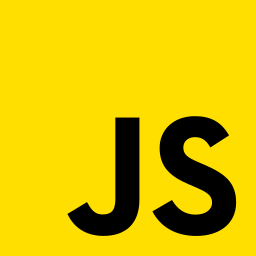
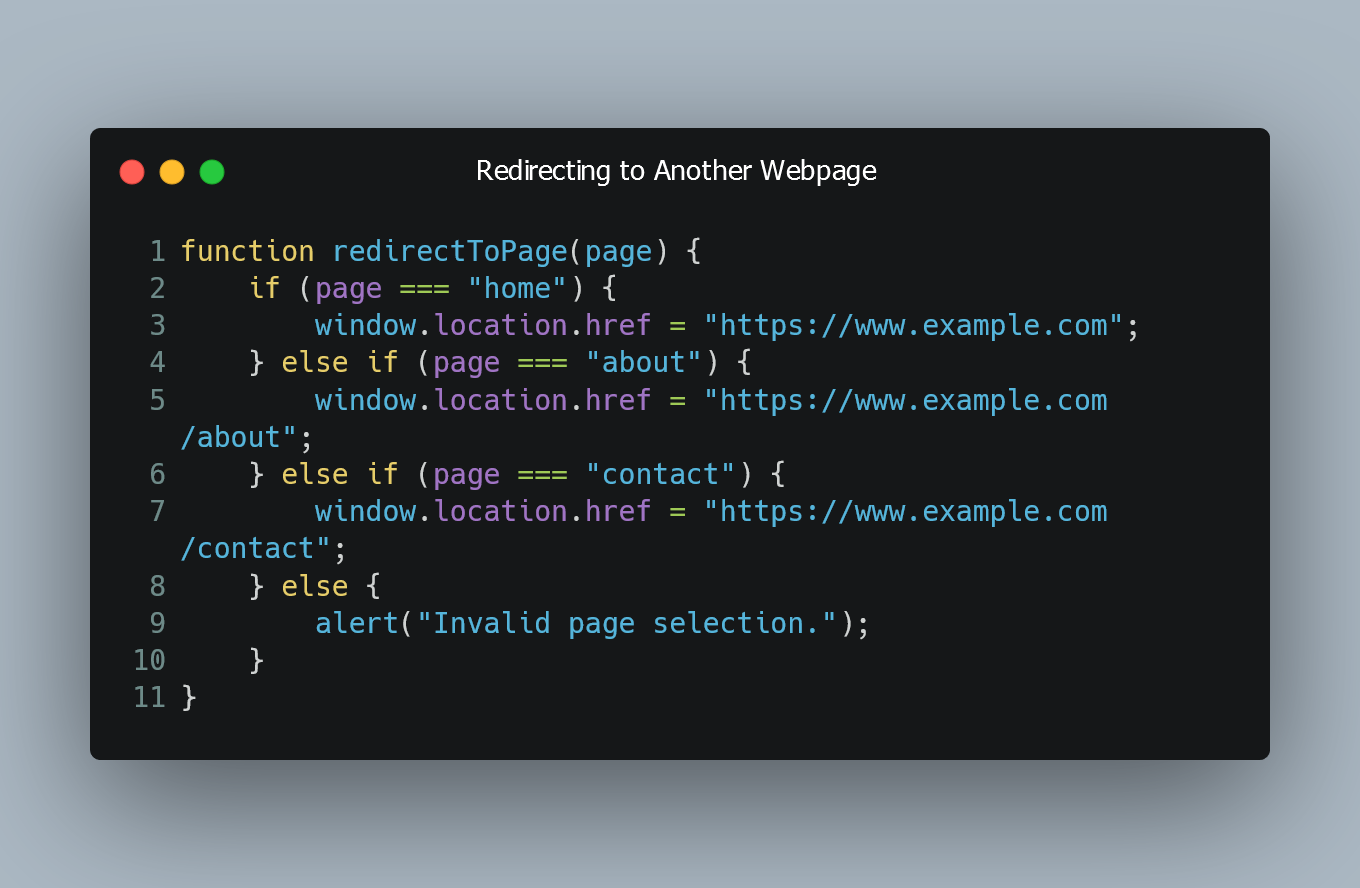
To redirect to another webpage in JavaScript, you can use the window.location
object to set the new URL.
Using window.location.href
The simplest and most common way to redirect to another webpage is by setting the window.location.href
property to the desired URL.
Example
// Redirect to a new webpage
window.location.href = "https://www.example.com";
Using window.location.replace()
Alternatively, you can use the window.location.replace()
method to perform a redirection. This method works similarly to window.location.href
, but it does not add a new entry to the browser's history, making it suitable for one-time redirects.
Example
// Redirect to a new webpage using replace()
window.location.replace("https://www.example.com");
Using window.location.assign()
The window.location.assign()
method is yet another way to redirect to a new webpage. It is similar to window.location.href
and window.location.replace()
in functionality.
Example
// Redirect to a new webpage using assign()
window.location.assign("https://www.example.com");
Delaying the Redirect
If you want to add a delay before the redirection takes place, you can use the setTimeout()
function.
Example
// Redirect after 3 seconds
setTimeout(() => {
window.location.href = "https://www.example.com";
}, 3000); // 3000 milliseconds (3 seconds)
Redirecting Based on User Input or Conditions
You can also redirect based on user input or conditions using any of the mentioned methods. For instance, you might redirect a user to different pages based on their actions or choices.
Example
function redirectToPage(page) {
if (page === "home") {
window.location.href = "https://www.example.com";
} else if (page === "about") {
window.location.href = "https://www.example.com/about";
} else if (page === "contact") {
window.location.href = "https://www.example.com/contact";
} else {
alert("Invalid page selection.");
}
}
By using window.location.href
, window.location.replace()
, or window.location.assign()
, you can effectively redirect to another webpage in JavaScript.
0 Comment