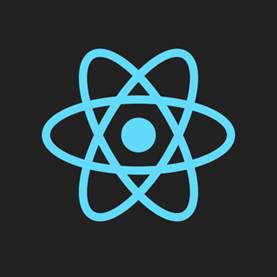
Published in
React
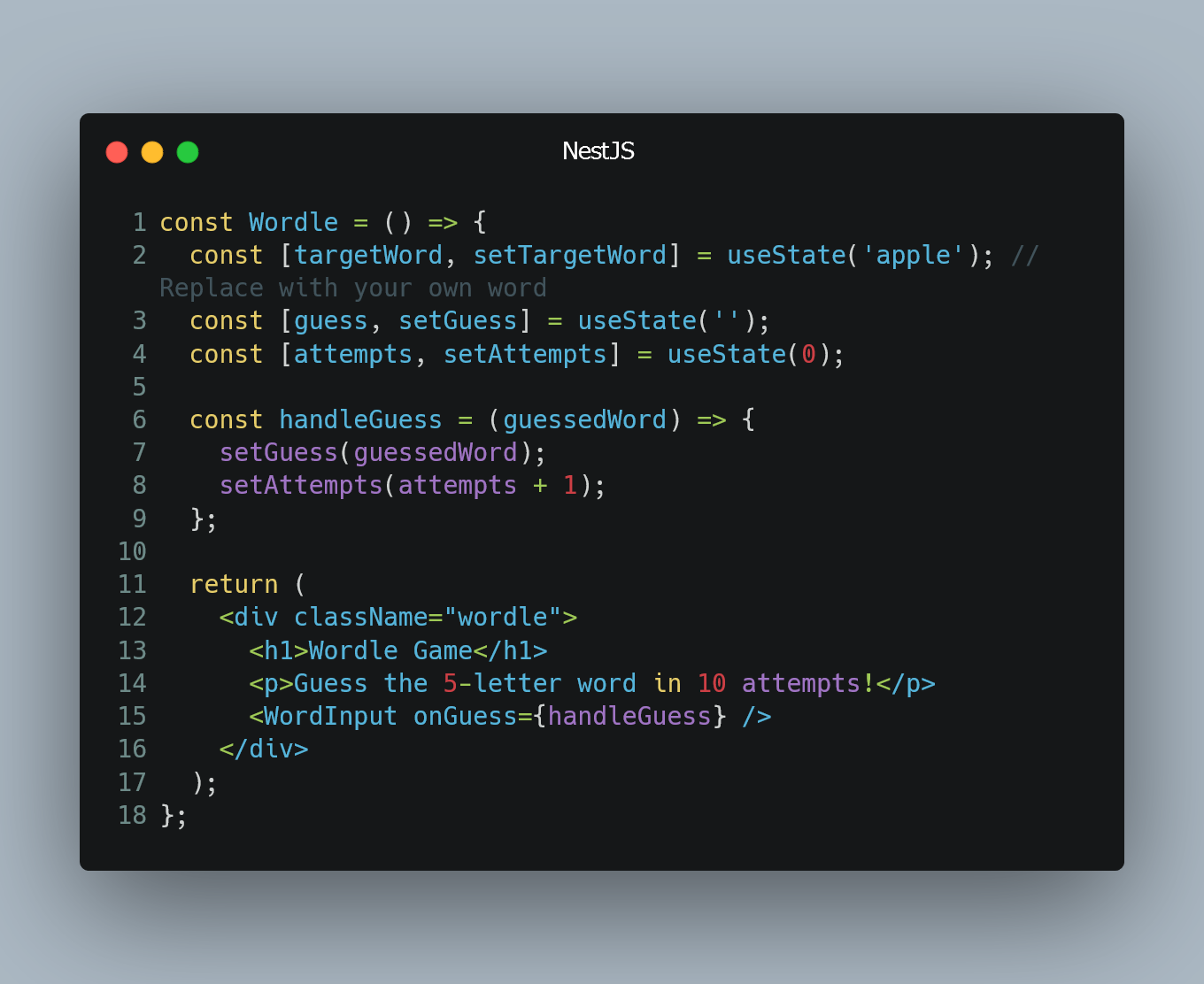
Wordle is a word puzzle game where players guess a hidden word within a limited number of tries.
Prerequisites:
- Basic knowledge of JavaScript and ReactJS
- Node.js and npm installed on your system
Step 1: Setup
- Create a new React app using Create React App. Open your terminal and run:
npx create-react-app wordle-game cd wordle-game
Step 2: Component Structure
- In the
src
directory, create a new component folder namedWordle
. - Inside the
Wordle
folder, create three files:Wordle.js
,WordInput.js
, andApp.css
.
Step 3: Implement the Components
- Open
Wordle.js
and define the basic structure of the game board. This component will manage the game state and render the game elements.
import React, { useState } from 'react';
import './App.css';
import WordInput from './WordInput';
const Wordle = () => {
const [targetWord, setTargetWord] = useState('apple'); // Replace with your own word
const [guess, setGuess] = useState('');
const [attempts, setAttempts] = useState(0);
const handleGuess = (guessedWord) => {
setGuess(guessedWord);
setAttempts(attempts + 1);
};
return (
<div className="wordle">
<h1>Wordle Game</h1>
<p>Guess the 5-letter word in 10 attempts!</p>
<WordInput onGuess={handleGuess} />
<p>Your guess: {guess}</p>
<p>Attempts: {attempts}</p>
</div>
);
};
export default Wordle;
- Open
WordInput.js
and create an input field where the player can guess words.
import React, { useState } from 'react';
const WordInput = ({ onGuess }) => {
const [inputValue, setInputValue] = useState('');
const handleChange = (e) => {
setInputValue(e.target.value);
};
const handleSubmit = (e) => {
e.preventDefault();
onGuess(inputValue);
setInputValue('');
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
value={inputValue}
onChange={handleChange}
maxLength={5}
/>
<button type="submit">Guess</button>
</form>
);
};
export default WordInput;
- Open
App.css
and add some basic styling to make the game look better.
.wordle {
text-align: center;
margin-top: 50px;
}
input {
padding: 5px;
margin-right: 10px;
text-transform: uppercase;
}
Demo:
Step 4: Run the Application
- Save your changes and return to the root directory of your app.
- In the terminal, run
npm start
to start the development server. - Open your web browser and navigate to
http://localhost:3000
to see your Wordle game in action!
0 Comment