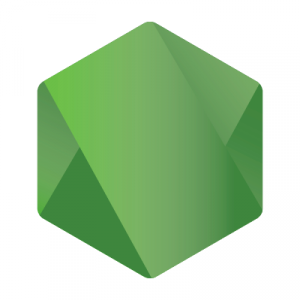
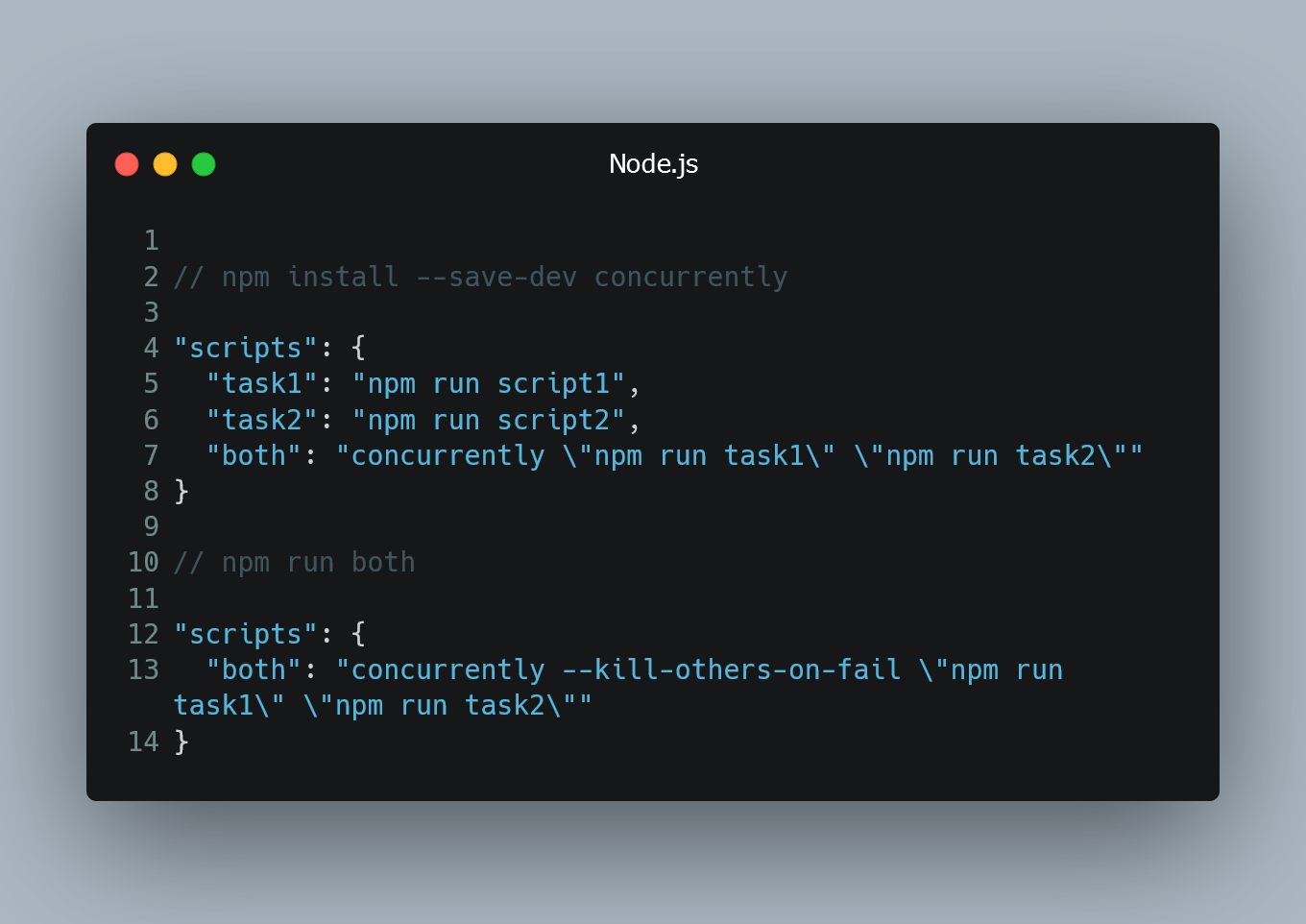
In Node.js, you can run multiple NPM scripts simultaneously to execute tasks in parallel. This can be useful when you have multiple tasks that can be executed independently and don't depend on each other.
Using the "concurrently" Package
To run multiple NPM scripts in parallel, you can use the "concurrently" package, which allows you to specify the scripts you want to run together in a single command. First, you need to install the "concurrently" package as a development dependency:
npm install --save-dev concurrently
Next, you can add the scripts you want to run in parallel to your package.json
file. For example:
"scripts": {
"task1": "npm run script1",
"task2": "npm run script2",
"both": "concurrently \"npm run task1\" \"npm run task2\""
}
In this example, we have two NPM scripts, "task1" and "task2", which are defined as separate scripts. The "both" script uses the "concurrently" package to run "task1" and "task2" together in parallel.
Running the Parallel Scripts
To execute the parallel scripts, simply run the "both" script using the npm run
command:
npm run both
The "concurrently" package will start both "task1" and "task2" at the same time, and you'll see their respective outputs in the terminal.
Additional "concurrently" Options
The "concurrently" package provides additional options to customize the behavior of parallel script execution. For example, you can use the --kill-others-on-fail
flag to stop other scripts if one of them fails:
"scripts": {
"both": "concurrently --kill-others-on-fail \"npm run task1\" \"npm run task2\""
}
You can find more options in the "concurrently" documentation on the npm website.
1 Comment
Another option to use pm2 cluster mode