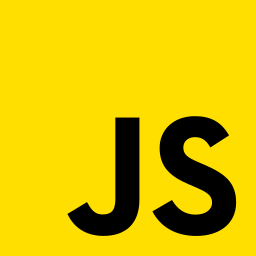
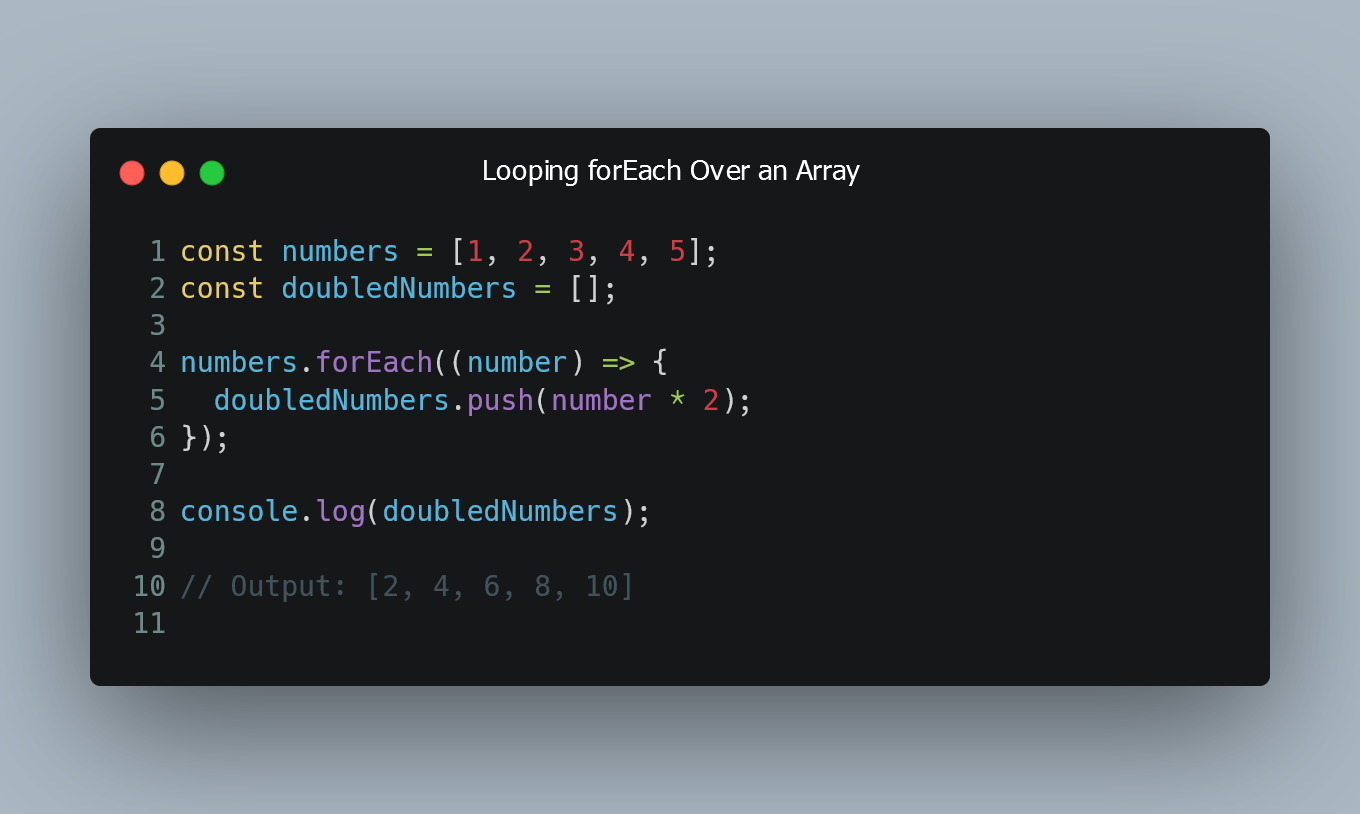
In JavaScript, you can use the forEach
method to loop over the elements of an array and perform an operation on each element.
Using forEach Loop
The forEach
method is available on arrays in JavaScript, and it allows you to execute a callback function for each element in the array.
Syntax
array.forEach(callbackFunction);
Example
const numbers = [1, 2, 3, 4, 5];
numbers.forEach((number) => {
console.log(number);
});
In this example, the forEach
loop iterates over the numbers
array and logs each element to the console.
Accessing Index and Full Array
You can also access the index and the full array during the forEach
iteration by providing additional parameters to the callback function.
Example
const fruits = ['apple', 'banana', 'orange'];
fruits.forEach((fruit, index, array) => {
console.log(`Fruit: ${fruit}, Index: ${index}, Array: [${array}]`);
});
In this example, the callback function receives three parameters: fruit
(the current element), index
(the current index), and array
(the original array). The function logs information about each fruit, its index, and the entire array.
Modifying the Original Array
The forEach
loop only iterates over the array without modifying it. If you need to modify the original array, you might consider using other loop types, such as for
, map
, or reduce
.
Example
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = [];
numbers.forEach((number) => {
doubledNumbers.push(number * 2);
});
console.log(doubledNumbers); // Output: [2, 4, 6, 8, 10]
In this example, the forEach
loop is used to double each number in the numbers
array and store the modified elements in a new array called doubledNumbers
.
0 Comment