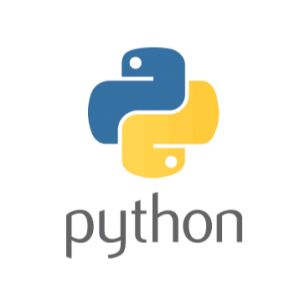
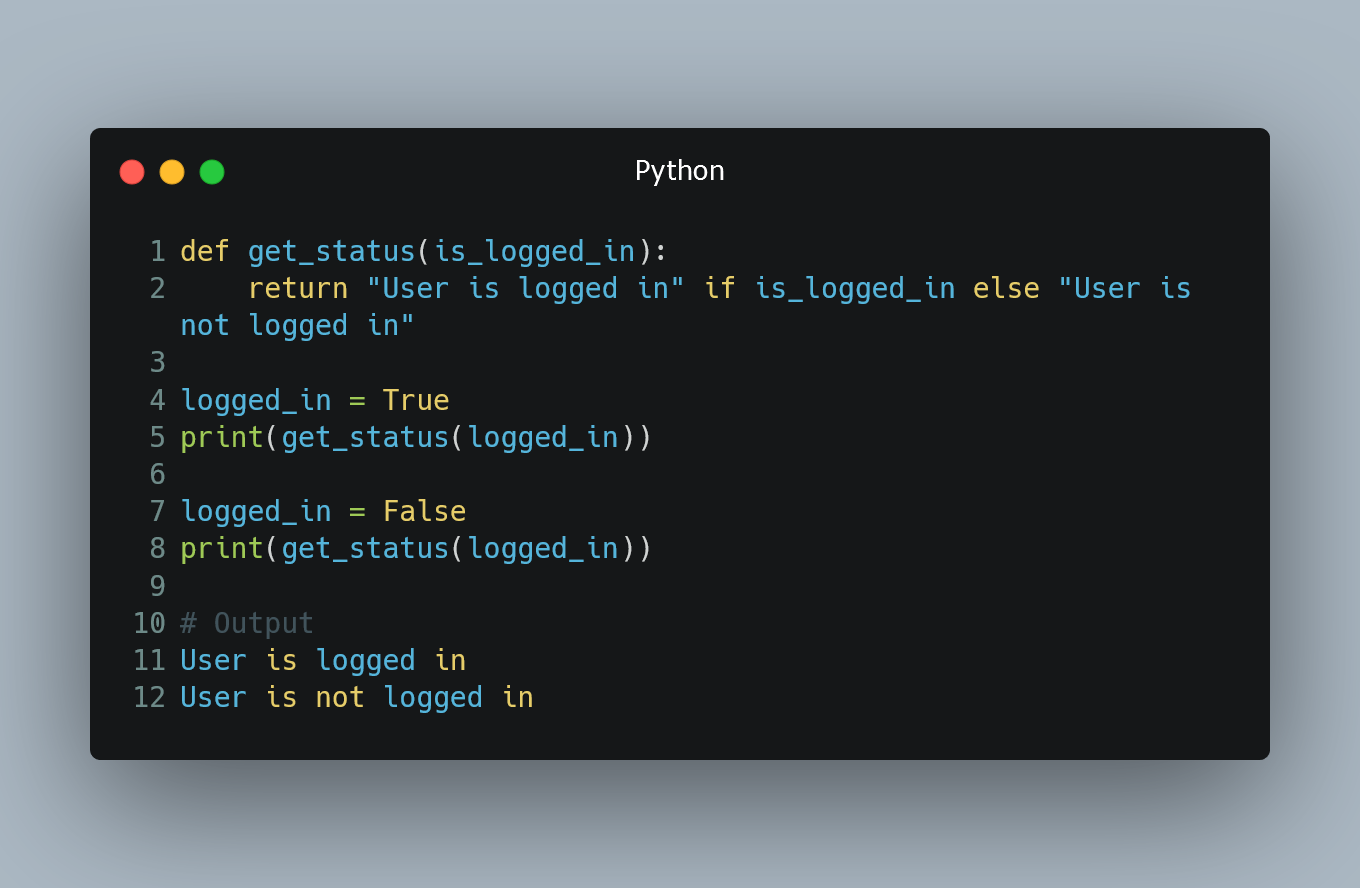
Python does have a ternary conditional operator, which allows you to write a concise one-liner for simple conditional expressions.
result_if_true if condition else result_if_false
Let's explore how to use the ternary conditional operator in Python.
Syntax
The syntax of the ternary conditional operator is straightforward. It consists of three parts:
- The condition: This is the expression that evaluates to either True or False.
- The result_if_true: This is the value returned if the condition is True.
- The result_if_false: This is the value returned if the condition is False.
Example 1: Basic Usage
Here's a simple example to illustrate the basic usage of the ternary conditional operator:
x = 10
message = "x is greater than 5" if x > 5 else "x is less than or equal to 5"
print(message)
Output:
x is greater than 5
In this example, if the condition x > 5
is True, the value "x is greater than 5"
will be assigned to the variable message
. Otherwise, the value "x is less than or equal to 5"
will be assigned.
Example 2: Using Ternary Operator with Functions
The ternary conditional operator is often used to call functions conditionally:
def get_status(is_logged_in):
return "User is logged in" if is_logged_in else "User is not logged in"
logged_in = True
print(get_status(logged_in))
logged_in = False
print(get_status(logged_in))
Output:
User is logged in
User is not logged in
In this example, the get_status()
function takes a boolean parameter is_logged_in
. If is_logged_in
is True, it returns the message "User is logged in," otherwise it returns "User is not logged in."
Example 3: Nesting Ternary Operators
You can also nest ternary operators for more complex conditions. However, be cautious as excessive nesting can reduce code readability.
num = 15
message = "even" if num % 2 == 0 else "odd" if num % 3 == 0 else "neither even nor odd"
print(f"{num} is {message}.")
Output:
15 is odd.
In this example, the ternary operators are nested to check if num
is even, odd, or neither even nor odd.
0 Comment