#Python
Making a Flat List Out of a List of Lists
To flatten a list of lists into a single, one-dimensional list in Python, you can use list comprehension or the itertools.chain() function.
#1. Using...
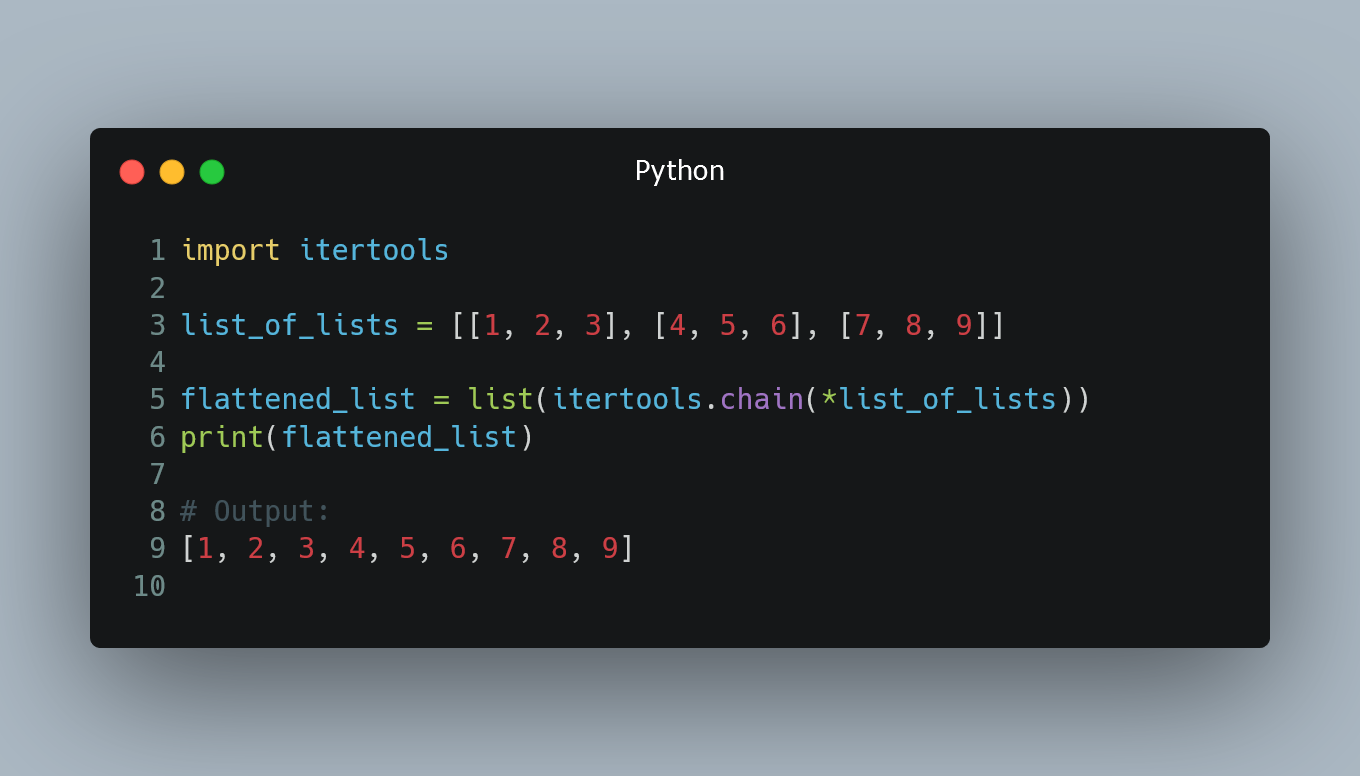
Adding New Keys to a Dictionary
In Python, you can add new keys to a dictionary by simply assigning a value to the desired key.
#1. Adding a Single Key-Value Pair
To add a single key...
