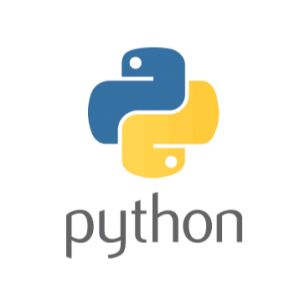
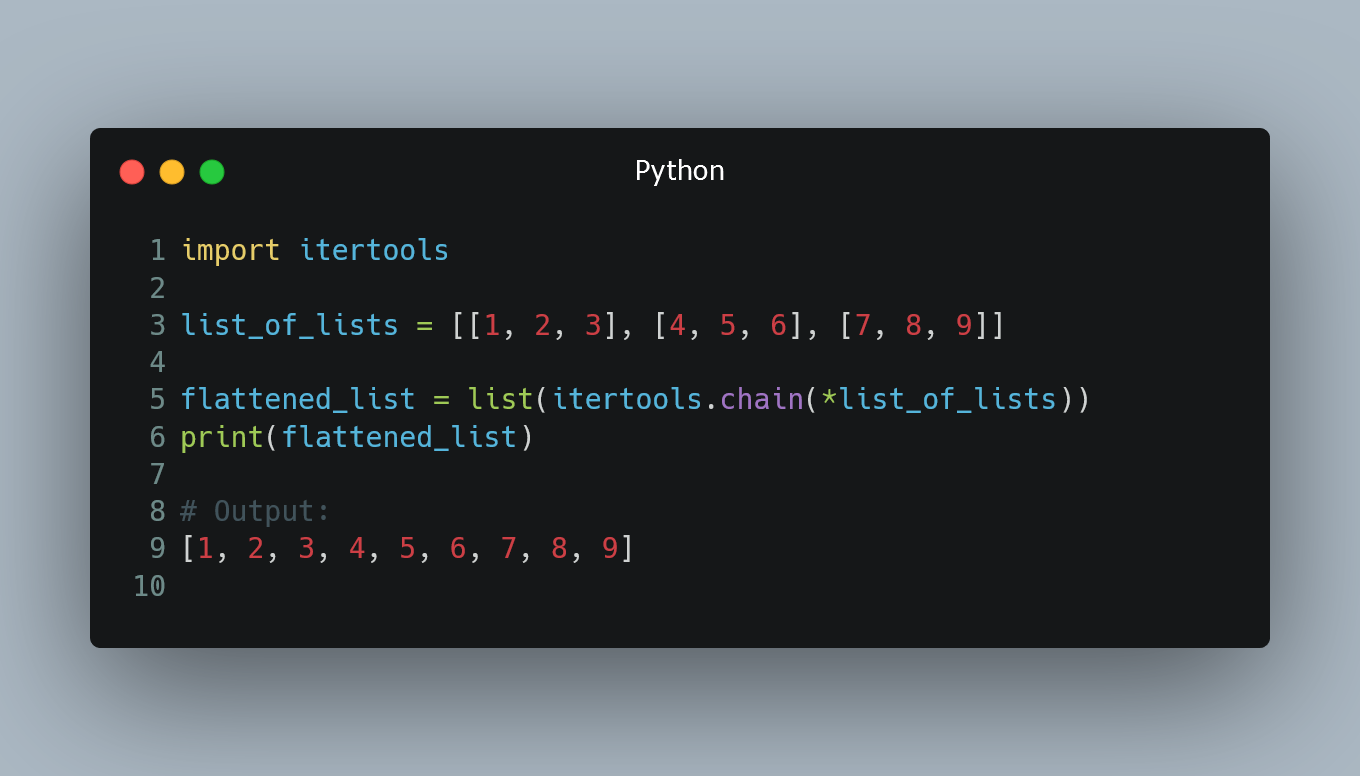
To flatten a list of lists into a single, one-dimensional list in Python, you can use list comprehension or the itertools.chain()
function.
#1. Using List Comprehension
List comprehension is a concise and readable way to create a new list by iterating over the elements of a list of lists and flattening them.
list_of_lists = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened_list = [element for sublist in list_of_lists for element in sublist]
print(flattened_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
In this example, the nested list is flattened into a single list using list comprehension. The outer loop iterates over each sublist, and the inner loop iterates over the elements of each sublist, adding them to the flattened_list
.
#2. Using itertools.chain()
The itertools.chain()
function is available in the itertools
module and provides a memory-efficient way to flatten a list of lists.
import itertools
list_of_lists = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened_list = list(itertools.chain(*list_of_lists))
print(flattened_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
The *list_of_lists
syntax unpacks the list of lists as separate arguments to itertools.chain()
, effectively flattening it.
#3. Nested Lists with Varying Lengths
Both methods described above work perfectly for nested lists with uniform lengths. However, if your nested lists have varying lengths, the itertools.chain()
function may provide more consistent results.
list_of_lists = [[1, 2], [3, 4, 5], [6, 7, 8, 9]]
flattened_list = list(itertools.chain(*list_of_lists))
print(flattened_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
In this example, the nested lists have varying lengths, and itertools.chain()
still successfully flattens them.
#4. Caution with Nested Lists
Keep in mind that when flattening a list of lists, you lose the original structure of the nested lists. If preserving the original structure is necessary, you may consider using more advanced techniques, such as recursion.
0 Comment