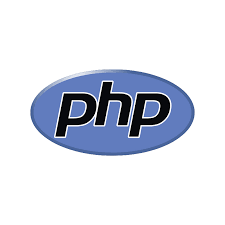
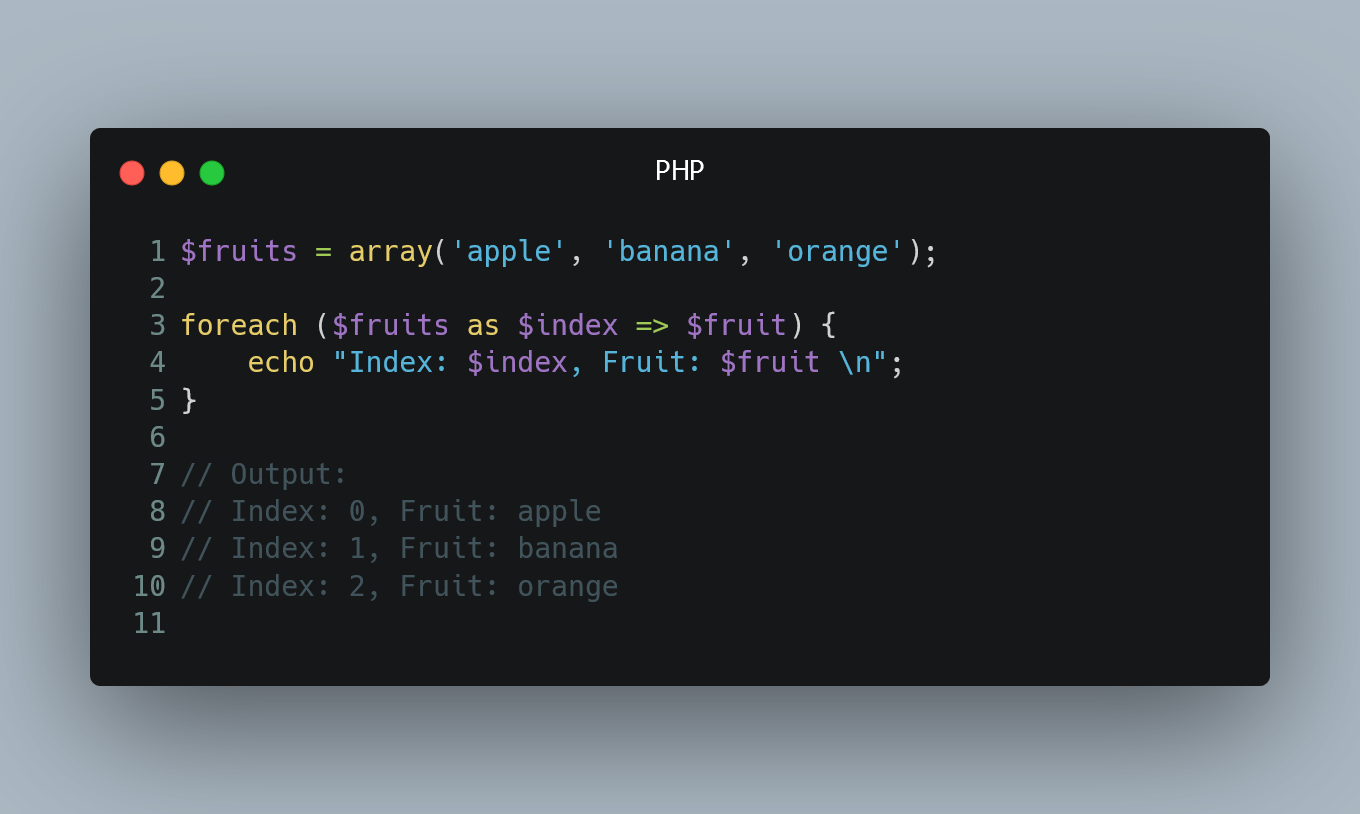
In PHP, the 'foreach' loop provides a convenient way to iterate over arrays and objects.
The Basics of foreach Loop
What is foreach?
The 'foreach' loop is used to loop through elements in an array or items in an object. It simplifies the process of iterating over each element without needing to manage array indexes or object properties manually.
Syntax of foreach
foreach ($arrayOrObject as $key => $value) {
// Code to be executed for each iteration
}
Here, $arrayOrObject
is the array or object you want to iterate over. $key
and $value
are variables that will be assigned the key and value of the current element in each iteration.
Using foreach with Arrays
Example
$fruits = array('apple', 'banana', 'orange');
foreach ($fruits as $index => $fruit) {
echo "Index: $index, Fruit: $fruit \n";
}
Output:
Index: 0, Fruit: apple
Index: 1, Fruit: banana
Index: 2, Fruit: orange
In this example, the 'foreach' loop iterates over the $fruits
array. In each iteration, the $index
variable holds the index of the current element, and the $fruit
variable holds the value of the current element.
Using foreach with Objects
Example
class Person {
public $name;
public $age;
function __construct($name, $age) {
$this->name = $name;
$this->age = $age;
}
}
$person1 = new Person('John', 30);
$person2 = new Person('Jane', 25);
$people = array($person1, $person2);
foreach ($people as $person) {
echo "Name: $person->name, Age: $person->age \n";
}
Output:
Name: John, Age: 30
Name: Jane, Age: 25
In this example, the 'foreach' loop iterates over the $people
array of objects. For each iteration, the $person
variable holds the current object, and we can access its properties using the ->
operator.
0 Comment