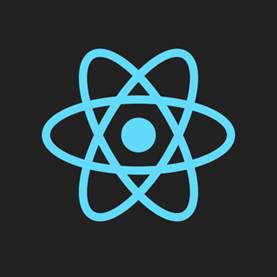
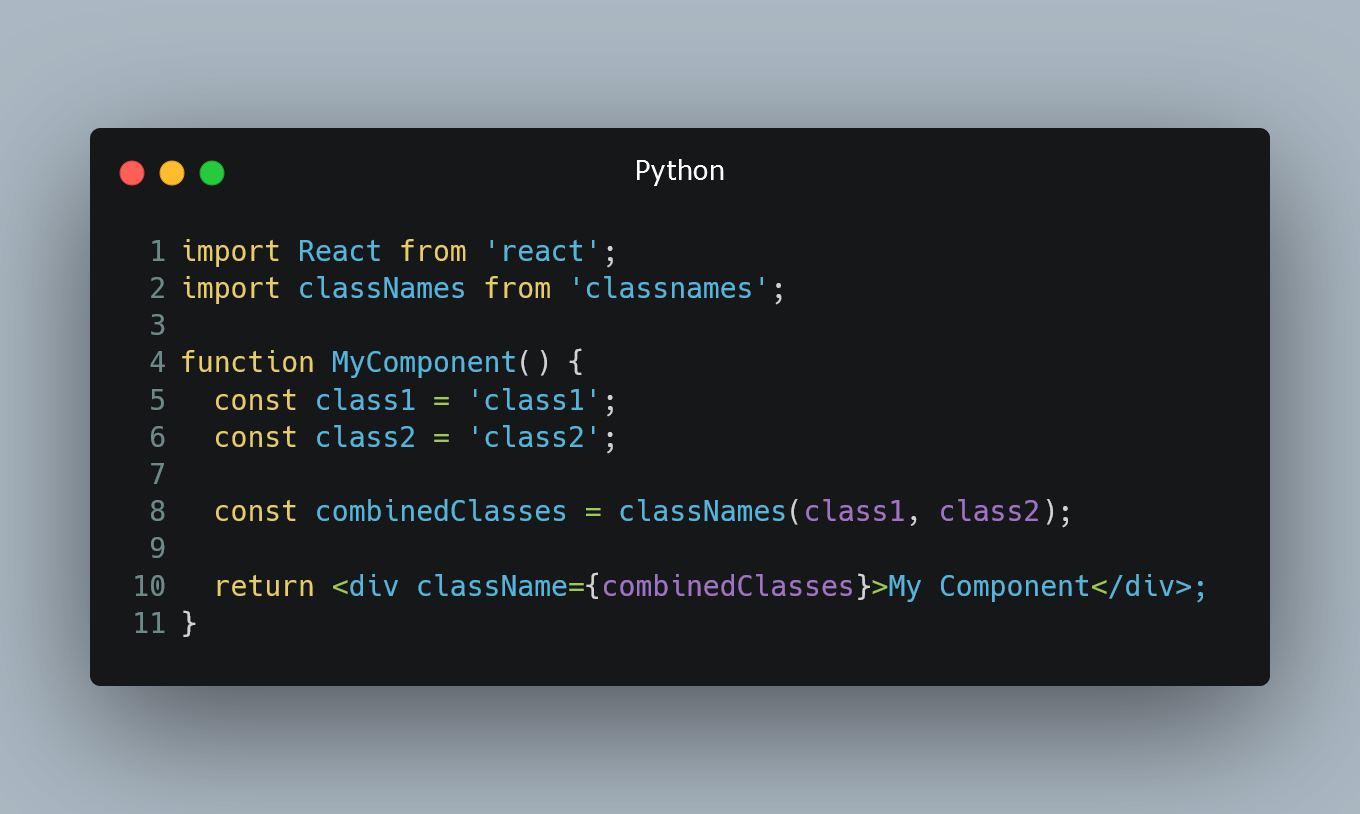
In React, you can apply multiple CSS classes to a component to style it according to your design requirements.
#1. Using String Concatenation
One of the simplest ways to add multiple classes to a React component is by using string concatenation within the className
prop.
import React from 'react';
function MyComponent() {
const class1 = 'class1';
const class2 = 'class2';
return <div className={class1 + ' ' + class2}>My Component</div>;
}
In this example, we define two CSS classes class1
and class2
. The className
prop of the div
element concatenates both classes together with a space to apply them to the component.
#2. Using Template Literals
ES6 template literals offer a cleaner way to add multiple classes to a React component.
import React from 'react';
function MyComponent() {
const class1 = 'class1';
const class2 = 'class2';
return <div className={`${class1} ${class2}`}>My Component</div>;
}
In this example, we use template literals to interpolate the variables class1
and class2
within backticks, separated by a space.
#3. Using Array Join Method
If you have an array of classes, you can use the join
method to concatenate them and apply them to the component.
import React from 'react';
function MyComponent() {
const classes = ['class1', 'class2'];
return <div className={classes.join(' ')}>My Component</div>;
}
In this example, we define an array classes
containing the class names. The join(' ')
method joins the elements of the array with a space, resulting in a single string of classes applied to the component.
#4. Using Classnames Library
For more advanced scenarios, you can use the classnames
library, which simplifies working with multiple classes in React components.
First, install the library:
npm install classnames
Then, use it in your component:
import React from 'react';
import classNames from 'classnames';
function MyComponent() {
const class1 = 'class1';
const class2 = 'class2';
const combinedClasses = classNames(class1, class2);
return <div className={combinedClasses}>My Component</div>;
}
In this example, we use the classNames
function from the classnames
library to combine the classes.
1 Comment
But I think this `className={`${class1} ${class2}`}` more simple and not need to add more library and increase my bundle