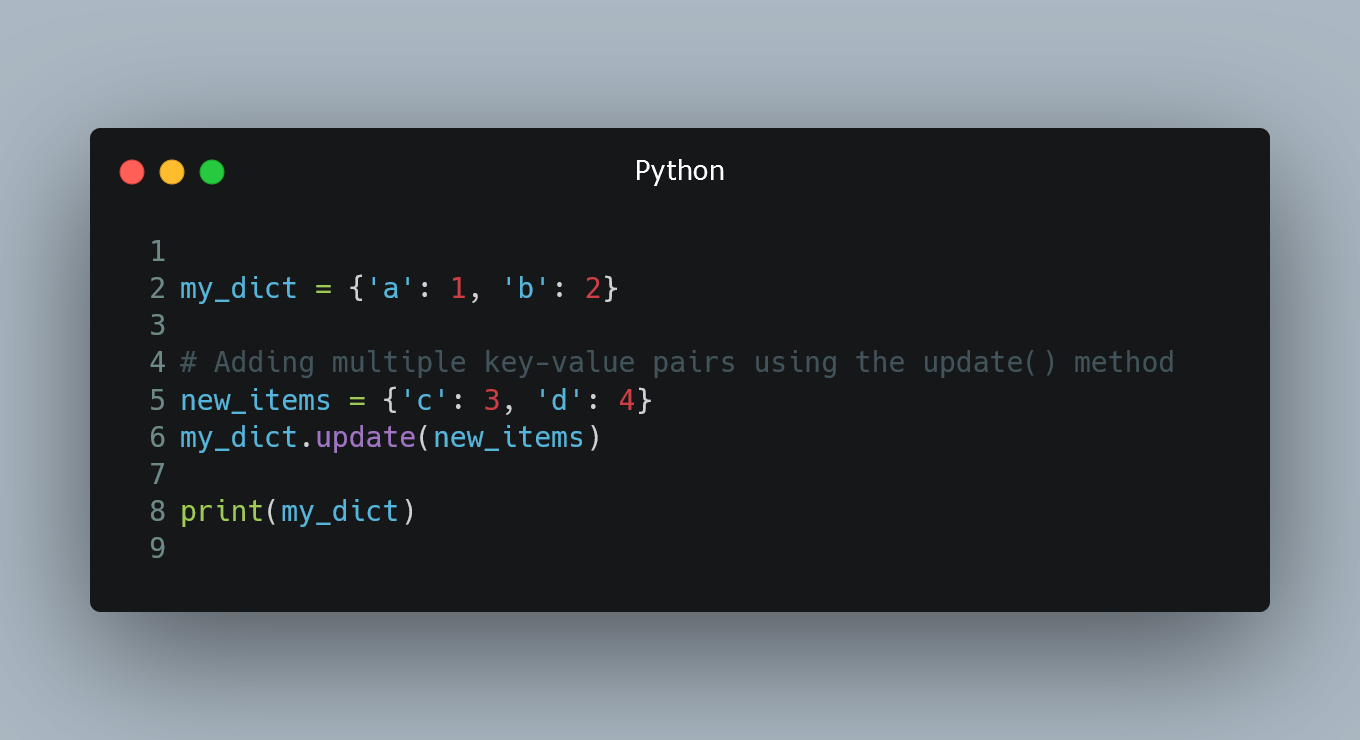
Iterating over Rows in a DataFrame in Pandas
In Pandas, you can iterate over rows in a DataFrame using various methods. Each row in a DataFrame is represented as a Series, and there are different...
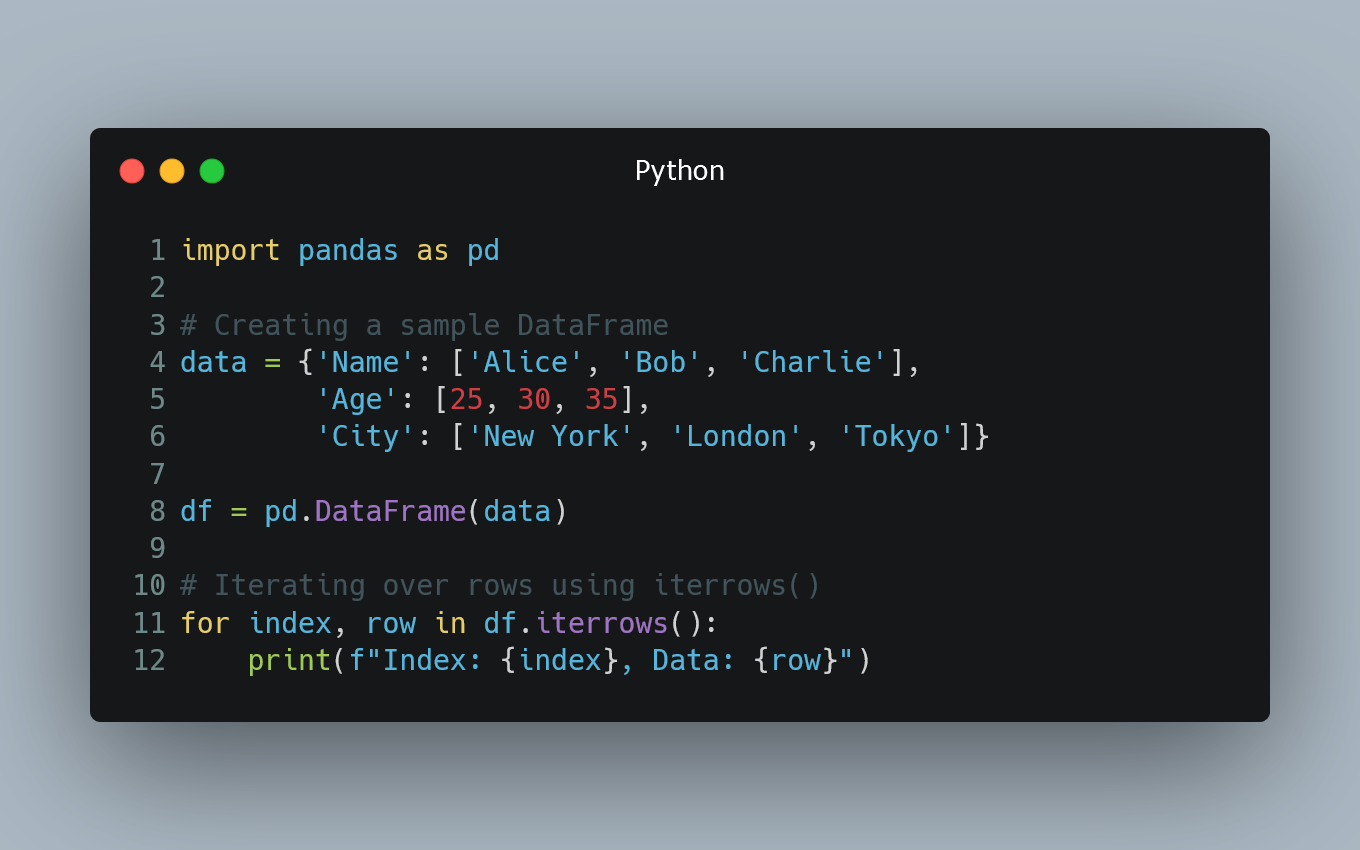
Ternary Conditional Operator
