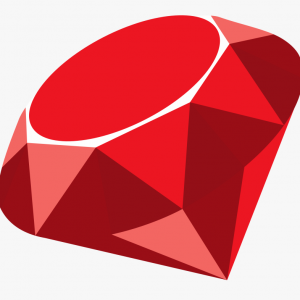
In Ruby, you can easily calculate the sum of an array of numbers using built-in methods.
Using the sum Method
What is sum?
The sum
method is a convenient way to calculate the sum of all elements in an array of numbers.
Syntax of sum
array.sum
Here, array
is the array of numbers for which you want to calculate the sum.
Example
numbers = [1, 2, 3, 4, 5]
total = numbers.sum
puts total # Output: 15
In this example, the sum
method is used on the numbers
array to calculate the sum of all its elements. The result is stored in the total
variable and then printed to the console.
Using the inject Method
What is inject?
The inject
method, also known as reduce
, is another way to calculate the sum of an array of numbers. It applies a binary operation on each element of the array, accumulating the result as it iterates through the elements.
Syntax of inject
array.inject(:+)
Here, array
is the array of numbers, and :+
is the binary operation for addition.
Example
numbers = [1, 2, 3, 4, 5]
total = numbers.inject(:+)
puts total # Output: 15
In this example, the inject
method with the :+
symbol is used on the numbers
array to calculate the sum of all its elements. The result is stored in the total
variable and then printed to the console.
0 Comment