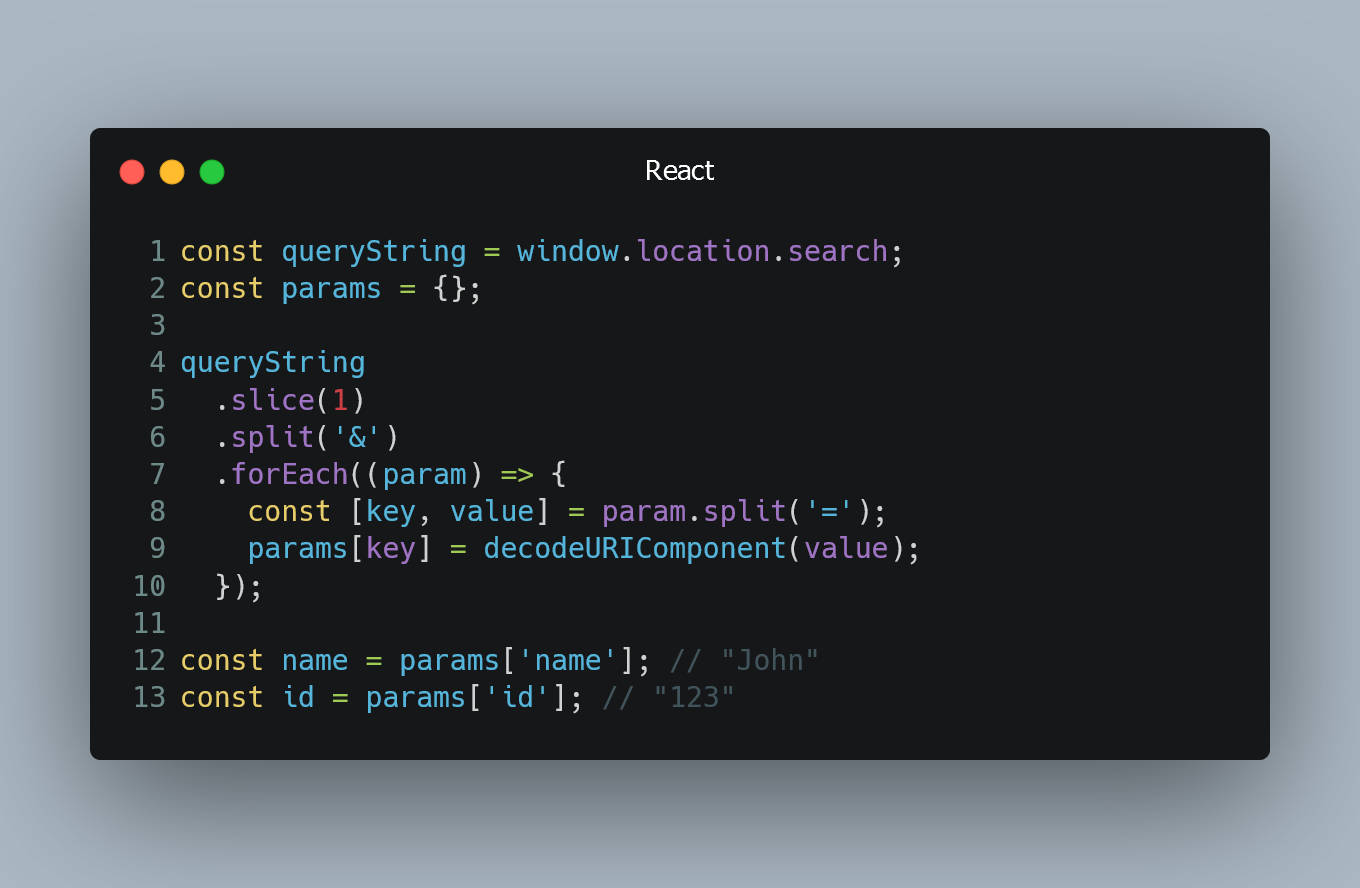
Detect Click Outside React Component
In React, you may encounter situations where you need to detect when a user clicks outside a specific component, such as a dropdown, modal, or popover...