Python does have a ternary conditional operator, which allows you to write a concise one-liner for simple conditional expressions.
result_if_true if c...
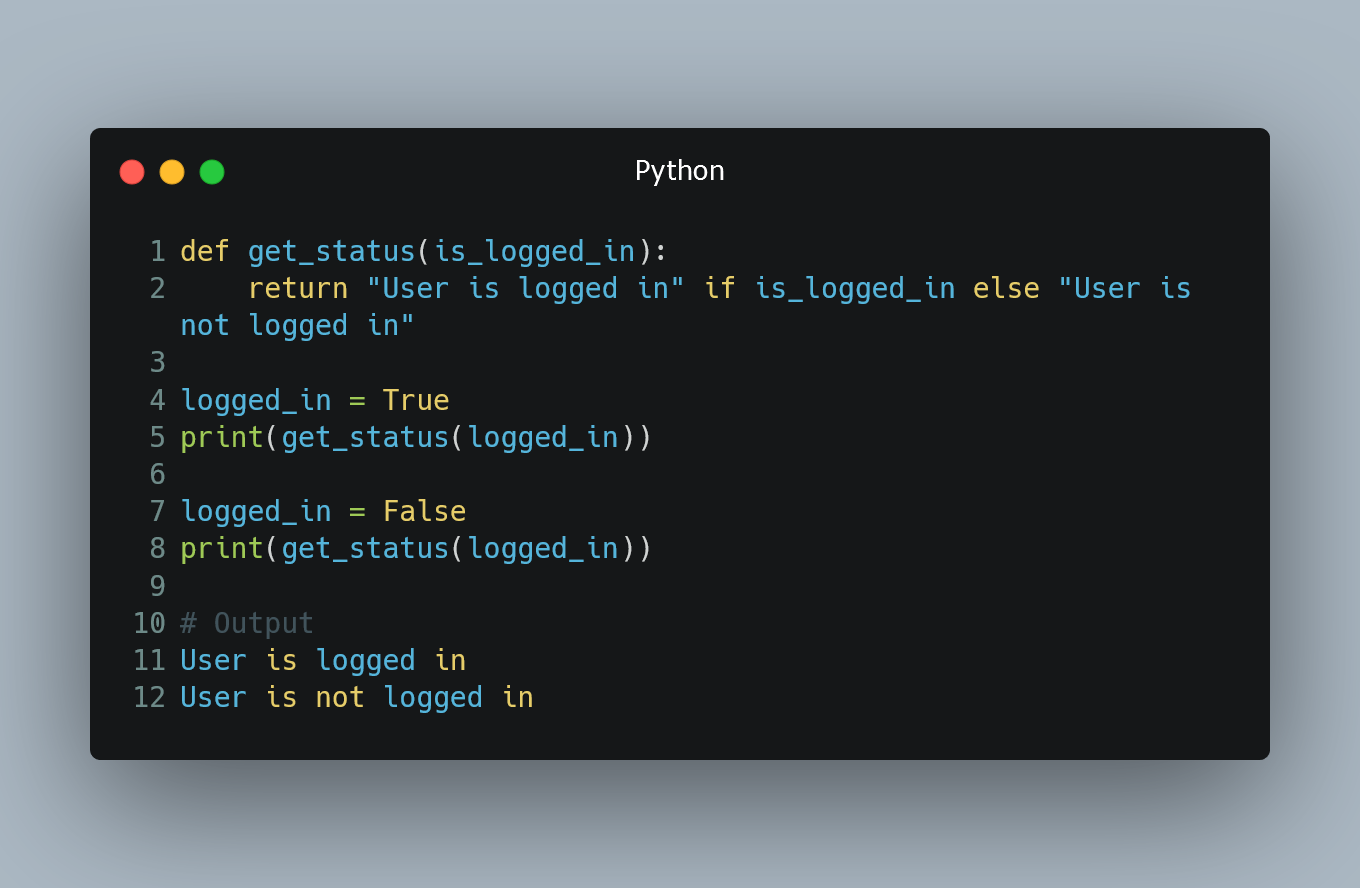
Finding the Index of an Item in a List
To find the index of an item in a list in Python, you can use the