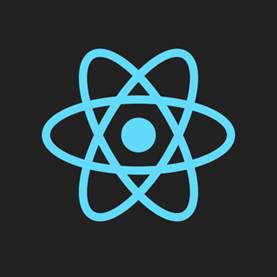
In React, it is possible for a parent component to call a method defined in a child component.
#1. Use Ref to Access Child Component
To call a child method from the parent component, you can use the ref
attribute in the parent to access the child component's instance.
import React, { useRef } from 'react';
// Child Component
class ChildComponent extends React.Component {
someMethod() {
console.log('Child method called from parent');
}
render() {
return <div>Child Component</div>;
}
}
// Parent Component
function ParentComponent() {
const childRef = useRef(null);
const handleClick = () => {
// Call the child method using the ref
childRef.current.someMethod();
};
return (
<div>
<button onClick={handleClick}>Call Child Method</button>
<ChildComponent ref={childRef} />
</div>
);
}
In this example, we create a child component called ChildComponent
with a method called someMethod()
. The parent component, ParentComponent
, uses the useRef
hook to create a reference called childRef
. When the button is clicked, the handleClick()
function calls the someMethod()
of the child component using the childRef
.
#2. Functional Child Component
If the child component is a functional component, you can use the useImperativeHandle
hook to expose specific functions to the parent component.
import React, { useRef, useImperativeHandle, forwardRef } from 'react';
// Child Component
const ChildComponent = forwardRef((props, ref) => {
useImperativeHandle(ref, () => ({
someMethod() {
console.log('Child method called from parent');
}
}));
return <div>Child Component</div>;
});
// Parent Component (same as previous example)
function ParentComponent() {
const childRef = useRef(null);
const handleClick = () => {
childRef.current.someMethod();
};
return (
<div>
<button onClick={handleClick}>Call Child Method</button>
<ChildComponent ref={childRef} />
</div>
);
}
In this case, the useImperativeHandle
hook allows the child component to expose the someMethod()
function to the parent component using the ref
provided by the parent.
0 Comment