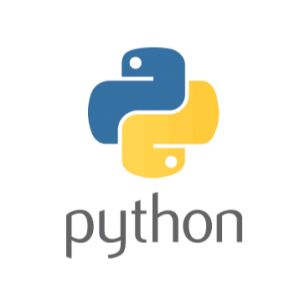
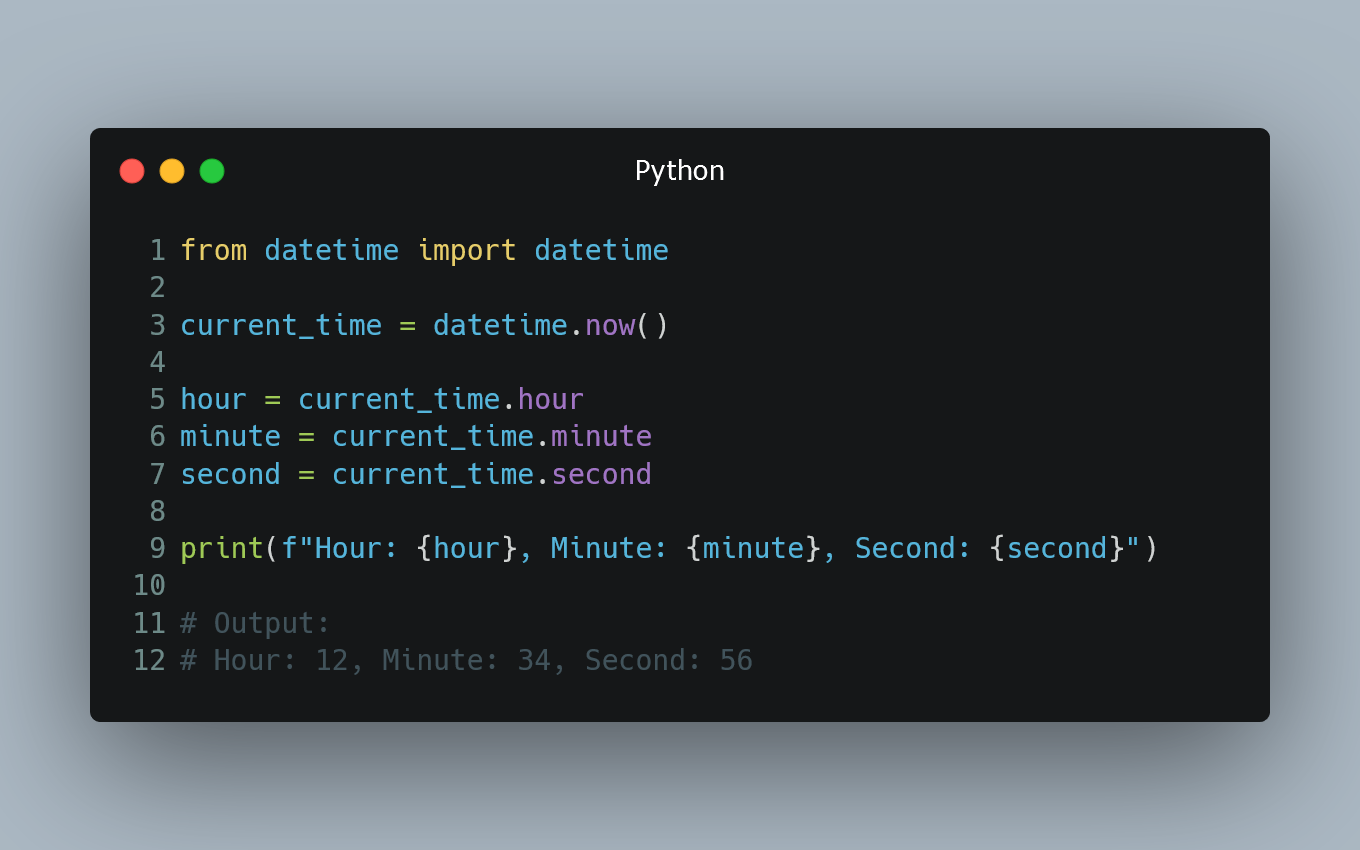
In Python, you can get the current time using the datetime
module. The datetime
module provides various functions and classes to work with dates and times, allowing you to retrieve the current time with precision.
#1. Using datetime.now()
The datetime.now()
function returns a datetime
object representing the current date and time.
from datetime import datetime
current_time = datetime.now()
print(current_time)
Output:
2023-07-28 12:34:56.789012
The datetime.now()
function includes the year, month, day, hour, minute, second, and microsecond in the output.
#2. Formatting the Current Time
If you want to display the current time in a specific format, you can use the strftime()
method. It allows you to format the datetime
object as a string according to your desired format.
from datetime import datetime
current_time = datetime.now()
# Format the current time
formatted_time = current_time.strftime("%Y-%m-%d %H:%M:%S")
print(formatted_time)
Output:
2023-07-28 12:34:56
In this example, the strftime()
method is used to format the current time in the format "YYYY-MM-DD HH:MM:SS".
#3. Time Components
If you only need specific components of the current time (e.g., hour, minute, or second), you can access them directly from the datetime
object.
from datetime import datetime
current_time = datetime.now()
hour = current_time.hour
minute = current_time.minute
second = current_time.second
print(f"Hour: {hour}, Minute: {minute}, Second: {second}")
Output:
Hour: 12, Minute: 34, Second: 56
The hour
, minute
, and second
attributes provide the corresponding components of the current time.
0 Comment