index() method or a combination of built-in functions such as enumerate() and list c...
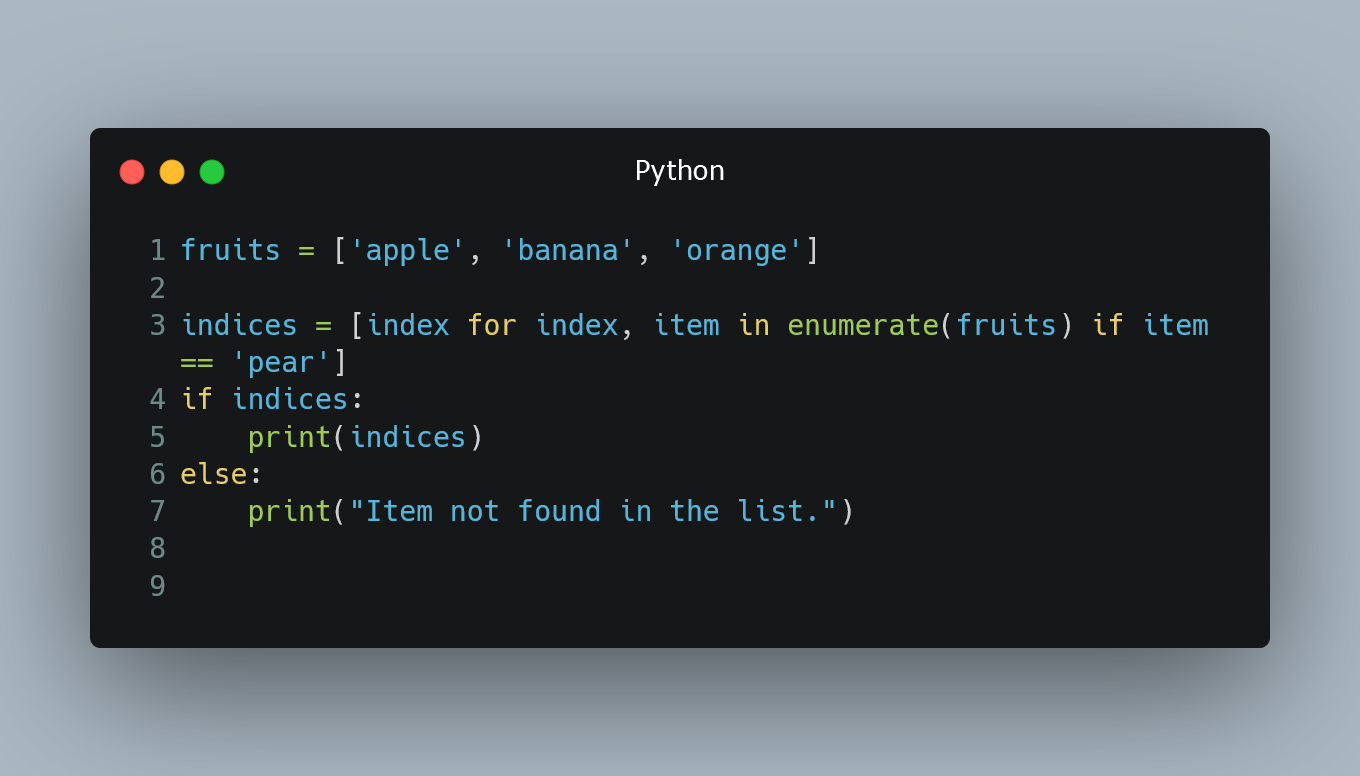
Getting the Current Time
In Python, you can get the current time using the datetime module. The datetime module provides various functions and classes to work with dates and t...
