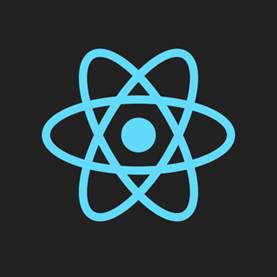
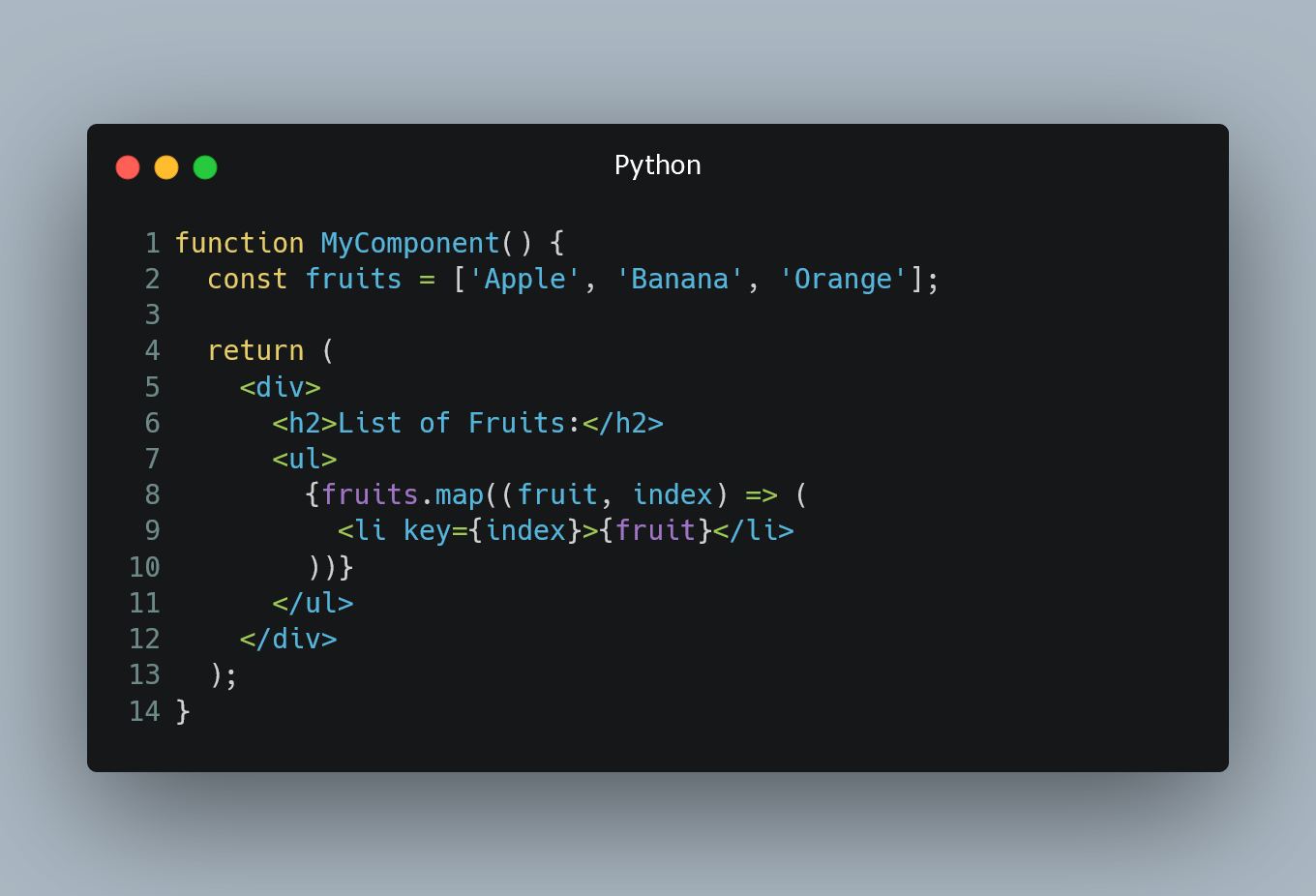
In React, you can use loops to dynamically render repetitive elements or content within JSX (JavaScript XML).
#1. Using map()
to Loop
One of the most common ways to loop inside JSX is by using the map()
method on an array. The map()
method creates a new array by calling a provided function on each element of the original array.
function MyComponent() {
const fruits = ['Apple', 'Banana', 'Orange'];
return (
<div>
<h2>List of Fruits:</h2>
<ul>
{fruits.map((fruit, index) => (
<li key={index}>{fruit}</li>
))}
</ul>
</div>
);
}
In this example, we have an array of fruits, and we use the map()
method to create a list of <li>
elements for each fruit in the array. The key
attribute is important to provide a unique identifier for each list item, improving rendering performance and helping React efficiently update the UI.
#2. Looping with Conditional Rendering
You can also use loops in combination with conditional rendering to conditionally display elements based on certain conditions.
function MyComponent() {
const numbers = [1, 2, 3, 4, 5];
return (
<div>
<h2>Even Numbers:</h2>
<ul>
{numbers.map((number) => (
{number % 2 === 0 && <li key={number}>{number}</li>}
))}
</ul>
</div>
);
}
In this example, we have an array of numbers, and we use the map()
method along with conditional rendering ({number % 2 === 0 && <li key={number}>{number}</li>}
) to only display even numbers.
#3. Using Other Loops
While the map()
method is the most common choice for looping inside JSX, you can also use other loop constructs such as for
loops or while
loops. However, remember to avoid using loops with side effects (e.g., modifying state or making network requests) directly inside the JSX, as it can lead to unexpected behavior and performance issues.
function MyComponent() {
const numbers = [1, 2, 3, 4, 5];
const items = [];
for (const number of numbers) {
items.push(<li key={number}>{number}</li>);
}
return (
<div>
<h2>List of Numbers:</h2>
<ul>
{items}
</ul>
</div>
);
}
In this example, we use a for
loop to create a list of <li>
elements for each number in the array.
0 Comment