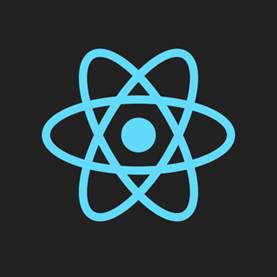
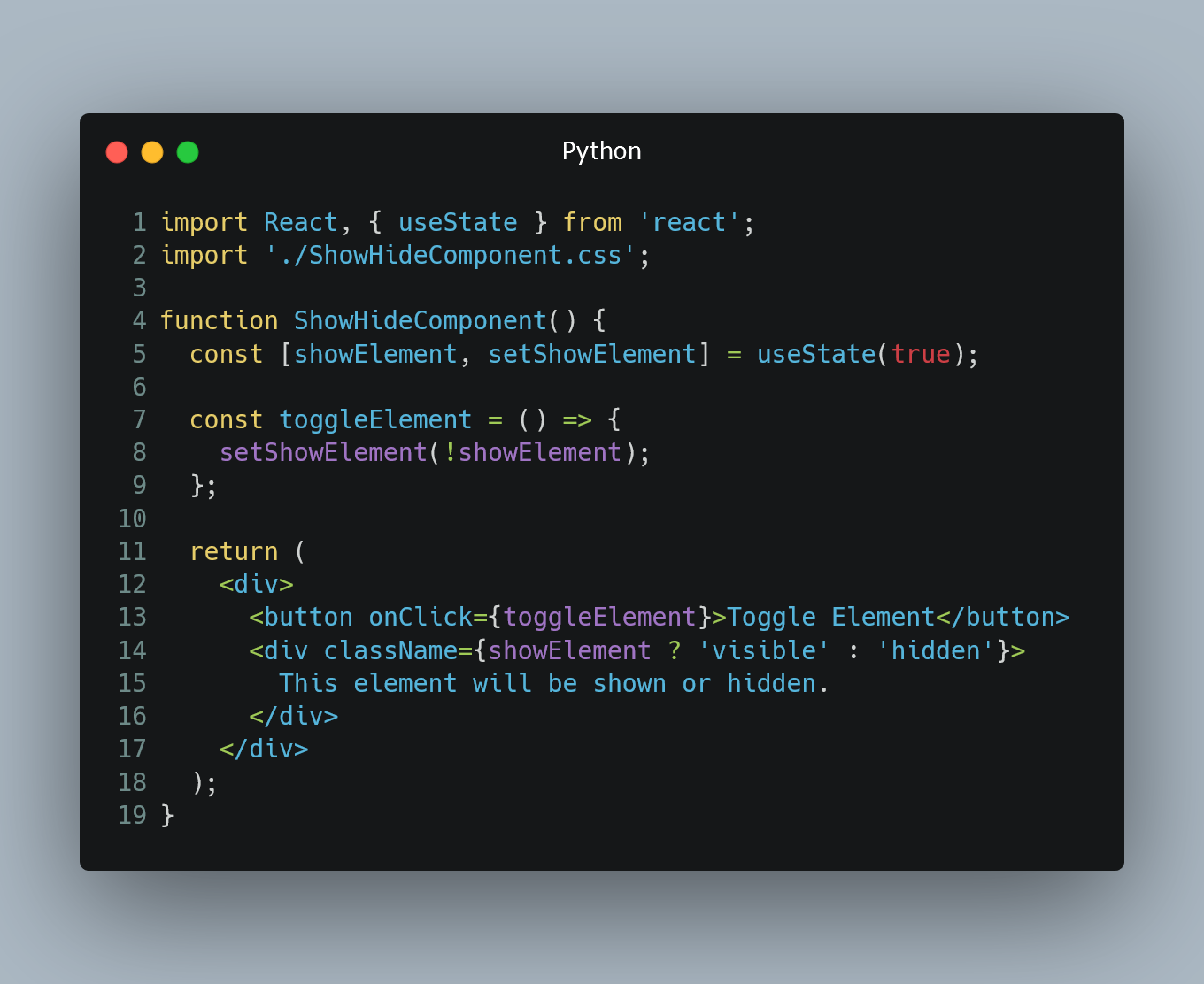
In React, you can dynamically show or hide elements based on certain conditions.
#1. Using Conditional Rendering with JavaScript if
statement
One of the simplest ways to show or hide an element in React is by using JavaScript if
statement inside the render
method.
import React, { useState } from 'react';
function ShowHideComponent() {
const [showElement, setShowElement] = useState(true);
const toggleElement = () => {
setShowElement(!showElement);
};
return (
<div>
<button onClick={toggleElement}>Toggle Element</button>
{showElement && <div>This element will be shown or hidden.</div>}
</div>
);
}
In this example, we have a component called ShowHideComponent
. It uses the useState
hook to manage the state of showElement
, which determines whether the element should be shown or hidden. When the button is clicked, the toggleElement
function updates the state, causing the element to be toggled on or off based on the value of showElement
.
#2. Using CSS with Inline Styles
You can also use CSS with inline styles to conditionally show or hide an element.
import React, { useState } from 'react';
function ShowHideComponent() {
const [showElement, setShowElement] = useState(true);
const toggleElement = () => {
setShowElement(!showElement);
};
const elementStyle = {
display: showElement ? 'block' : 'none'
};
return (
<div>
<button onClick={toggleElement}>Toggle Element</button>
<div style={elementStyle}>This element will be shown or hidden.</div>
</div>
);
}
In this example, we create an object elementStyle
with a display
property set to 'block'
or 'none'
based on the value of showElement
. The element's style
attribute is then set to elementStyle
, controlling its visibility.
#3. Using CSS Classes
If you have more complex styling requirements, you can use CSS classes to show or hide an element.
import React, { useState } from 'react';
import './ShowHideComponent.css';
function ShowHideComponent() {
const [showElement, setShowElement] = useState(true);
const toggleElement = () => {
setShowElement(!showElement);
};
return (
<div>
<button onClick={toggleElement}>Toggle Element</button>
<div className={showElement ? 'visible' : 'hidden'}>
This element will be shown or hidden.
</div>
</div>
);
}
In this example, we have two CSS classes defined in a separate file ShowHideComponent.css
: .visible
and .hidden
. We conditionally apply these classes to the element based on the value of showElement
, controlling its visibility with CSS rules.
0 Comment