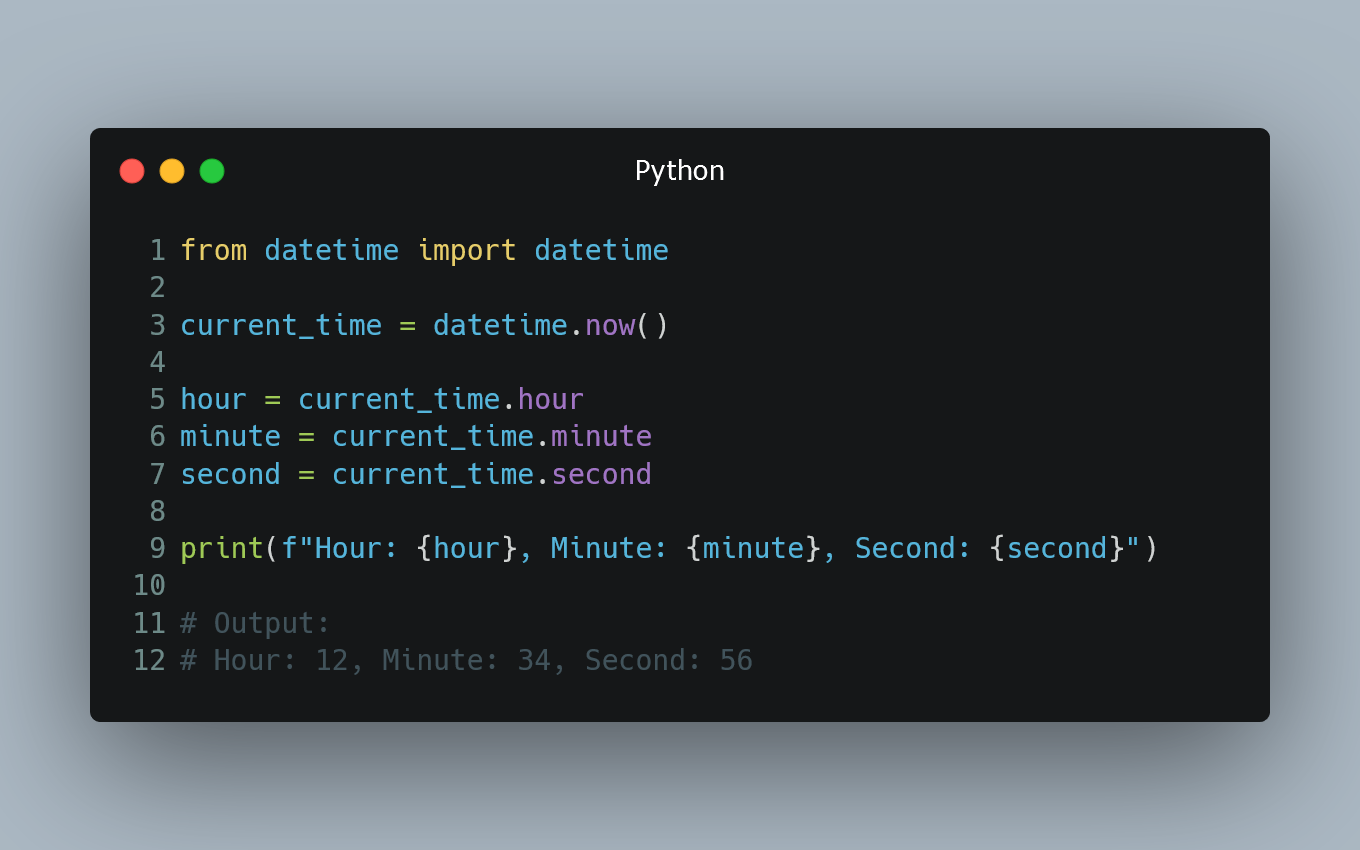
Checking Whether a File Exists Without Exceptions
To check whether a file exists in Python without raising exceptions, you can use various methods provided by the os and os.path modules.
#1. Using os....
