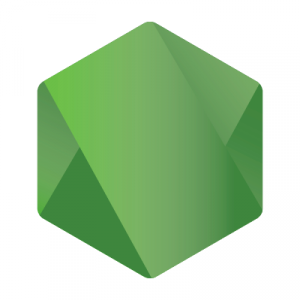
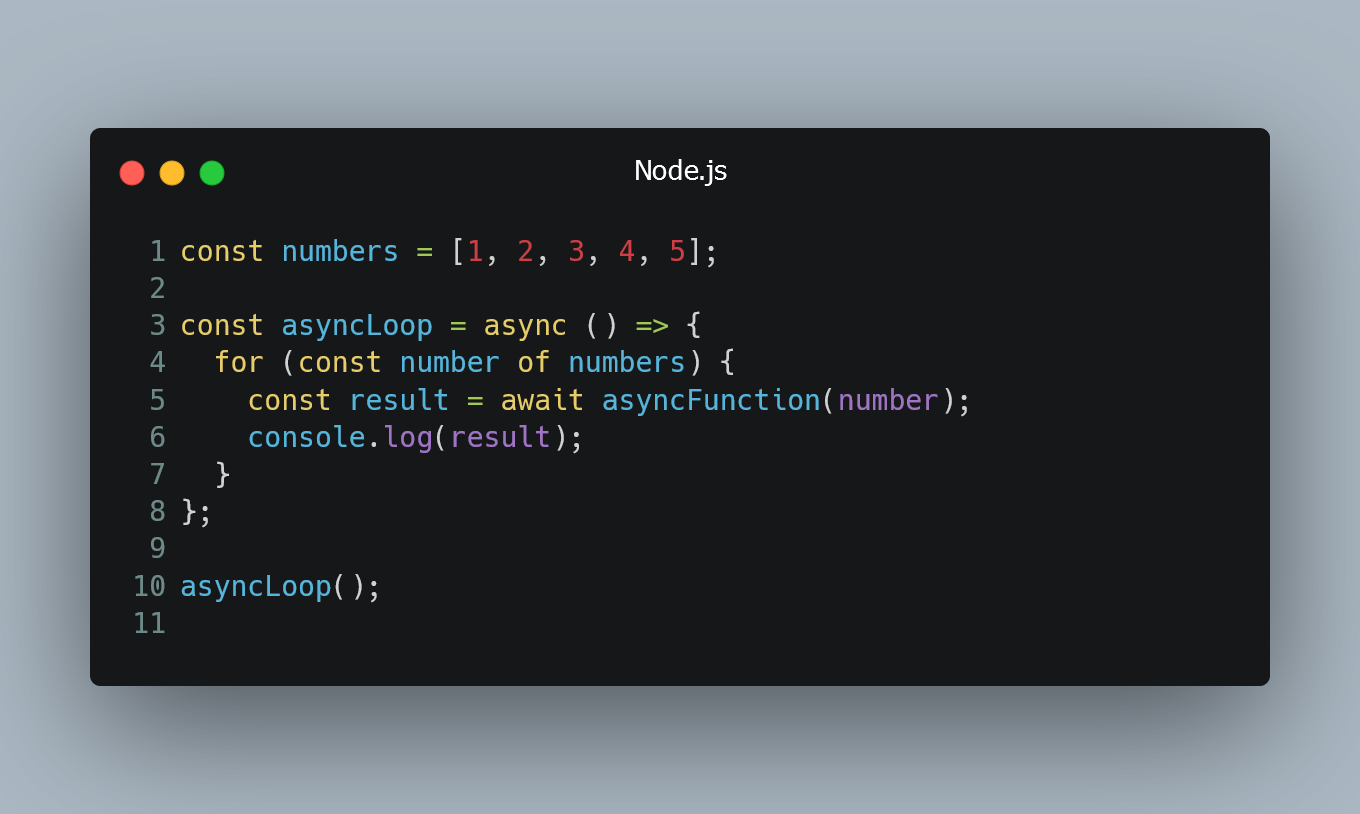
In JavaScript, using async/await with a forEach loop can be a bit tricky, as forEach
is not promise-aware and cannot be used directly with async functions.
Setting Up a Simple Async Function
Before we dive into the loop methods, let's define a simple async function that we will use in our examples. This function just simulates an asynchronous operation using setTimeout
.
const asyncFunction = (i) => {
return new Promise((resolve) => {
setTimeout(() => {
resolve(i);
}, 100);
});
};
Using Async/Await with a For...Of Loop
One straightforward way to work with async/await inside a loop is by using a for...of
loop. This loop allows us to wait for a promise to resolve before continuing with the next iteration.
const numbers = [1, 2, 3, 4, 5];
const asyncLoop = async () => {
for (const number of numbers) {
const result = await asyncFunction(number);
console.log(result);
}
};
asyncLoop();
In this example, each asynchronous operation will complete before the next one starts.
Using Async/Await with Array.prototype.map and Promise.all
If you want to perform all operations concurrently, you can use Array.prototype.map
to create an array of promises, and then use Promise.all
to wait for all of them to resolve.
const numbers = [1, 2, 3, 4, 5];
const asyncParallel = async () => {
const promises = numbers.map(asyncFunction);
const results = await Promise.all(promises);
console.log(results);
};
asyncParallel();
In this example, all asynchronous operations start at the same time, and the Promise.all
function waits for all of them to complete.
0 Comment