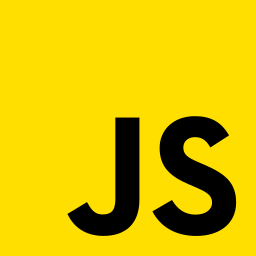
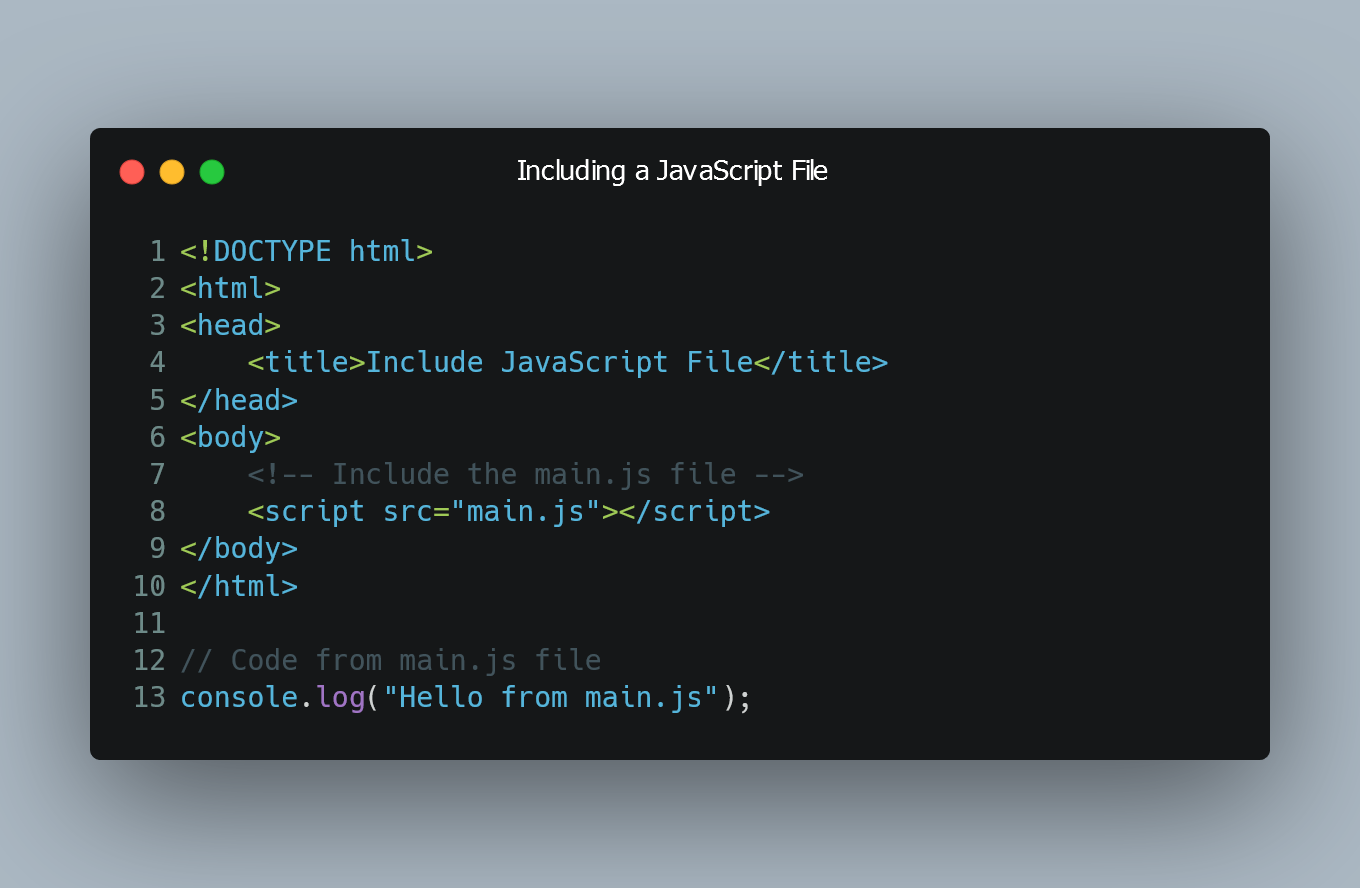
To include a JavaScript file within another JavaScript file, you can use different methods depending on whether you are working in a browser environment or using a module bundler like Node.js.
Using Script Tags in HTML
In a browser environment, you can include a JavaScript file in another JavaScript file by using script tags in your HTML file.
HTML File (index.html)
<!DOCTYPE html>
<html>
<head>
<title>Include JavaScript File</title>
</head>
<body>
<!-- Include the main.js file -->
<script src="main.js"></script>
</body>
</html>
JavaScript File (main.js)
// Code from main.js file
console.log("Hello from main.js");
In this example, the main.js
file is included in the HTML file using the script tag. When the HTML file is loaded in the browser, it will also load and execute the main.js
file.
Using CommonJS Modules (Node.js)
If you are working with Node.js or using a module bundler like Webpack, you can use CommonJS modules to include JavaScript files.
JavaScript File (moduleA.js)
// Code from moduleA.js file
console.log("Hello from moduleA.js");
module.exports = {
message: "Hello from moduleA"
};
JavaScript File (main.js)
// Code from main.js file
const moduleA = require('./moduleA');
console.log(moduleA.message);
In this example, we define a CommonJS module in moduleA.js
and export an object with a message property. In main.js
, we use require()
to include the moduleA.js
file and access its exported value.
Using ES6 Modules (Browser or Bundler)
If you are working in a modern browser environment or using a module bundler with ES6 module support, you can use ES6 modules to include JavaScript files.
JavaScript File (moduleB.js)
// Code from moduleB.js file
console.log("Hello from moduleB.js");
export const message = "Hello from moduleB";
JavaScript File (main.js)
// Code from main.js file
import { message } from './moduleB.js';
console.log(message);
In this example, we define an ES6 module in moduleB.js
using the export
keyword. In main.js
, we use the import
statement to include the moduleB.js
file and access its exported value.
0 Comment