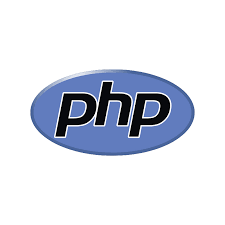
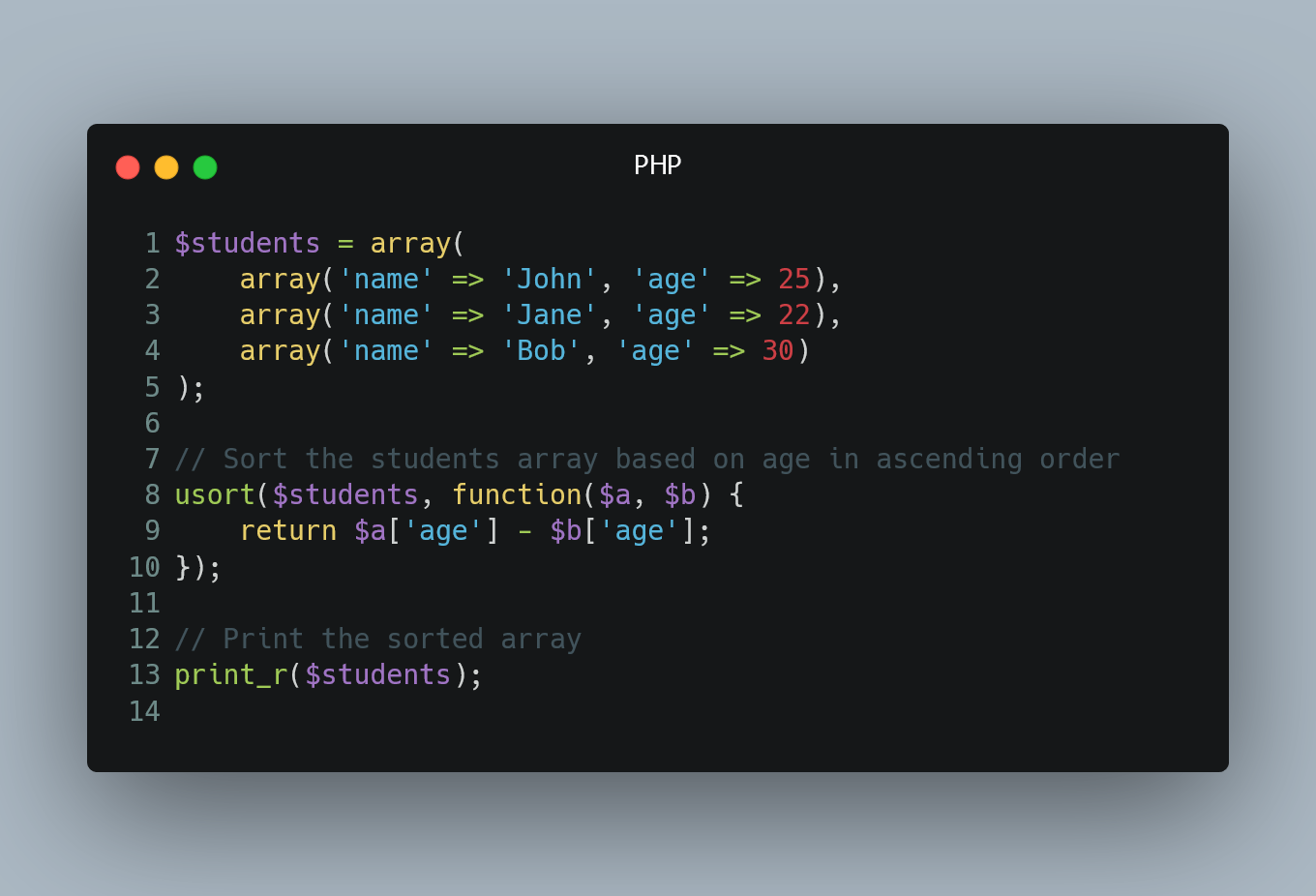
In PHP, you can sort a multi-dimensional array by its values using various sorting functions available.
Using usort() Function
The usort() Function
The usort() function is a built-in PHP function that sorts an array using a user-defined comparison function. This function allows you to sort multi-dimensional arrays based on the values of a specific key.
Syntax of usort()
usort($array, function($a, $b) {
// Comparison logic here
});
Example
$students = array(
array('name' => 'John', 'age' => 25),
array('name' => 'Jane', 'age' => 22),
array('name' => 'Bob', 'age' => 30)
);
// Sort the students array based on age in ascending order
usort($students, function($a, $b) {
return $a['age'] - $b['age'];
});
// Print the sorted array
print_r($students);
Output:
Array
(
[0] => Array
(
[name] => Jane
[age] => 22
)
[1] => Array
(
[name] => John
[age] => 25
)
[2] => Array
(
[name] => Bob
[age] => 30
)
)
In this example, the usort() function is used with an anonymous function as the comparison logic. The array is sorted based on the 'age' key in ascending order.
Using array_multisort() Function
The array_multisort() Function
The array_multisort() function is another built-in PHP function that can sort multiple arrays or multi-dimensional arrays simultaneously based on the values.
Syntax of array_multisort()
array_multisort($array1, $sortingOrder1, $array2, $sortingOrder2, ...);
Example
$names = array('John', 'Jane', 'Bob');
$ages = array(25, 22, 30);
// Sort the arrays simultaneously based on ages in ascending order
array_multisort($ages, SORT_ASC, $names, SORT_ASC);
// Print the sorted arrays
print_r($names);
print_r($ages);
Output:
Array
(
[0] => Jane
[1] => John
[2] => Bob
)
Array
(
[0] => 22
[1] => 25
[2] => 30
)
In this example, the array_multisort() function is used to sort both the 'names' and 'ages' arrays simultaneously based on the 'ages' in ascending order.
0 Comment