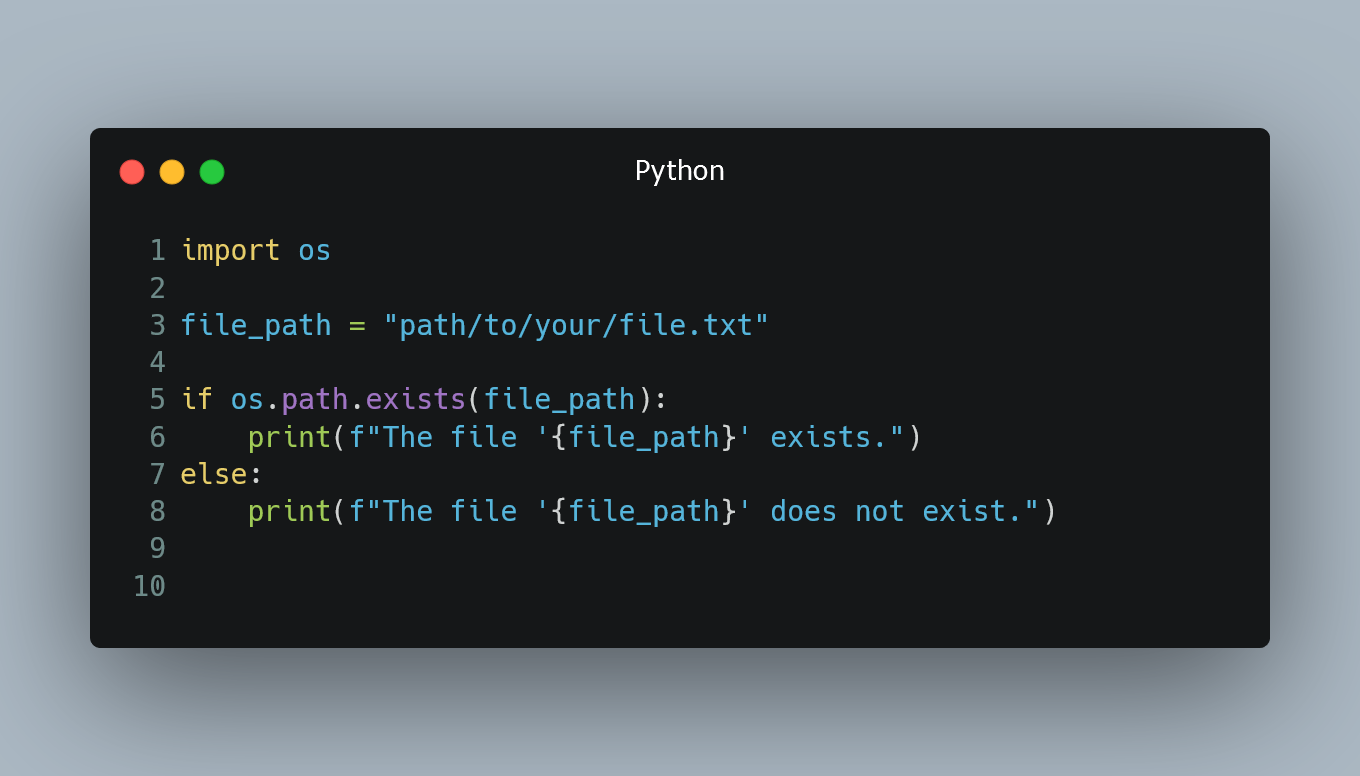
Accessing the Index in for Loops
In Python, you can access the index of the current item in a 'for' loop using the built-in enumerate() function.
#1. Using enumerate() Function
The en...
