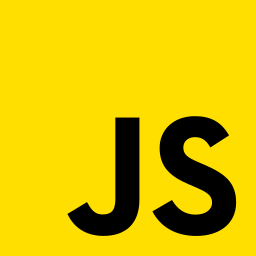
The "use strict" directive is a pragma in JavaScript that enables a strict mode for the code. It helps developers write safer and more reliable code by enforcing stricter rules and preventing certain mistakes.
Enabling Strict Mode with "use strict"
To enable strict mode in a JavaScript file or function, you can add the "use strict" directive at the beginning of the file or function.
Example
"use strict";
function myFunction() {
// Code in strict mode
}
Strict Mode Restrictions and Enhancements
"use strict" imposes certain restrictions and enhancements on JavaScript code:
1. Variable Declaration
In strict mode, all variables must be declared using var
, let
, or const
. Declaring variables without these keywords will raise an error.
2. Prevents Implicit Global Variables
In non-strict mode, if you accidentally forget to declare a variable, it will become a global variable, potentially causing unexpected behavior. Strict mode prevents this and raises an error when undeclared variables are used.
3. Prohibits Deleting Variables and Functions
In strict mode, you cannot delete variables or function names. Attempting to do so will result in an error.
4. Disallows Duplicate Parameter Names
Strict mode does not allow duplicate parameter names in function declarations or expressions.
5. "this" Value in Functions
In strict mode, the value of this
inside a function is not automatically converted to the global object (e.g., window
in browsers). If a function is called without a specific context, this
will be undefined
.
6. Forbids Octal Numeric Literals
Strict mode prohibits the use of octal literals, such as 010
, as they can lead to confusion and bugs.
The Reasoning Behind "use strict"
The "use strict" directive was introduced in ECMAScript 5 (ES5) to address some of the language's historical issues and to make it more robust. By enabling strict mode, developers are encouraged to follow best practices and avoid common pitfalls, leading to safer, more maintainable code.
Strict mode also allows JavaScript engines to make certain optimizations, improving performance for code written in strict mode.
0 Comment