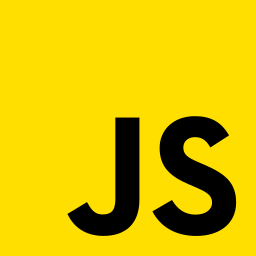
In JavaScript, you can remove a specific item from an array using various array methods.
Using Array.filter()
The Array.filter()
method creates a new array with all elements that pass the test implemented by the provided function. To remove a specific item, you can use Array.filter()
to exclude it from the new array.
Example
const array = [1, 2, 3, 4, 5];
const itemToRemove = 3;
const filteredArray = array.filter((item) => item !== itemToRemove);
console.log(filteredArray); // Output: [1, 2, 4, 5]
Using Array.splice()
The Array.splice()
method changes the contents of an array by removing or replacing existing elements. To remove a specific item, you can find its index using Array.indexOf()
and then use Array.splice()
to remove it.
Example
const array = [1, 2, 3, 4, 5];
const itemToRemove = 3;
const indexToRemove = array.indexOf(itemToRemove);
if (indexToRemove !== -1) {
array.splice(indexToRemove, 1);
}
console.log(array); // Output: [1, 2, 4, 5]
Using Array.indexOf() and Array.slice()
Similarly to the previous method, you can use Array.indexOf()
to find the index of the item to remove, and then use Array.slice()
to create a new array without that item.
Example
const array = [1, 2, 3, 4, 5];
const itemToRemove = 3;
const indexToRemove = array.indexOf(itemToRemove);
const newArray = indexToRemove !== -1 ? array.slice(0, indexToRemove).concat(array.slice(indexToRemove + 1)) : array;
console.log(newArray); // Output: [1, 2, 4, 5]
Using Array.reduce()
The Array.reduce()
method can also be used to remove a specific item from an array. It iterates through the array and accumulates elements into a new array, excluding the item to remove during the accumulation process.
Example
const array = [1, 2, 3, 4, 5];
const itemToRemove = 3;
const newArray = array.reduce((acc, item) => {
if (item !== itemToRemove) {
acc.push(item);
}
return acc;
}, []);
console.log(newArray); // Output: [1, 2, 4, 5]
By using Array.filter()
, Array.splice()
, Array.indexOf()
with Array.slice()
, or Array.reduce()
, you can effectively remove a specific item from an array in JavaScript, depending on your specific needs and preference.
0 Comment