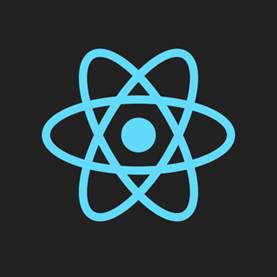
In React, when rendering arrays of elements as children, it is essential to provide a unique key
prop for each child.
#1. Why Keys are Necessary
Keys serve as hints to React about the identity of each element in the array. When React renders an array, it uses the keys to determine which elements have changed, been added, or removed. Without keys, React may have to re-render the entire list of elements, which can lead to performance issues and potential UI glitches.
#2. Choosing a Suitable Key
For a key to be effective, it should be a unique identifier for each item in the array. Commonly, you can use unique IDs provided by your data or, in the absence of such IDs, use array indices as keys. However, using array indices should be a last resort, as reordering or adding/removing elements can lead to incorrect rendering.
function MyComponent() {
const data = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Charlie' },
];
return (
<ul>
{data.map((item) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
}
In this example, the id
property from each data item is used as the unique key
for the list of elements.
#3. The Importance of Stability
It's crucial to keep the keys stable across renders. Changing keys for existing elements in the array can lead to unintended remounting of components and adversely affect performance.
When using database IDs as keys, they typically remain stable as long as the data order doesn't change. However, if you use random or generated keys, ensure they don't change between renders.
#4. Key and Component Reconciliation
React uses keys to perform a process called "reconciliation." When the array changes, React will compare the new keys with the previous ones and determine which elements are new, removed, or modified. By using stable and unique keys, React can efficiently update the DOM, leading to a smoother user experience.
0 Comment