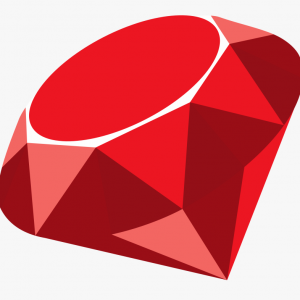
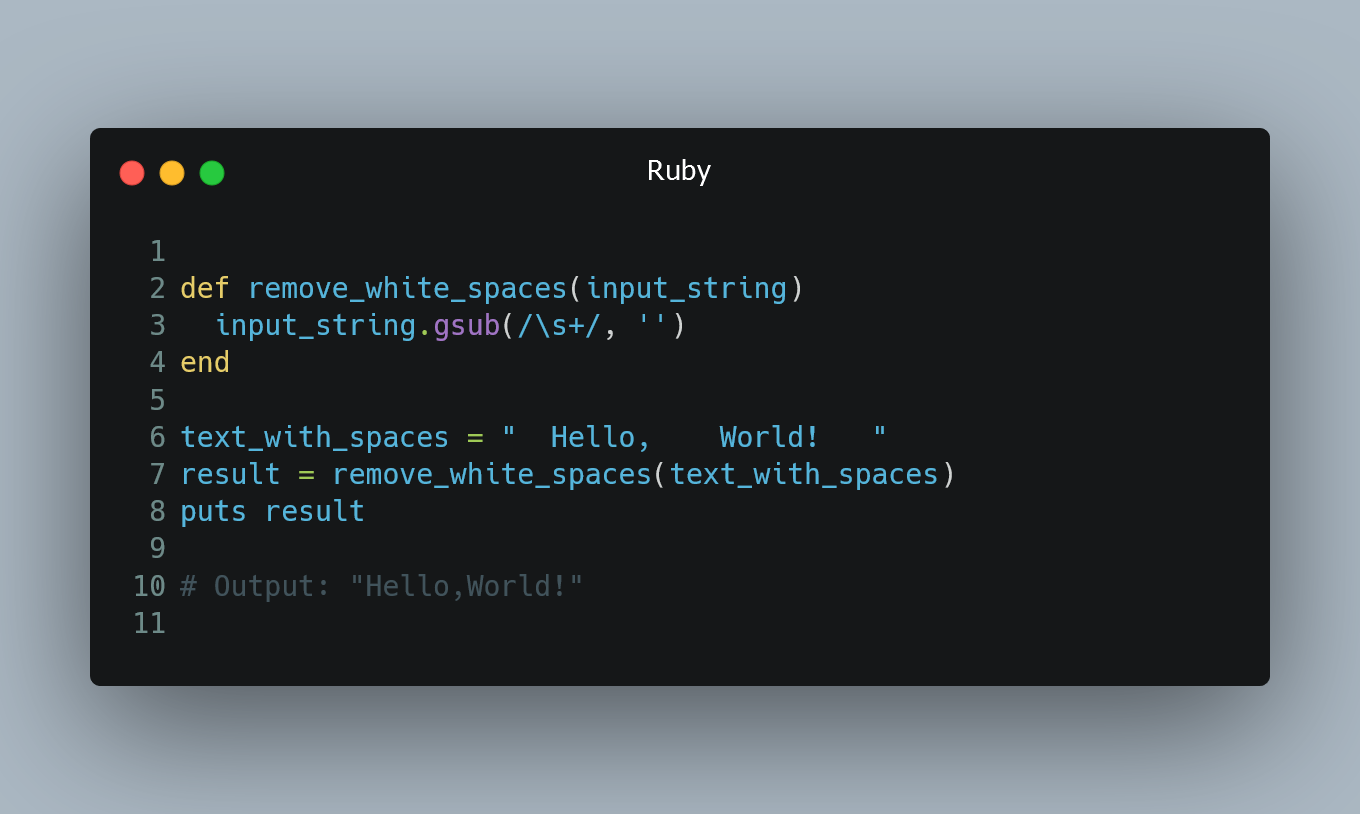
In Ruby, you can create a custom function to remove all white spaces from a given string.
Using the gsub Method with Regular Expression
What is gsub?
The gsub
method is a built-in Ruby string method used for substitution. When combined with a regular expression, it can be used to remove white spaces from a string.
Syntax of gsub
string.gsub(/\s+/, '')
Here, string
is the original string containing white spaces, and /\s+/
is the regular expression pattern to match one or more white spaces.
Example
def remove_white_spaces(input_string)
input_string.gsub(/\s+/, '')
end
text_with_spaces = " Hello, World! "
result = remove_white_spaces(text_with_spaces)
puts result # Output: "Hello,World!"
In this example, we define a custom function remove_white_spaces
that takes an input_string
. Inside the function, we use the gsub
method with the regular expression /s+/
to match one or more white spaces and replace them with an empty string.
When we call the function with the text_with_spaces
, it removes all the white spaces, and the output is "Hello,World!".
Using the delete Method
What is delete?
The delete
method is another built-in Ruby string method that can be used to remove specified characters from a string. It can be used to remove all white spaces by passing the space character and any other white space characters as arguments.
Syntax of delete
string.delete(' \t\n\r')
Here, string
is the original string containing white spaces, and ' \t\n\r'
are the characters to be removed.
Example
def remove_white_spaces(input_string)
input_string.delete(' \t\n\r')
end
text_with_spaces = " Hello, World! "
result = remove_white_spaces(text_with_spaces)
puts result # Output: "Hello,World!"
In this example, we define a custom function remove_white_spaces
that takes an input_string
. Inside the function, we use the delete
method with the argument ' \t\n\r'
, which includes the space character, tab, newline, and carriage return, to remove all the specified white space characters from the input string.
0 Comment