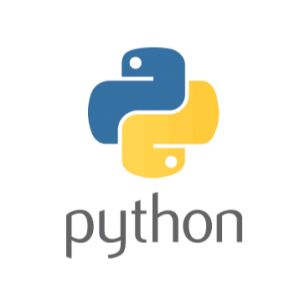
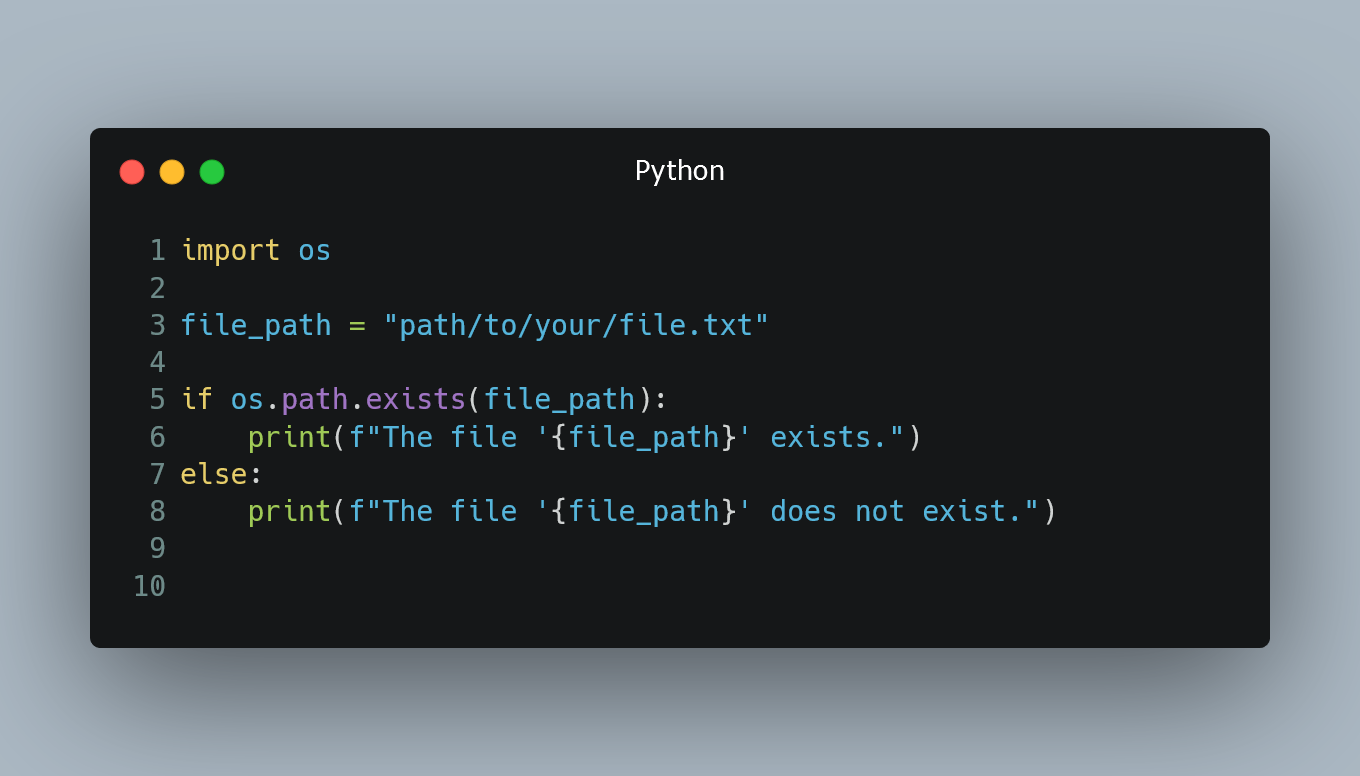
To check whether a file exists in Python without raising exceptions, you can use various methods provided by the os
and os.path
modules.
#1. Using os.path.exists()
The os.path.exists()
function is a straightforward way to check if a file exists in a given path.
import os
file_path = "path/to/your/file.txt"
if os.path.exists(file_path):
print(f"The file '{file_path}' exists.")
else:
print(f"The file '{file_path}' does not exist.")
#2. Using os.path.isfile()
If you specifically want to check if the path points to a regular file (not a directory or a symbolic link), you can use the os.path.isfile()
function.
import os
file_path = "path/to/your/file.txt"
if os.path.isfile(file_path):
print(f"The file '{file_path}' exists and is a regular file.")
else:
print(f"The file '{file_path}' does not exist or is not a regular file.")
#3. Using os.access()
The os.access()
function can be used to check whether a file has specific permissions, but it can also be used to check file existence.
import os
file_path = "path/to/your/file.txt"
if os.access(file_path, os.F_OK):
print(f"The file '{file_path}' exists.")
else:
print(f"The file '{file_path}' does not exist.")
In the above code, os.F_OK
is a constant representing the existence test.
#4. Using pathlib.Path
The pathlib
module provides an object-oriented approach to file path manipulation. You can use the Path.exists()
method to check for file existence.
from pathlib import Path
file_path = Path("path/to/your/file.txt")
if file_path.exists():
print(f"The file '{file_path}' exists.")
else:
print(f"The file '{file_path}' does not exist.")
#5. Caution with Filesystem Race Conditions
Keep in mind that file existence checks do not guarantee that the file will remain present at a later time. In a multi-threaded or multi-process environment, the file's existence might change between the check and any subsequent file operations. Be mindful of potential race conditions when working with files.
0 Comment