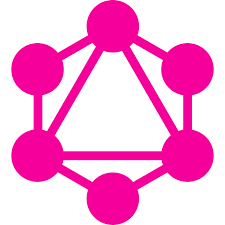
In GraphQL, the exclamation point (!
) is a significant symbol that conveys important information about the fields in your schema. It is used to denote non-nullability for both scalar types and custom object types.
Denoting Non-Nullable Scalar Types
In GraphQL, scalar types represent simple data types like strings, numbers, and booleans. By default, scalar types are nullable, meaning they can have a value of null
. However, you can explicitly specify that a scalar type is non-nullable by adding an exclamation point at the end of its definition.
Nullable Scalar Type
type ExampleType {
someField: String
}
In this example, someField
is a nullable scalar type because it doesn't have an exclamation point.
Non-Nullable Scalar Type
type ExampleType {
someField: String!
}
In this example, someField
is a non-nullable scalar type because it has an exclamation point at the end.
Ensuring Non-Nullable Object Fields
Similarly, you can use the exclamation point to denote non-nullable fields in custom object types.
Nullable Object Field
type ExampleType {
someObject: AnotherType
}
Here, someObject
is a nullable object field because it doesn't have an exclamation point.
Non-Nullable Object Field
type ExampleType {
someObject: AnotherType!
}
With the exclamation point, someObject
becomes a non-nullable object field.
Dealing with Non-Nullable Fields in Queries
When a field is marked as non-nullable in the schema, the GraphQL server ensures that it always returns a value for that field. If, for any reason, a non-nullable field cannot be resolved during a query, an error will be returned.
When querying non-nullable fields, you don't need to use an exclamation point in the query itself. The exclamation point is only used in the schema to define the non-nullable nature of the field.
0 Comment