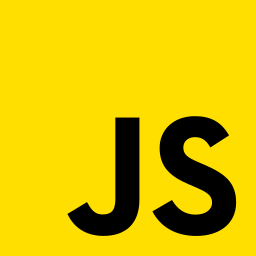
In JavaScript, when working with asynchronous functions that involve network requests or other time-consuming operations, you often need to return the response from the asynchronous call.
Using Callbacks
Callbacks are a traditional way to handle asynchronous results in JavaScript. You can pass a callback function as an argument to the asynchronous function, and then invoke the callback with the response once it's available.
Example
function fetchData(callback) {
// Simulating an asynchronous operation with setTimeout
setTimeout(() => {
const data = { id: 1, name: "John Doe" };
callback(data);
}, 1000); // Simulate a 1-second delay
}
function handleResponse(response) {
console.log(response);
}
fetchData(handleResponse); // Output: { id: 1, name: "John Doe" }
Using Promises
Promises provide a cleaner and more structured way to handle asynchronous results in JavaScript. You can wrap the asynchronous operation in a Promise and use resolve
to return the response when it's ready.
Example
function fetchData() {
return new Promise((resolve) => {
// Simulating an asynchronous operation with setTimeout
setTimeout(() => {
const data = { id: 1, name: "John Doe" };
resolve(data);
}, 1000); // Simulate a 1-second delay
});
}
fetchData().then((response) => {
console.log(response); // Output: { id: 1, name: "John Doe" }
});
Using async/await
async/await is a modern and more readable way to work with asynchronous code. It allows you to write asynchronous code that looks like synchronous code, making it easier to follow and reason about.
Example
function fetchData() {
return new Promise((resolve) => {
// Simulating an asynchronous operation with setTimeout
setTimeout(() => {
const data = { id: 1, name: "John Doe" };
resolve(data);
}, 1000); // Simulate a 1-second delay
});
}
async function getData() {
const response = await fetchData();
console.log(response); // Output: { id: 1, name: "John Doe" }
}
getData();
By using callbacks, Promises, or async/await, you can effectively return the response from an asynchronous call in JavaScript.
0 Comment