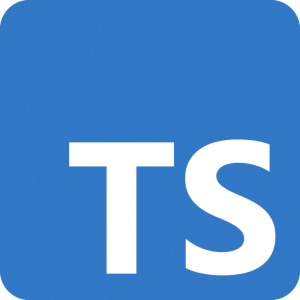
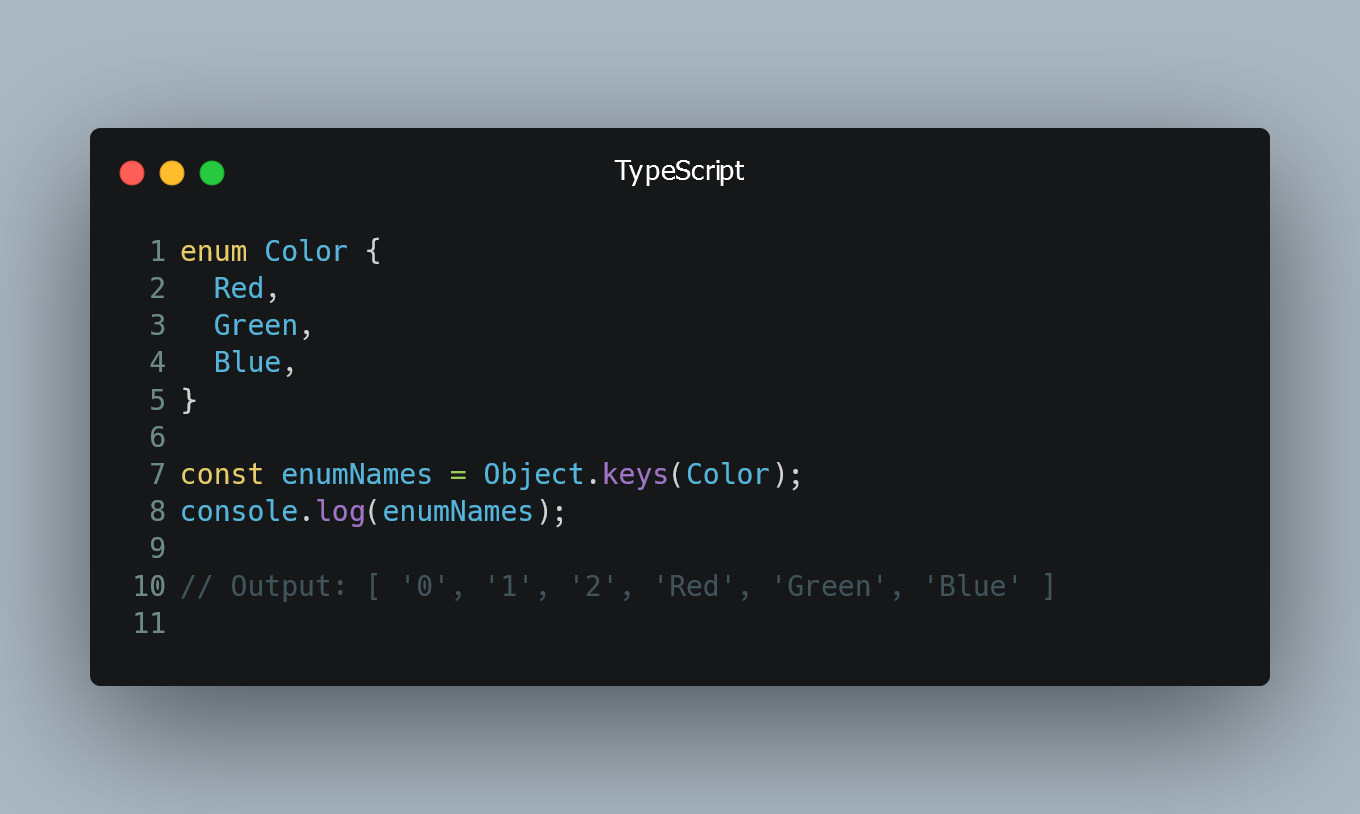
In TypeScript, you can get the names of enum entries (also known as enum keys) programmatically. Enumerations, or enums, are a set of named constants representing discrete values.
#1. Define an Enum
First, define an enum with named entries.
enum Color {
Red,
Green,
Blue,
}
In this example, we define an enum called Color
with three constant values: Red
, Green
, and Blue
. By default, enums are assigned numeric values starting from 0, which corresponds to the index of the enum entries.
#2. Get Names of Enum Entries
To get the names of the enum entries, you can use the Object.keys()
method.
enum Color {
Red,
Green,
Blue,
}
const enumNames = Object.keys(Color);
console.log(enumNames); // Output: [ '0', '1', '2', 'Red', 'Green', 'Blue' ]
In this example, we use Object.keys()
to get all the keys (including numeric keys and enum entry names) of the Color
enum. The result is an array containing the string representation of each key.
#3. Filter Out Numeric Keys
To get only the names of the enum entries (excluding the numeric keys), you can use the filter()
method.
enum Color {
Red,
Green,
Blue,
}
const enumNames = Object.keys(Color).filter((key) => isNaN(Number(key)));
console.log(enumNames); // Output: [ 'Red', 'Green', 'Blue' ]
In this example, we use the filter()
method to remove numeric keys from the array of enum entry names. The isNaN()
function helps us identify non-numeric keys, which correspond to the enum entry names.
#4. Use a Custom Enum with String Values
If you want to define an enum with string values and still get the enum entry names, you can explicitly assign string values to the enum entries.
enum Language {
English = "en",
Spanish = "es",
French = "fr",
}
const enumNames = Object.keys(Language).filter((key) => isNaN(Number(key)));
console.log(enumNames); // Output: [ 'English', 'Spanish', 'French' ]
In this example, we define an enum called Language
with string values. The filter()
method allows us to get only the enum entry names.
0 Comment