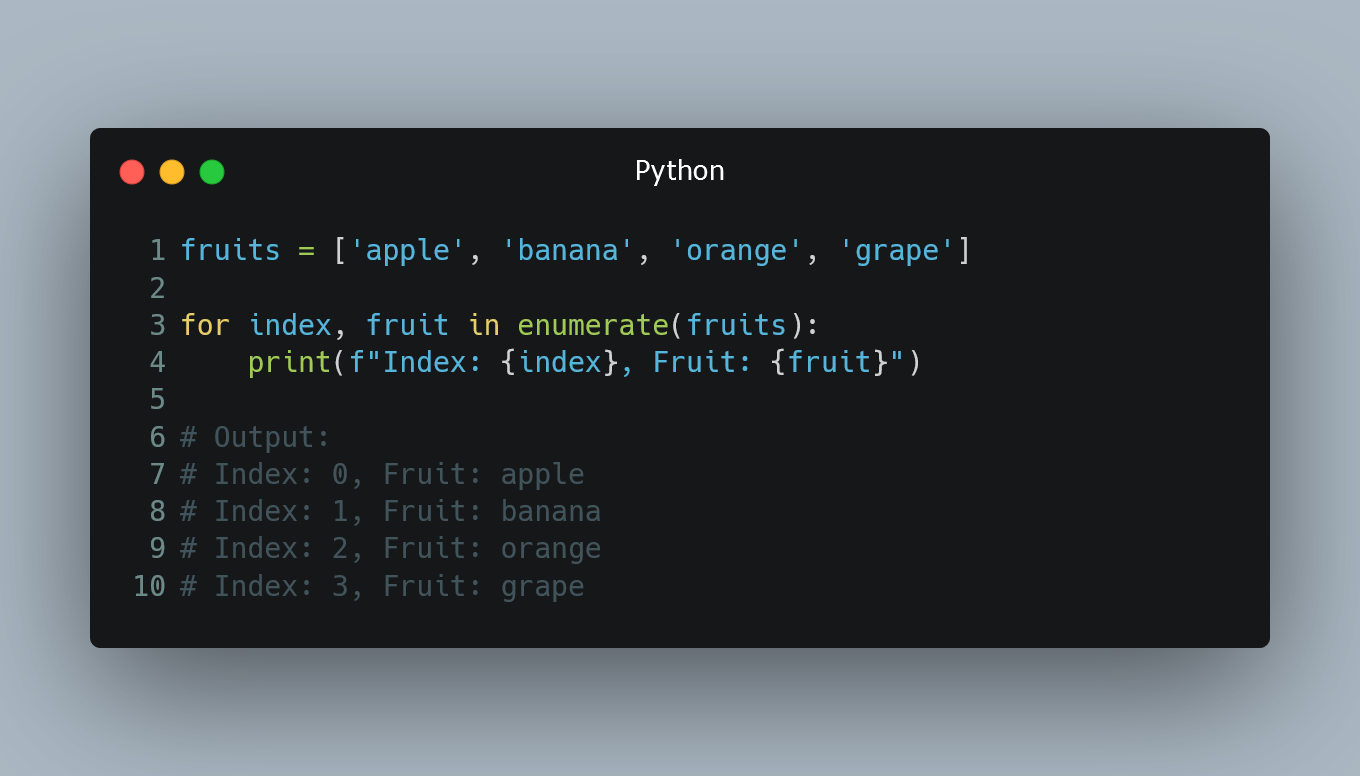
Merging Two Dictionaries in a Single Expression
In Python, you can easily merge two dictionaries into a single dictionary using a concise one-liner expression.
#1. Using the update() Method
The upda...
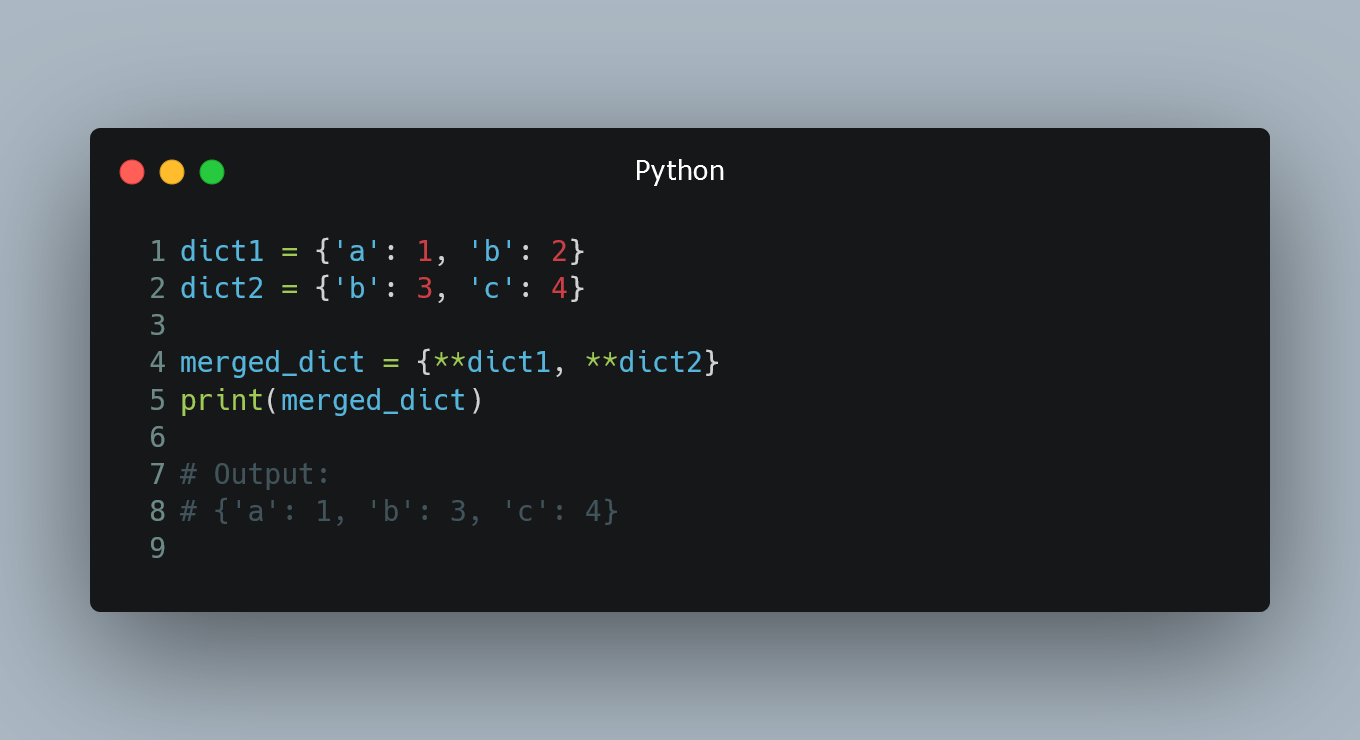
Iterating over dictionaries using for loops
In Python, you can iterate over the key-value pairs in a dictionary using 'for' loops.
