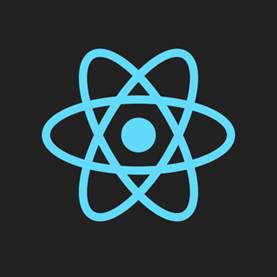
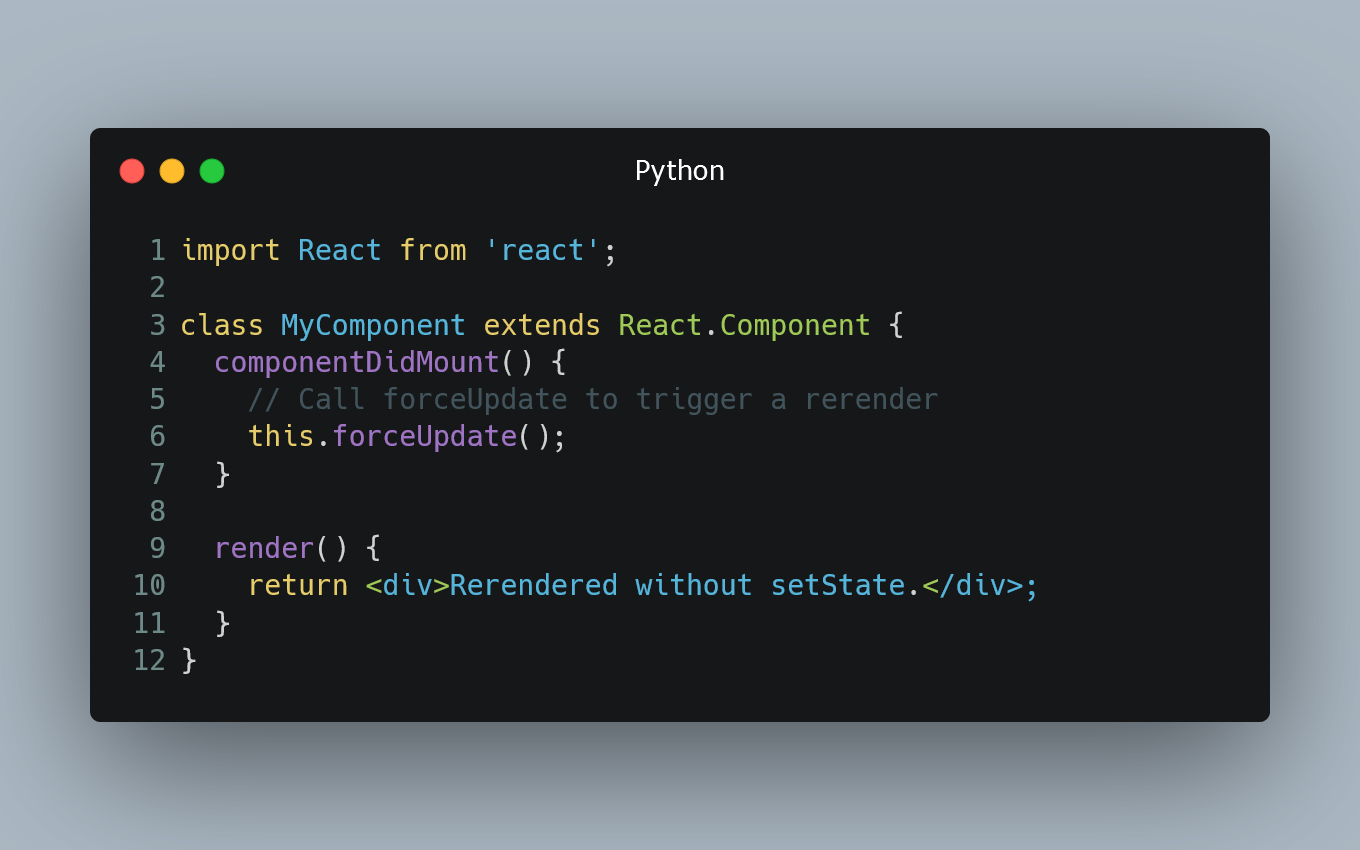
In React, components rerender automatically when their state or props change. However, there may be cases where you want to force a component to rerender without calling the setState
method.
#1. Using forceUpdate()
React provides a method called forceUpdate()
that forces a component to rerender. It should be used sparingly since manually triggering updates can lead to performance issues and may not be the best practice in most scenarios.
import React from 'react';
class MyComponent extends React.Component {
componentDidMount() {
// Call forceUpdate to trigger a rerender
this.forceUpdate();
}
render() {
return <div>Rerendered without setState.</div>;
}
}
By calling forceUpdate()
inside the componentDidMount()
method, the component will rerender once when it first mounts.
#2. Using key
Prop
Another way to force a component to rerender is by using the key
prop. When the key
prop changes, React treats it as a new component instance and forces a rerender.
import React, { useState } from 'react';
function MyComponent() {
const [rerenderKey, setRerenderKey] = useState(1);
const handleRerender = () => {
// Increment the key to force a rerender
setRerenderKey((prevKey) => prevKey + 1);
};
return (
<div>
<button onClick={handleRerender}>Rerender</button>
<div key={rerenderKey}>Component will rerender.</div>
</div>
);
}
In this example, a state variable rerenderKey
is used as the key
prop. When the button is clicked, the rerenderKey
state is incremented, causing the component to rerender.
#3. Use with Caution
Both methods allow you to force a component to rerender without using setState
, but they should be used with caution. Manually triggering rerenders can lead to difficult-to-maintain code and impact performance.
0 Comment