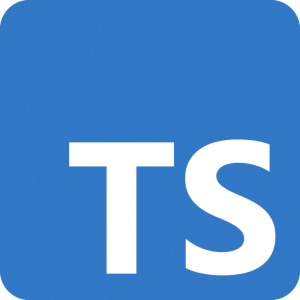
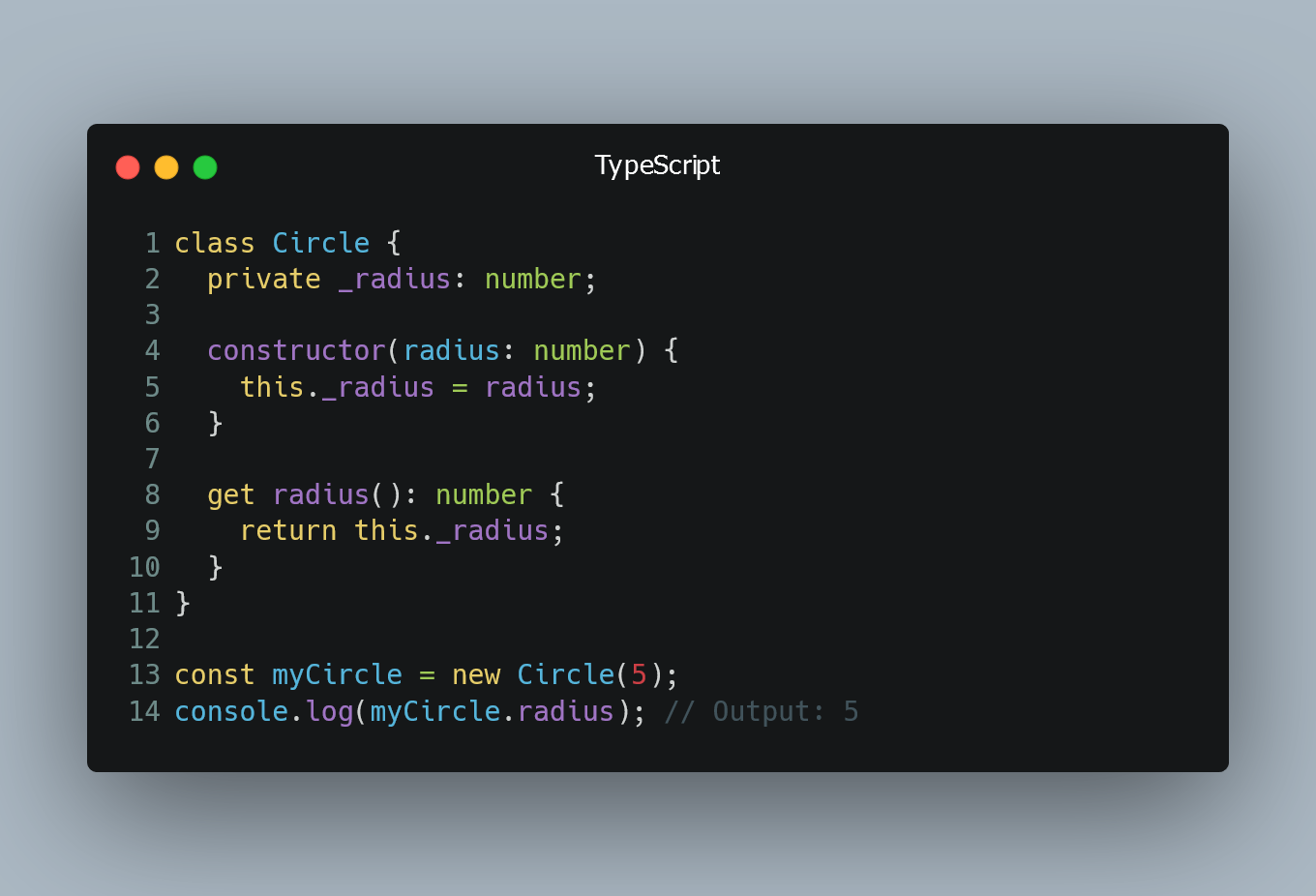
In TypeScript, you can define getter and setter methods for a class to access and modify its private properties. Getters and setters allow you to control how these properties are accessed and manipulated, providing encapsulation and additional logic if needed.
#1. Using Getters
A getter is a method that allows you to retrieve the value of a private property in a class.
class Circle {
private _radius: number;
constructor(radius: number) {
this._radius = radius;
}
get radius(): number {
return this._radius;
}
}
const myCircle = new Circle(5);
console.log(myCircle.radius); // Output: 5
In this example, we have a Circle
class with a private property _radius
. The getter method radius
allows us to access the value of _radius
from outside the class without directly accessing the private property.
#2. Using Setters
A setter is a method that allows you to modify the value of a private property in a class.
class Circle {
private _radius: number;
constructor(radius: number) {
this._radius = radius;
}
get radius(): number {
return this._radius;
}
set radius(newRadius: number) {
if (newRadius >= 0) {
this._radius = newRadius;
} else {
console.error("Radius cannot be negative.");
}
}
}
const myCircle = new Circle(5);
console.log(myCircle.radius); // Output: 5
myCircle.radius = 10;
console.log(myCircle.radius); // Output: 10
myCircle.radius = -3; // Output: "Radius cannot be negative."
console.log(myCircle.radius); // Output: 10 (radius remains unchanged)
In this example, we add a setter method radius
that allows us to modify the value of _radius
. Before assigning the new value, we can perform additional checks or logic to ensure that the new value is valid.
#3. Encapsulation and Logic
Getters and setters are powerful tools for encapsulating private data and providing controlled access to class properties. They allow you to add logic and validation when accessing or modifying private properties, maintaining the integrity of the class's data.
class BankAccount {
private _balance: number;
constructor(initialBalance: number) {
this._balance = initialBalance;
}
get balance(): number {
return this._balance;
}
set balance(newBalance: number) {
if (newBalance >= 0) {
this._balance = newBalance;
} else {
console.error("Balance cannot be negative.");
}
}
deposit(amount: number): void {
this.balance += amount;
}
withdraw(amount: number): void {
if (this.balance >= amount) {
this.balance -= amount;
} else {
console.error("Insufficient balance.");
}
}
}
const myAccount = new BankAccount(1000);
console.log(myAccount.balance); // Output: 1000
myAccount.deposit(500);
console.log(myAccount.balance); // Output: 1500
myAccount.withdraw(2000); // Output: "Insufficient balance."
console.log(myAccount.balance); // Output: 1500 (balance remains unchanged)
In this example, we have a BankAccount
class with private property _balance
, which is accessed and modified using getter and setter methods. The deposit
and withdraw
methods ensure that balance updates are done safely and with proper checks.
0 Comment