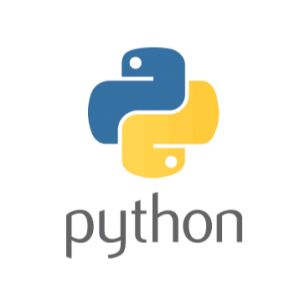
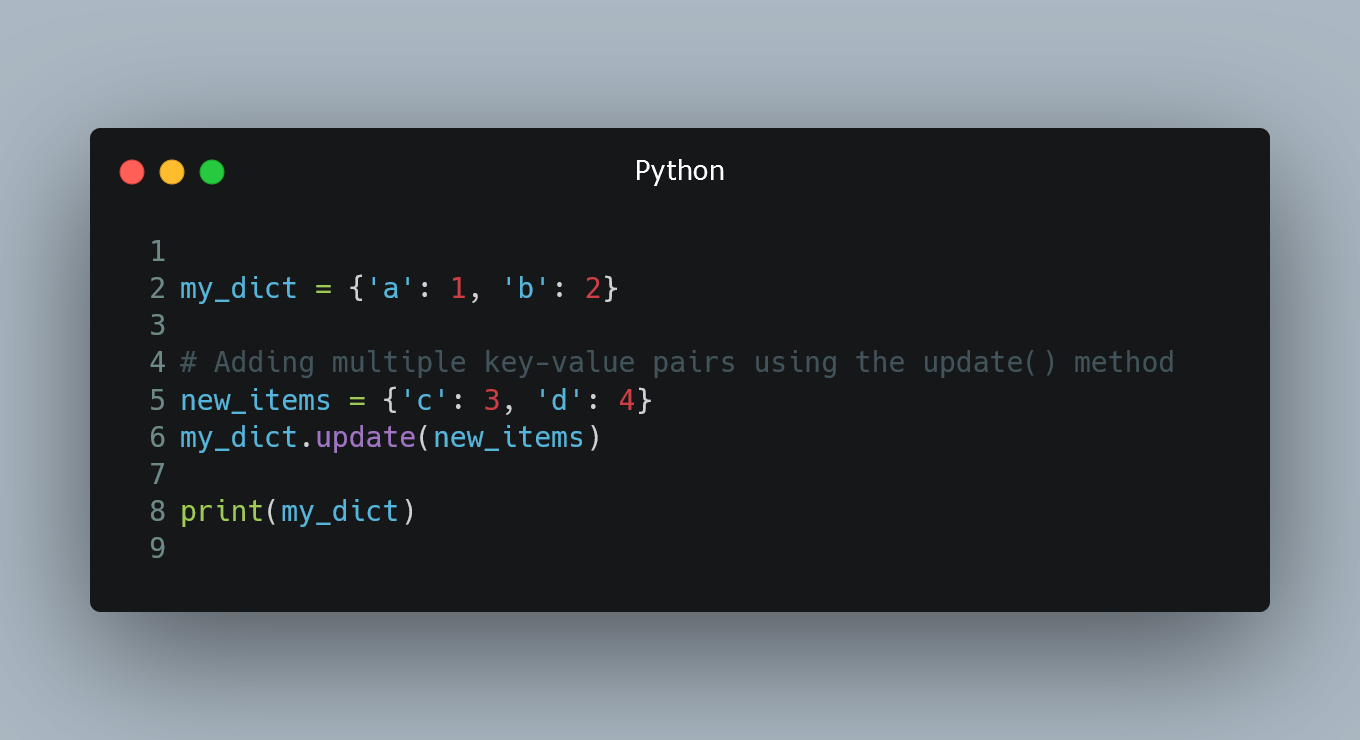
In Python, you can add new keys to a dictionary by simply assigning a value to the desired key.
#1. Adding a Single Key-Value Pair
To add a single key-value pair to a dictionary, use the following syntax:
my_dict = {'a': 1, 'b': 2}
# Adding a new key-value pair
my_dict['c'] = 3
print(my_dict)
Output:
{'a': 1, 'b': 2, 'c': 3}
In this example, the key 'c'
is added to the dictionary with the value 3
.
#2. Adding Multiple Key-Value Pairs
You can add multiple key-value pairs to a dictionary at once using either dictionary unpacking or the update()
method.
Using Dictionary Unpacking:
my_dict = {'a': 1, 'b': 2}
# Adding multiple key-value pairs using dictionary unpacking
new_items = {'c': 3, 'd': 4}
my_dict = {**my_dict, **new_items}
print(my_dict)
Output:
{'a': 1, 'b': 2, 'c': 3, 'd': 4}
Using update()
Method:
my_dict = {'a': 1, 'b': 2}
# Adding multiple key-value pairs using the update() method
new_items = {'c': 3, 'd': 4}
my_dict.update(new_items)
print(my_dict)
Output:
{'a': 1, 'b': 2, 'c': 3, 'd': 4}
Both methods result in the same dictionary with the additional key-value pairs.
#3. Overwriting Existing Keys
If you add a key that already exists in the dictionary, its value will be updated to the new value.
my_dict = {'a': 1, 'b': 2}
# Overwriting an existing key
my_dict['a'] = 10
print(my_dict)
Output:
{'a': 10, 'b': 2}
In this example, the value associated with the key 'a'
is changed from 1
to 10
.
0 Comment