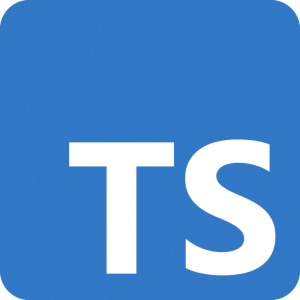
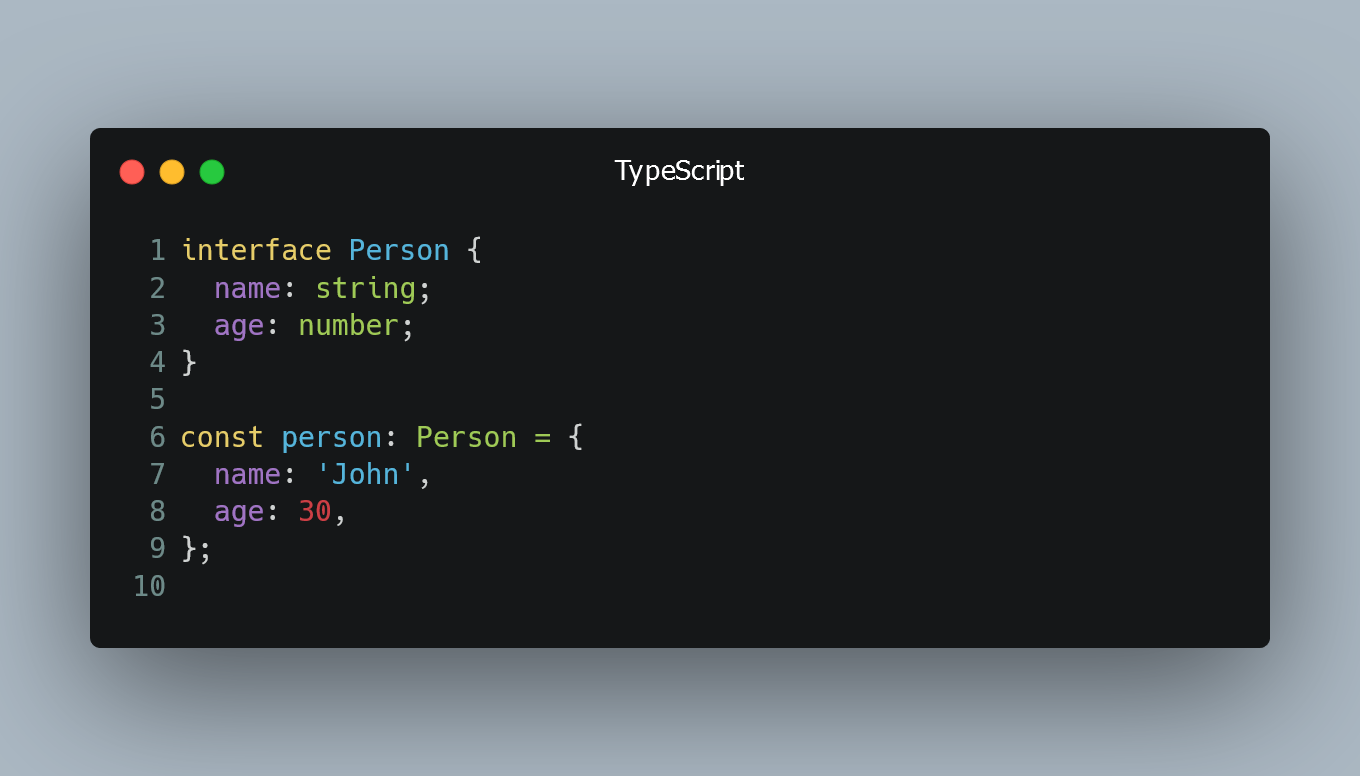
In TypeScript, both interfaces and types are used to define custom data structures and enforce type checking. They are often used interchangeably, but there are some differences between them.
#1. Interfaces
Interfaces are a way to define object shapes and contracts in TypeScript. They are commonly used for defining the structure of objects and ensuring that an object meets specific requirements.
interface Person {
name: string;
age: number;
}
const person: Person = {
name: 'John',
age: 30,
};
In this example, we define an interface called Person
, specifying that objects of this type must have a name
property of type string
and an age
property of type number
. The variable person
must conform to the Person
interface, ensuring that it contains the required properties.
#2. Types
Types in TypeScript provide a more general way to define custom types, not just for objects. They can be used for defining unions, intersections, and other complex types.
type UserRole = 'admin' | 'editor' | 'viewer';
type User = {
name: string;
age: number;
role: UserRole;
};
In this example, we create a type UserRole
, which is a union of three string literals: 'admin'
, 'editor'
, and 'viewer'
. Then, we define a type User
, which consists of three properties: name
of type string
, age
of type number
, and role
of type UserRole
.
#3. Extending Interfaces and Intersection Types
One of the key differences between interfaces and types is the ability to extend interfaces and create intersection types.
interface Shape {
color: string;
}
interface Circle extends Shape {
radius: number;
}
type FilledCircle = Circle & { filled: boolean };
In this example, we create an interface Shape
with a color
property. We then define an interface Circle
that extends Shape
and adds a radius
property. Finally, we create a type FilledCircle
, which is an intersection of Circle
and an object with a filled
property of type boolean
.
#4. Declaration Merging
Interfaces support declaration merging, allowing you to extend existing interfaces and merge them into a single definition.
interface Car {
brand: string;
}
interface Car {
model: string;
}
const myCar: Car = {
brand: 'Toyota',
model: 'Corolla',
};
In this example, we have two interface declarations for Car
, but TypeScript merges them into a single definition. This is useful when working with third-party libraries or when you want to add additional properties to an existing interface.
0 Comment