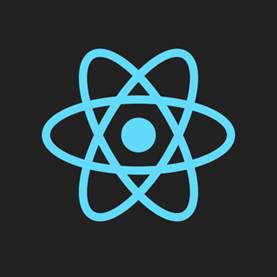
In React, you may encounter situations where you need to detect when a user clicks outside a specific component, such as a dropdown, modal, or popover, to close it or perform other actions. This tutorial will show you how to achieve this functionality.
#1. Using the useRef
Hook
To detect clicks outside a React component, you can use the useRef
hook to create a reference to a DOM element. Then, you can listen for click events on the document and check if the clicked element is outside the component.
import React, { useEffect, useRef } from 'react';
function OutsideClickComponent() {
const wrapperRef = useRef(null);
useEffect(() => {
/**
* Function to handle clicks outside the component.
* If the clicked element is not a child of the component, perform actions.
*/
const handleClickOutside = (event) => {
if (wrapperRef.current && !wrapperRef.current.contains(event.target)) {
// Perform actions here (e.g., close the component)
console.log('Clicked outside the component');
}
};
// Bind the event listener when the component mounts
document.addEventListener('click', handleClickOutside);
// Unbind the event listener when the component unmounts
return () => {
document.removeEventListener('click', handleClickOutside);
};
}, []);
return (
<div ref={wrapperRef}>
{/* Your component content here */}
<p>Click outside this component to detect the click.</p>
</div>
);
}
In this example, we create a component called OutsideClickComponent
. We use the useRef
hook to create a reference called wrapperRef
, which points to the component's outermost DOM element. Then, we use the useEffect
hook with an empty dependency array ([]
) to add an event listener to the document when the component mounts. The event listener checks if the clicked element is not a child of the component, and if so, it performs the desired actions.
#2. Custom Hook
You can also create a custom hook to encapsulate the logic for detecting clicks outside the component and make it reusable across multiple components.
import React, { useEffect, useRef } from 'react';
function useOutsideClick(callback) {
const ref = useRef(null);
useEffect(() => {
const handleClickOutside = (event) => {
if (ref.current && !ref.current.contains(event.target)) {
callback();
}
};
document.addEventListener('click', handleClickOutside);
return () => {
document.removeEventListener('click', handleClickOutside);
};
}, [callback]);
return ref;
}
function OutsideClickComponent() {
const handleOutsideClick = () => {
// Perform actions here (e.g., close the component)
console.log('Clicked outside the component');
};
const wrapperRef = useOutsideClick(handleOutsideClick);
return (
<div ref={wrapperRef}>
{/* Your component content here */}
<p>Click outside this component to detect the click.</p>
</div>
);
}
In this example, we create a custom hook called useOutsideClick
, which takes a callback
function as a parameter. Inside the component OutsideClickComponent
, we use the custom hook to handle the click outside logic.
By using either the useRef
hook directly or a custom hook, you can easily detect clicks outside a React component and trigger the desired actions accordingly. This technique is helpful for creating user-friendly interfaces with components that need to respond to clicks outside their boundaries.
0 Comment