#1. Iterating over Keys
To iterate over the keys of a dictionar...
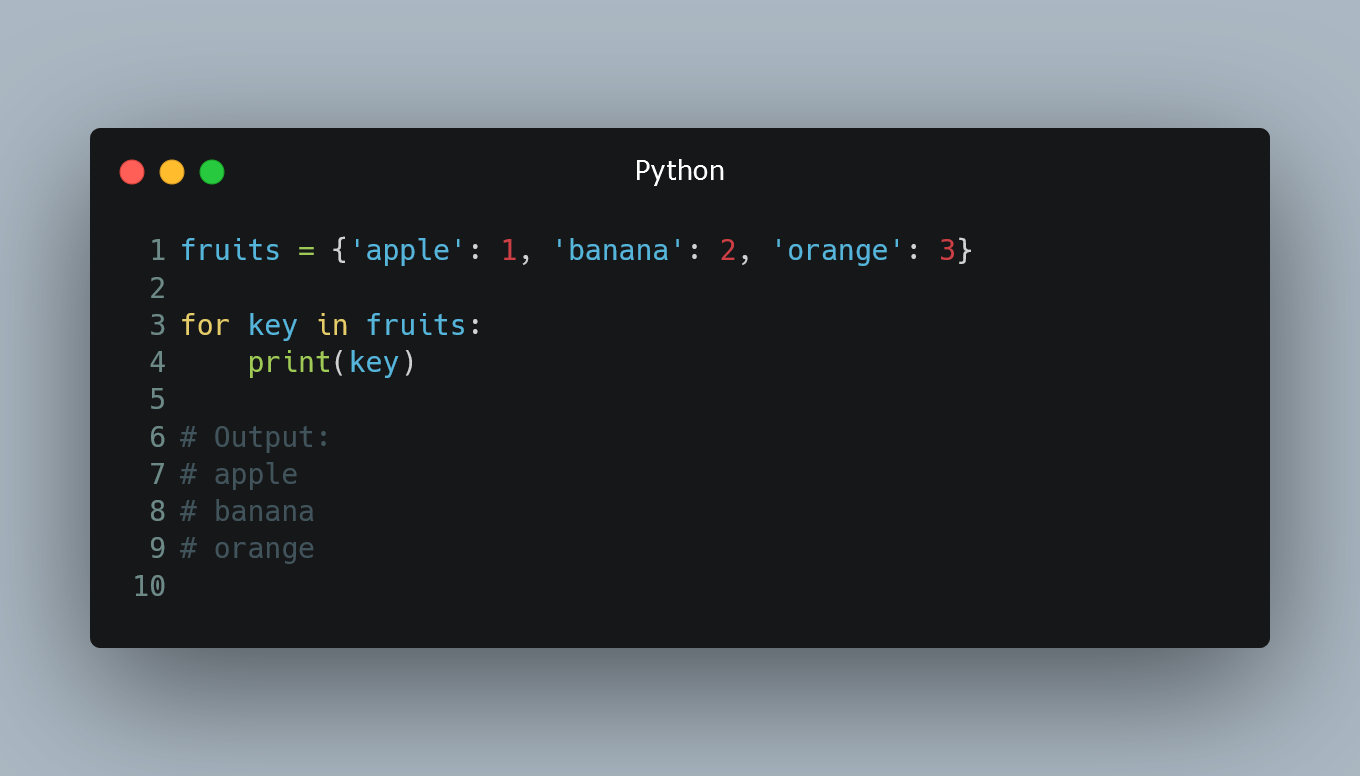