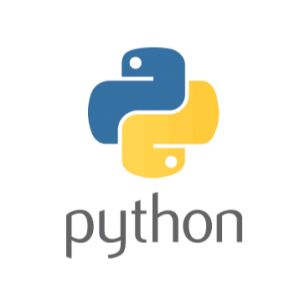
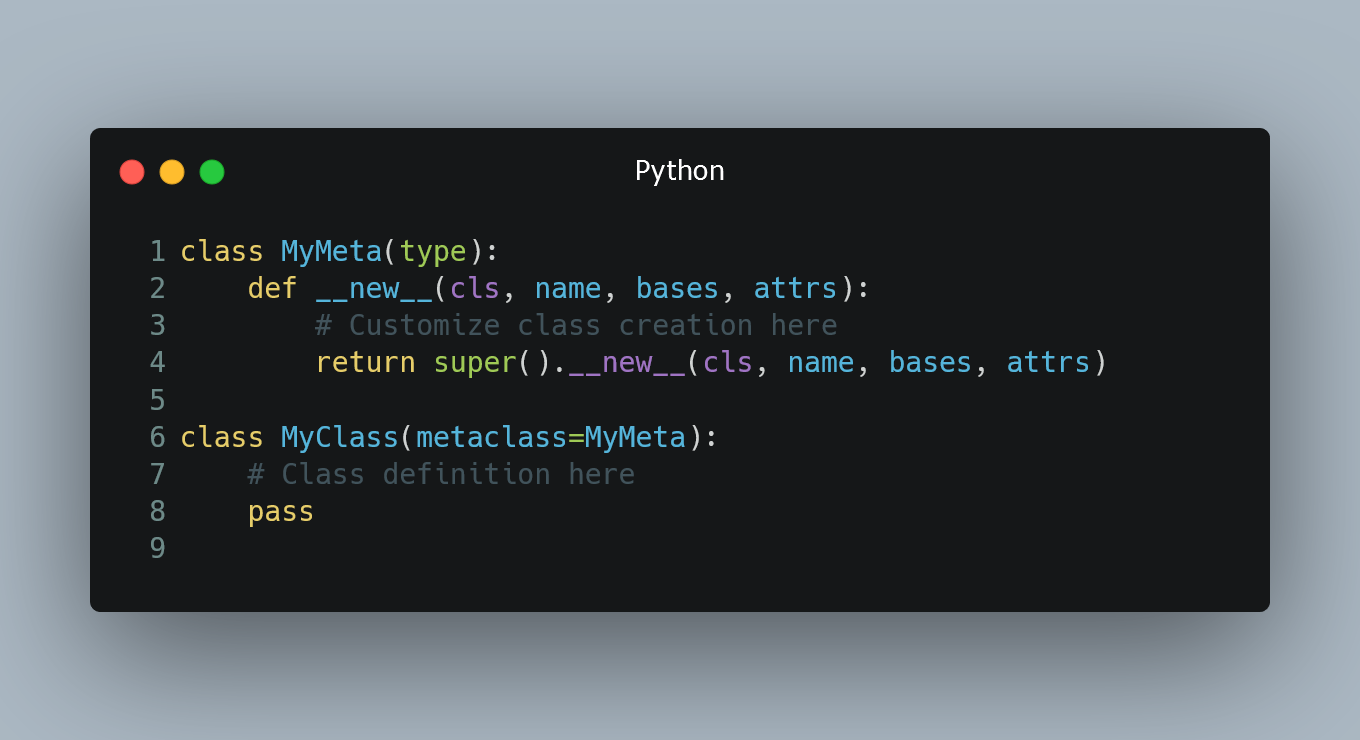
Metaclasses are a powerful and advanced concept in Python that allow you to control the behavior of class creation.
#1. What are Metaclasses?
In Python, everything is an object, including classes. A metaclass is the class of a class, meaning it is responsible for creating and defining classes. When you define a new class in Python, it is actually an instance of its metaclass.
#2. How to Define a Metaclass?
To define a metaclass, you need to create a class that subclasses type
. The metaclass can then override certain methods to customize class creation. The most commonly used method for this purpose is __new__
, which is responsible for creating the class.
class MyMeta(type):
def __new__(cls, name, bases, attrs):
# Customize class creation here
return super().__new__(cls, name, bases, attrs)
class MyClass(metaclass=MyMeta):
# Class definition here
pass
In this example, MyMeta
is the metaclass, and MyClass
is a class that uses MyMeta
as its metaclass.
#3. What Can You Do with Metaclasses?
Metaclasses offer a wide range of possibilities, such as:
-
Modifying Class Attributes: You can alter class attributes, methods, or add new ones before the class is created.
-
Enforcing Coding Standards: Metaclasses can enforce coding standards and conventions by inspecting the class definition and raising errors if certain rules are not followed.
-
Singleton Pattern: You can implement the Singleton design pattern using a metaclass to ensure a class has only one instance.
-
Automatic Property Generation: Metaclasses can automatically generate properties or methods based on class attributes.
#4. Example: A Simple Singleton Metaclass
Here's a basic example of implementing the Singleton pattern using a metaclass:
class SingletonMeta(type):
_instances = {}
def __call__(cls, *args, **kwargs):
if cls not in cls._instances:
cls._instances[cls] = super().__call__(*args, **kwargs)
return cls._instances[cls]
class SingletonClass(metaclass=SingletonMeta):
pass
In this example, SingletonMeta
is the metaclass responsible for creating SingletonClass
instances. The metaclass ensures that only one instance of SingletonClass
exists.
#5. Caution with Metaclasses
Metaclasses can be powerful but are also complex and may lead to obscure code. Use them judiciously and consider more straightforward alternatives if possible.
0 Comment