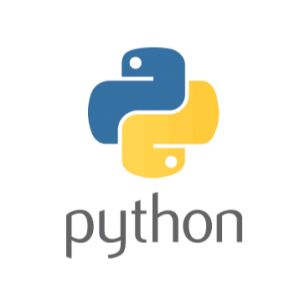
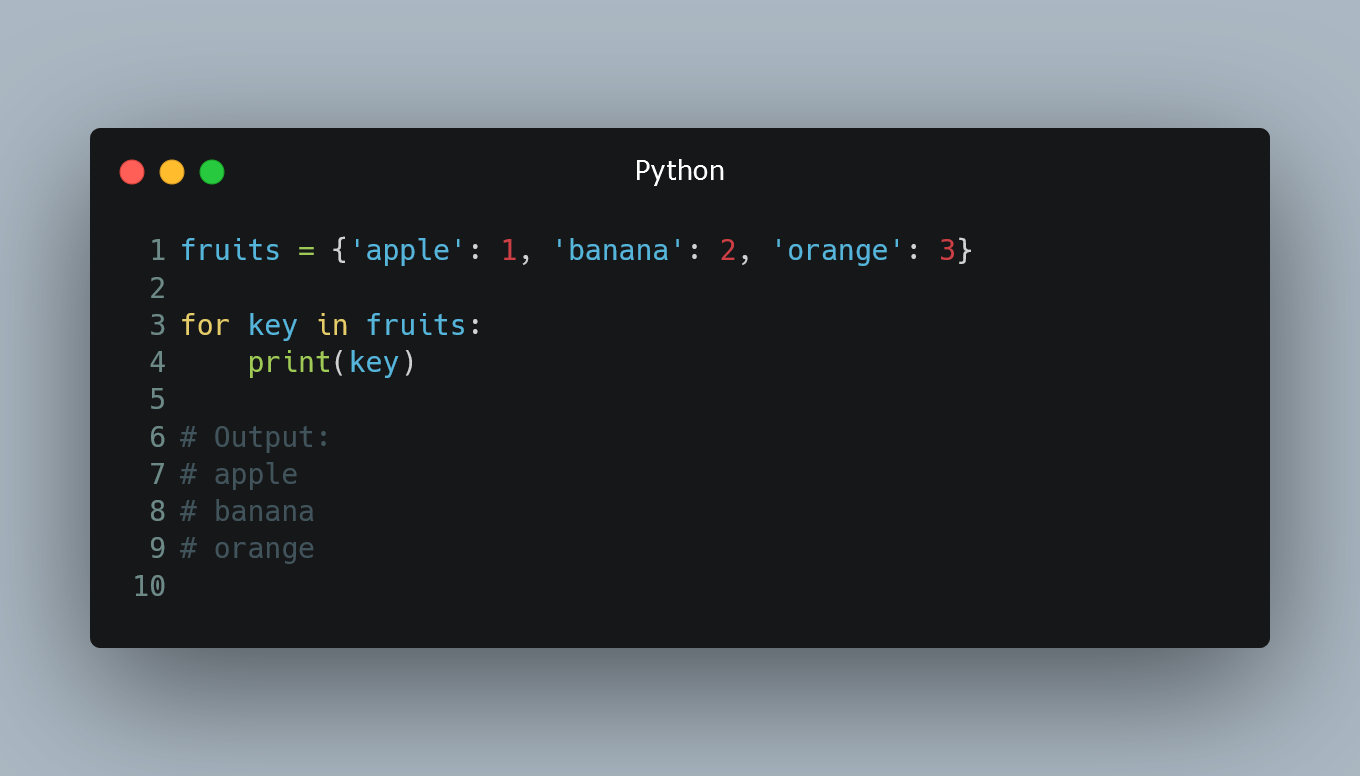
In Python, you can iterate over the key-value pairs in a dictionary using 'for' loops.
#1. Iterating over Keys
To iterate over the keys of a dictionary, you can use the 'for' loop with the dictionary itself:
fruits = {'apple': 1, 'banana': 2, 'orange': 3}
for key in fruits:
print(key)
Output:
apple
banana
orange
In this example, the 'for' loop iterates over the keys of the 'fruits' dictionary, and in each iteration, the variable 'key' contains the current key.
#2. Iterating over Values
If you need to iterate over the values of the dictionary, you can use the 'values()' method:
fruits = {'apple': 1, 'banana': 2, 'orange': 3}
for value in fruits.values():
print(value)
Output:
1
2
3
The 'values()' method returns a view object that represents the values of the dictionary. The 'for' loop iterates over this view object, and in each iteration, the variable 'value' contains the current value.
#3. Iterating over Key-Value Pairs
To iterate over both the keys and values simultaneously, you can use the 'items()' method:
fruits = {'apple': 1, 'banana': 2, 'orange': 3}
for key, value in fruits.items():
print(key, value)
Output:
apple 1
banana 2
orange 3
The 'items()' method returns a view object that represents the key-value pairs in the dictionary. The 'for' loop iterates over this view object, and in each iteration, the variables 'key' and 'value' contain the current key and value, respectively.
#4. Ordering in Python 3.7 and Later
Since Python 3.7, dictionary insertion order is preserved. This means that when iterating over a dictionary, the items will be in the order they were inserted.
#5. Caution with Large Dictionaries
When iterating over large dictionaries, keep in mind that dictionaries are unordered collections. So, the order of items might not be what you expect, especially in Python versions prior to 3.7.
0 Comment