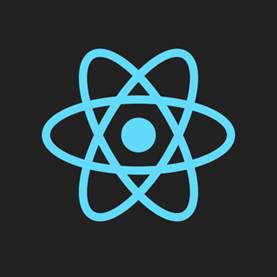
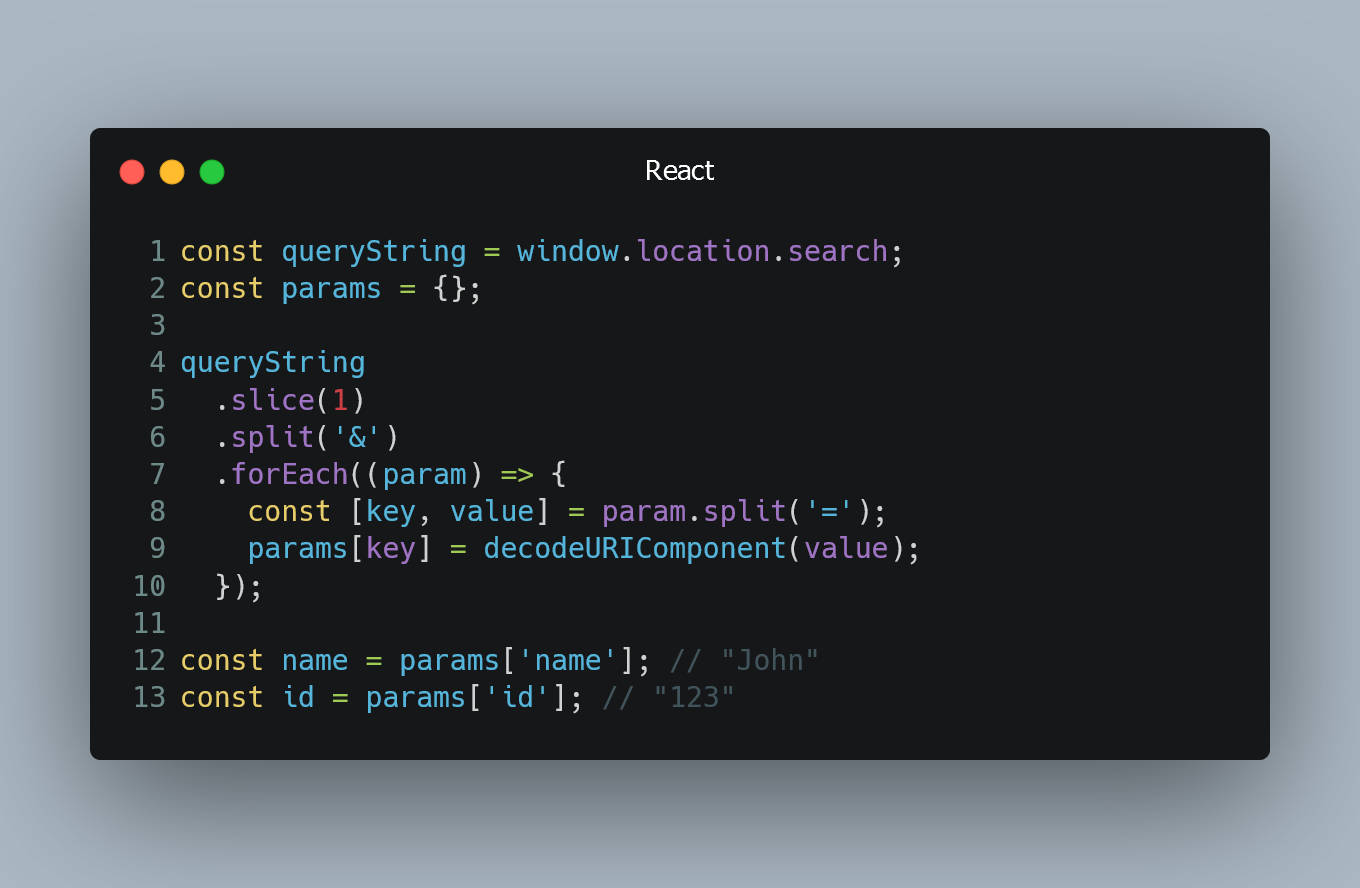
In web development, query strings are used to pass data in the URL. These query strings can contain parameters and their values.
#1. Using URLSearchParams API
Modern browsers provide the URLSearchParams
API, which makes it easy to work with query strings.
// Example URL: http://example.com/?name=John&id=123
const queryString = window.location.search;
const urlParams = new URLSearchParams(queryString);
// Get the value of a parameter
const name = urlParams.get('name'); // "John"
const id = urlParams.get('id'); // "123"
In this example, we first extract the query string from the URL using window.location.search
. Then, we create a new URLSearchParams
object with the query string. With urlParams.get('paramName')
, we can get the value of a specific parameter from the query string.
#2. Parsing Manually
If you need to support older browsers that do not have the URLSearchParams
API, you can still parse the query string manually using JavaScript.
// Example URL: http://example.com/?name=John&id=123
const queryString = window.location.search;
const params = {};
queryString
.slice(1)
.split('&')
.forEach((param) => {
const [key, value] = param.split('=');
params[key] = decodeURIComponent(value);
});
const name = params['name']; // "John"
const id = params['id']; // "123"
In this example, we manually parse the query string by splitting it at the '&' character and then further splitting each parameter at the '=' character. We decode the parameter values using decodeURIComponent
to handle special characters.
#3. Handling Missing Parameters
When extracting parameter values, it's essential to handle cases where a parameter may not be present in the query string.
const queryString = window.location.search;
const urlParams = new URLSearchParams(queryString);
// Get the value of a parameter with a default value
const name = urlParams.get('name') || 'Guest';
In this example, if the 'name' parameter is not present in the query string, the variable name
will be assigned the default value 'Guest'.
0 Comment