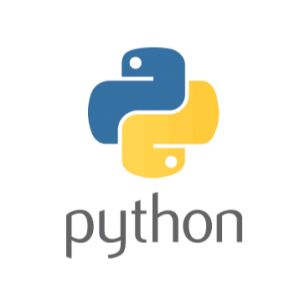
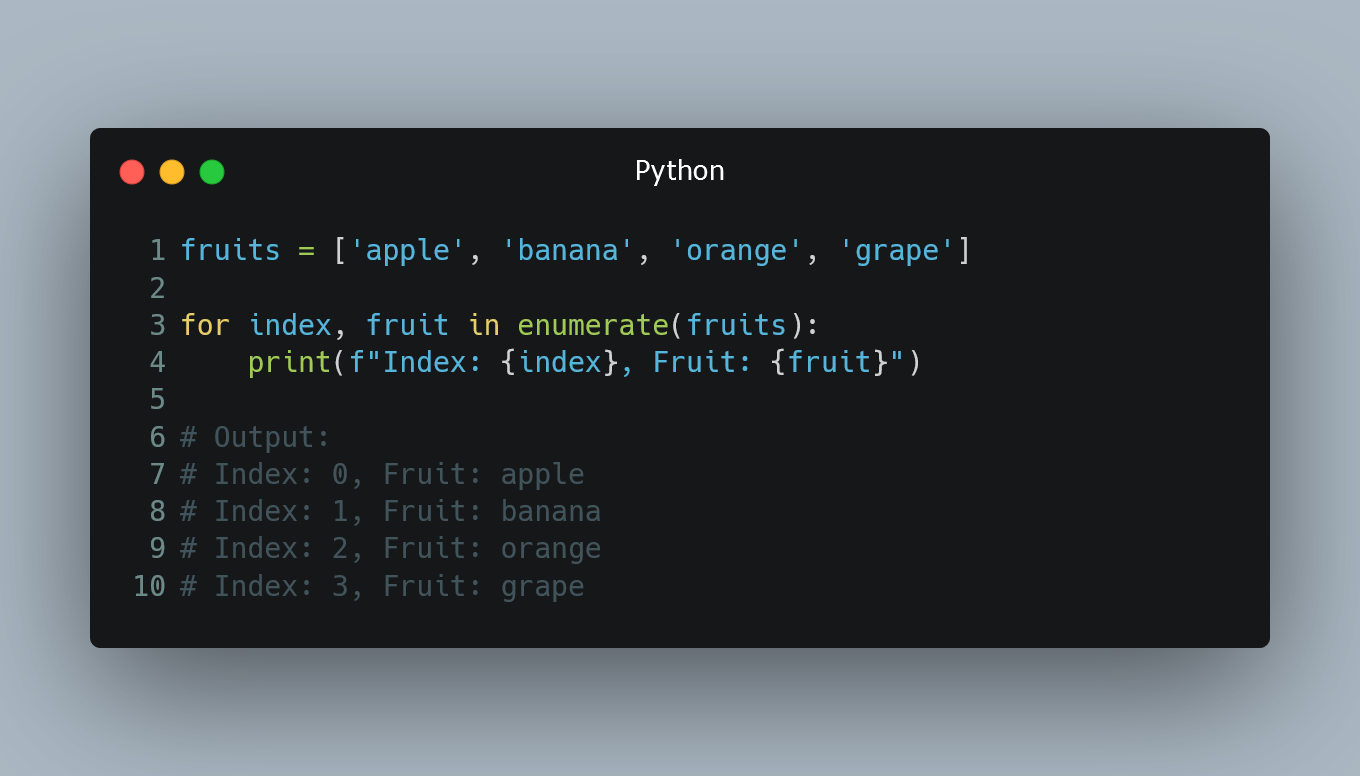
In Python, you can access the index of the current item in a 'for' loop using the built-in enumerate()
function.
#1. Using enumerate()
Function
The enumerate()
function is a powerful Python built-in function that allows you to iterate over a sequence while simultaneously accessing both the elements and their corresponding indices.
fruits = ['apple', 'banana', 'orange', 'grape']
for index, fruit in enumerate(fruits):
print(f"Index: {index}, Fruit: {fruit}")
Output:
Index: 0, Fruit: apple
Index: 1, Fruit: banana
Index: 2, Fruit: orange
Index: 3, Fruit: grape
In this example, enumerate(fruits)
returns an iterator that yields tuples containing both the index and the element in each iteration. By unpacking these tuples in the 'for' loop, you can access both the index and the value of the current item.
#2. Specifying the Start Index
By default, the enumerate()
function starts the index from 0. However, you can specify the start index by providing a second argument to the function.
fruits = ['apple', 'banana', 'orange', 'grape']
for index, fruit in enumerate(fruits, start=1):
print(f"Index: {index}, Fruit: {fruit}")
Output:
Index: 1, Fruit: apple
Index: 2, Fruit: banana
Index: 3, Fruit: orange
Index: 4, Fruit: grape
In this modified example, the 'for' loop starts the index from 1 instead of 0.
#3. Accessing Indices in Nested Iterations
You can also use enumerate()
for nested iterations to access the indices at different levels.
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
for row_idx, row in enumerate(matrix):
for col_idx, value in enumerate(row):
print(f"Row: {row_idx}, Column: {col_idx}, Value: {value}")
Output:
Row: 0, Column: 0, Value: 1
Row: 0, Column: 1, Value: 2
Row: 0, Column: 2, Value: 3
Row: 1, Column: 0, Value: 4
Row: 1, Column: 1, Value: 5
Row: 1, Column: 2, Value: 6
Row: 2, Column: 0, Value: 7
Row: 2, Column: 1, Value: 8
Row: 2, Column: 2, Value: 9
#4. Use Cases
Accessing the index in 'for' loops using enumerate()
is helpful in various situations, such as:
- Keeping track of the position of elements while processing a list.
- Updating specific elements of a list while iterating.
- Handling cases where both the value and the index of the item are required.
0 Comment