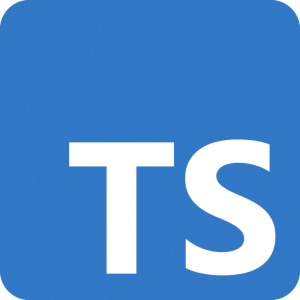
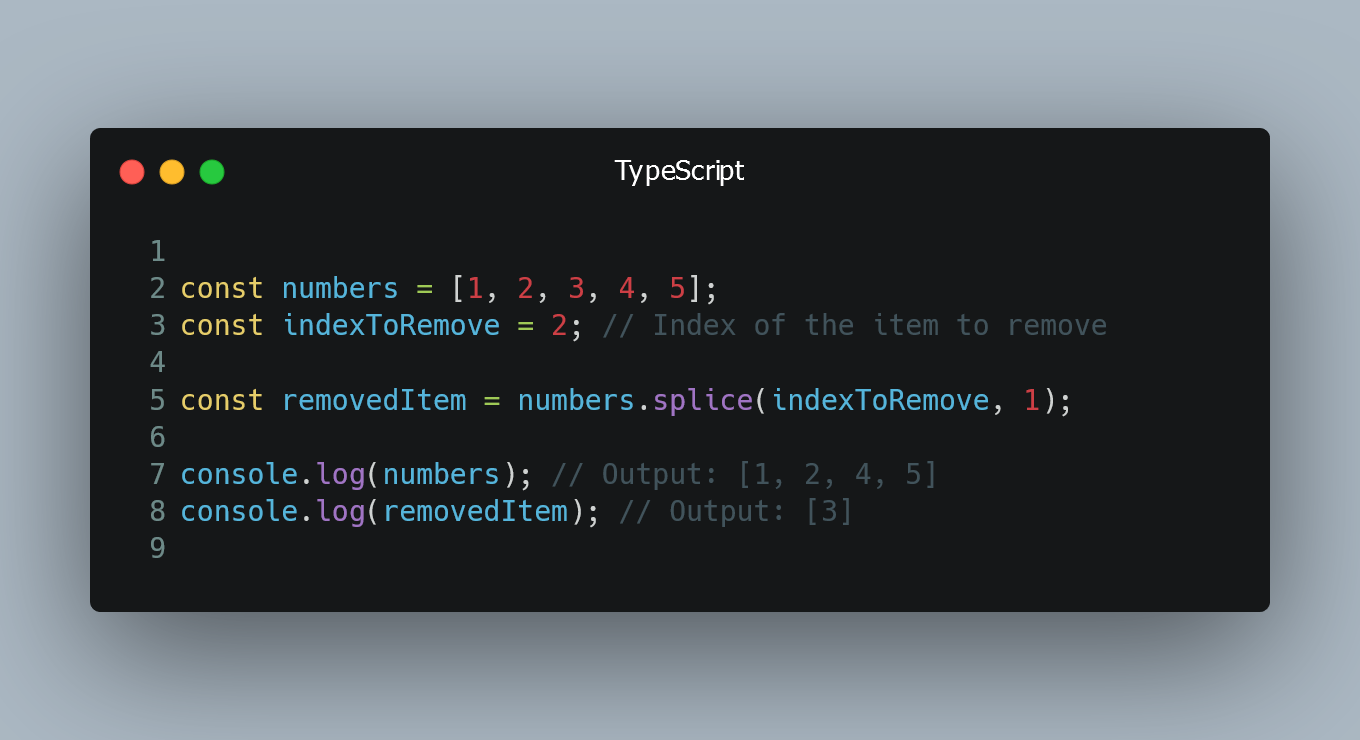
In TypeScript, you can remove an item from an array using different methods, depending on your specific requirements and the version of TypeScript you are using.
#1. Using Array.prototype.splice()
The splice()
method is a built-in JavaScript method that can be used to add or remove elements from an array.
const numbers = [1, 2, 3, 4, 5];
const indexToRemove = 2; // Index of the item to remove
numbers.splice(indexToRemove, 1);
console.log(numbers); // Output: [1, 2, 4, 5]
In this example, we have an array called numbers
. We use the splice()
method to remove one element from the array at the specified indexToRemove
.
#2. Using Array.prototype.filter()
The filter()
method is another approach to remove an item from an array by creating a new array with elements that meet specific conditions.
const numbers = [1, 2, 3, 4, 5];
const itemToRemove = 3; // Item to remove
const filteredNumbers = numbers.filter((item) => item !== itemToRemove);
console.log(filteredNumbers); // Output: [1, 2, 4, 5]
In this example, we use the filter()
method to create a new array filteredNumbers
, excluding the itemToRemove
from the original array.
#3. Using Array.prototype.slice() and Spread Operator
The slice()
method can also be used in combination with the spread operator to remove an item from an array without modifying the original array.
const numbers = [1, 2, 3, 4, 5];
const indexToRemove = 2; // Index of the item to remove
const numbersWithoutItem = [...numbers.slice(0, indexToRemove), ...numbers.slice(indexToRemove + 1)];
console.log(numbersWithoutItem); // Output: [1, 2, 4, 5]
In this example, we use the slice()
method to create two slices of the array, excluding the item at indexToRemove
. Then, we use the spread operator to merge the slices into a new array numbersWithoutItem
.
#4. Using Array.prototype.splice() with Spread Operator
If you want to remove an item from an array and obtain both the modified array and the removed item, you can use splice()
in combination with the spread operator.
const numbers = [1, 2, 3, 4, 5];
const indexToRemove = 2; // Index of the item to remove
const removedItem = numbers.splice(indexToRemove, 1);
console.log(numbers); // Output: [1, 2, 4, 5]
console.log(removedItem); // Output: [3]
In this example, the splice()
method removes the item at indexToRemove
and returns an array containing the removed item, which we store in the removedItem
variable.
0 Comment