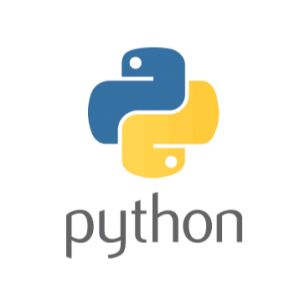
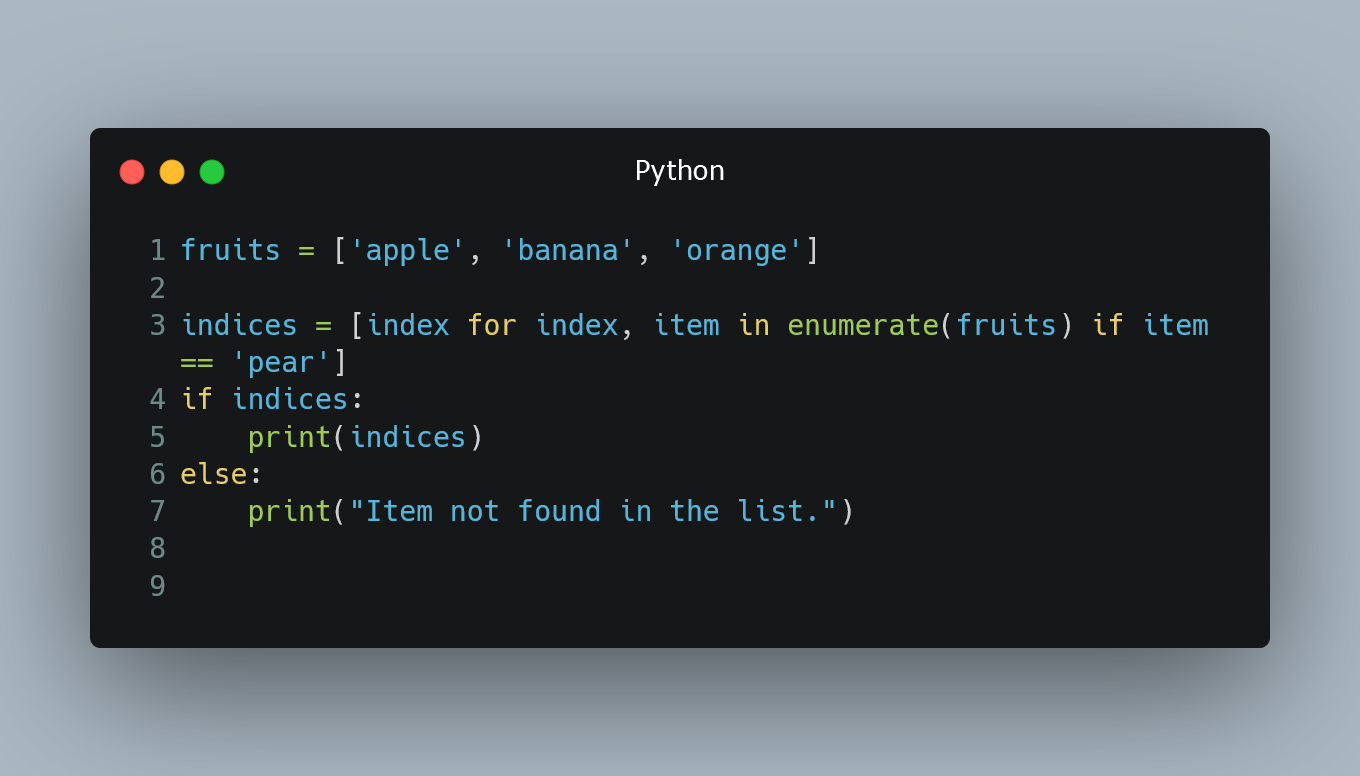
To find the index of an item in a list in Python, you can use the index()
method or a combination of built-in functions such as enumerate()
and list comprehension.
#1. Using the index()
Method
The index()
method is a simple and straightforward way to find the index of an item in a list. It returns the index of the first occurrence of the specified item.
fruits = ['apple', 'banana', 'orange', 'banana']
# Find the index of 'banana'
index = fruits.index('banana')
print(index) # Output: 1
In this example, the index()
method returns the index of the first occurrence of 'banana,' which is 1.
#1.1 Handling Non-Existing Items
If the item is not present in the list, the index()
method raises a ValueError
. To handle this scenario, you can use a try-except block.
fruits = ['apple', 'banana', 'orange']
try:
index = fruits.index('pear')
print(index)
except ValueError:
print("Item not found in the list.")
#2. Using enumerate()
with List Comprehension
Another way to find the index of an item is by using enumerate()
with list comprehension. This method allows you to find the indices of all occurrences of the item.
fruits = ['apple', 'banana', 'orange', 'banana']
# Find the indices of 'banana'
indices = [index for index, item in enumerate(fruits) if item == 'banana']
print(indices) # Output: [1, 3]
In this example, the list comprehension iterates through the elements of the list, and for each element that matches 'banana,' it appends the corresponding index to the indices
list.
#2.1 Handling Non-Existing Items
If the item is not present in the list, the list comprehension will result in an empty list.
fruits = ['apple', 'banana', 'orange']
indices = [index for index, item in enumerate(fruits) if item == 'pear']
if indices:
print(indices)
else:
print("Item not found in the list.")
0 Comment