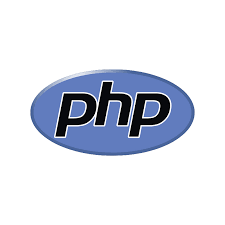
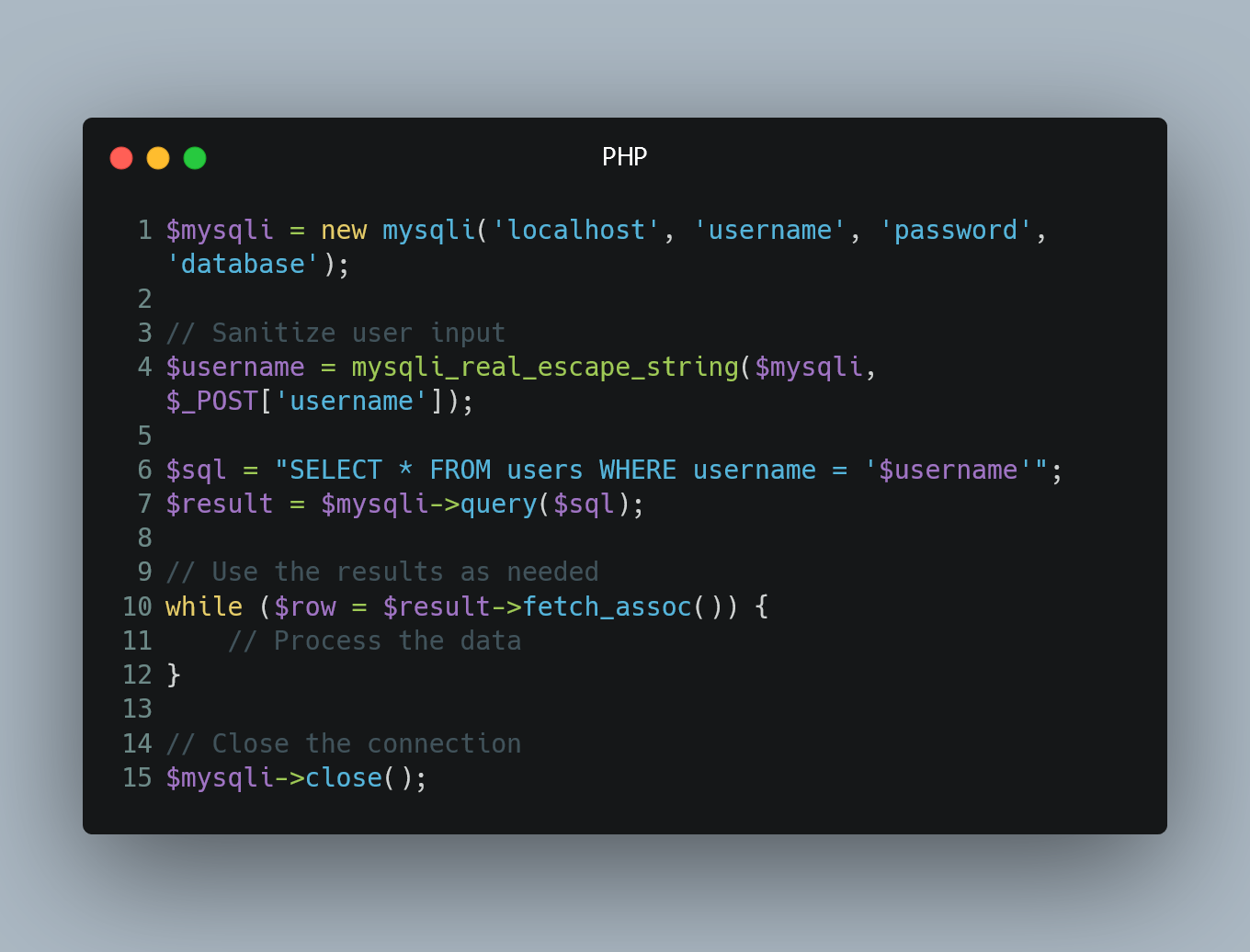
In PHP, it is crucial to protect your database from SQL injection, a common security vulnerability that allows malicious users to manipulate SQL queries.
Using Prepared Statements
What are Prepared Statements?
Prepared statements are a feature provided by most database management systems. They allow you to separate SQL code from user input, reducing the risk of SQL injection attacks.
How to Use Prepared Statements
Here's an example of using prepared statements with PHP and MySQLi:
// Establish a database connection
$mysqli = new mysqli('localhost', 'username', 'password', 'database');
// Prepare a SQL statement with a placeholder for the user input
$stmt = $mysqli->prepare("SELECT * FROM users WHERE username = ?");
// Bind the user input to the placeholder
$stmt->bind_param("s", $username);
// Set the user input
$username = $_POST['username'];
// Execute the statement
$stmt->execute();
// Fetch the results
$result = $stmt->get_result();
// Use the results as needed
while ($row = $result->fetch_assoc()) {
// Process the data
}
// Close the statement and connection
$stmt->close();
$mysqli->close();
Sanitizing User Input
What is Sanitization?
Sanitization involves cleaning and validating user input before using it in SQL queries. Though it is not a substitute for prepared statements, it can add an extra layer of protection.
How to Sanitize User Input
One way to sanitize user input is by using the mysqli_real_escape_string
function:
// Establish a database connection
$mysqli = new mysqli('localhost', 'username', 'password', 'database');
// Sanitize user input
$username = mysqli_real_escape_string($mysqli, $_POST['username']);
// Construct the SQL query
$sql = "SELECT * FROM users WHERE username = '$username'";
// Execute the query
$result = $mysqli->query($sql);
// Use the results as needed
while ($row = $result->fetch_assoc()) {
// Process the data
}
// Close the connection
$mysqli->close();
While sanitization can help prevent some SQL injection attacks, it is not foolproof. Prepared statements should always be your primary defense against SQL injection.
0 Comment