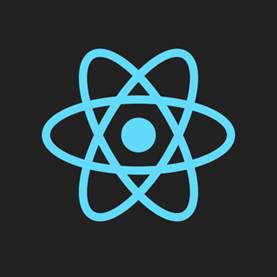
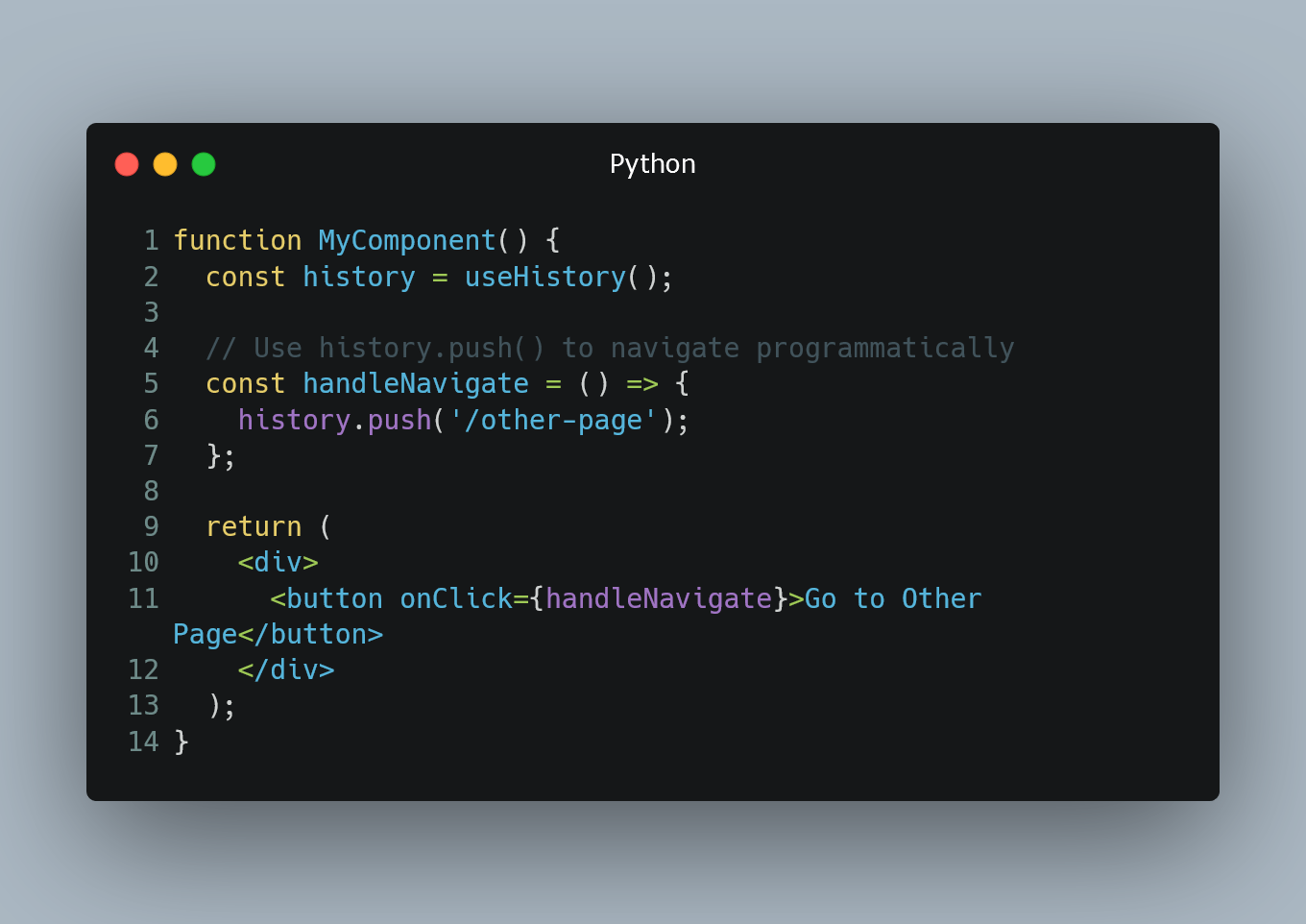
React Router is a popular library for handling routing in React applications. It allows you to navigate between different components or pages based on the URL.
#1. Install React Router
Before you can use React Router, you need to install it in your project. You can do this using npm or yarn.
npm install react-router-dom
or
yarn add react-router-dom
#2. Import Required Components
To programmatically navigate using React Router, you need to import some components from the react-router-dom
package.
import { useHistory } from 'react-router-dom';
#3. Access the History Object
The useHistory
hook allows you to access the history
object, which contains methods to navigate programmatically.
function MyComponent() {
const history = useHistory();
// Use history.push() to navigate programmatically
const handleNavigate = () => {
history.push('/other-page');
};
return (
<div>
<button onClick={handleNavigate}>Go to Other Page</button>
</div>
);
}
#4. Navigate to Specific Routes
You can use the history.push()
method to navigate to a specific route programmatically. Provide the desired route path as an argument to the function.
function AnotherComponent() {
const history = useHistory();
const handleNavigate = () => {
history.push('/another-page');
};
return (
<div>
<button onClick={handleNavigate}>Go to Another Page</button>
</div>
);
}
0 Comment