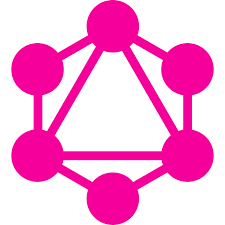
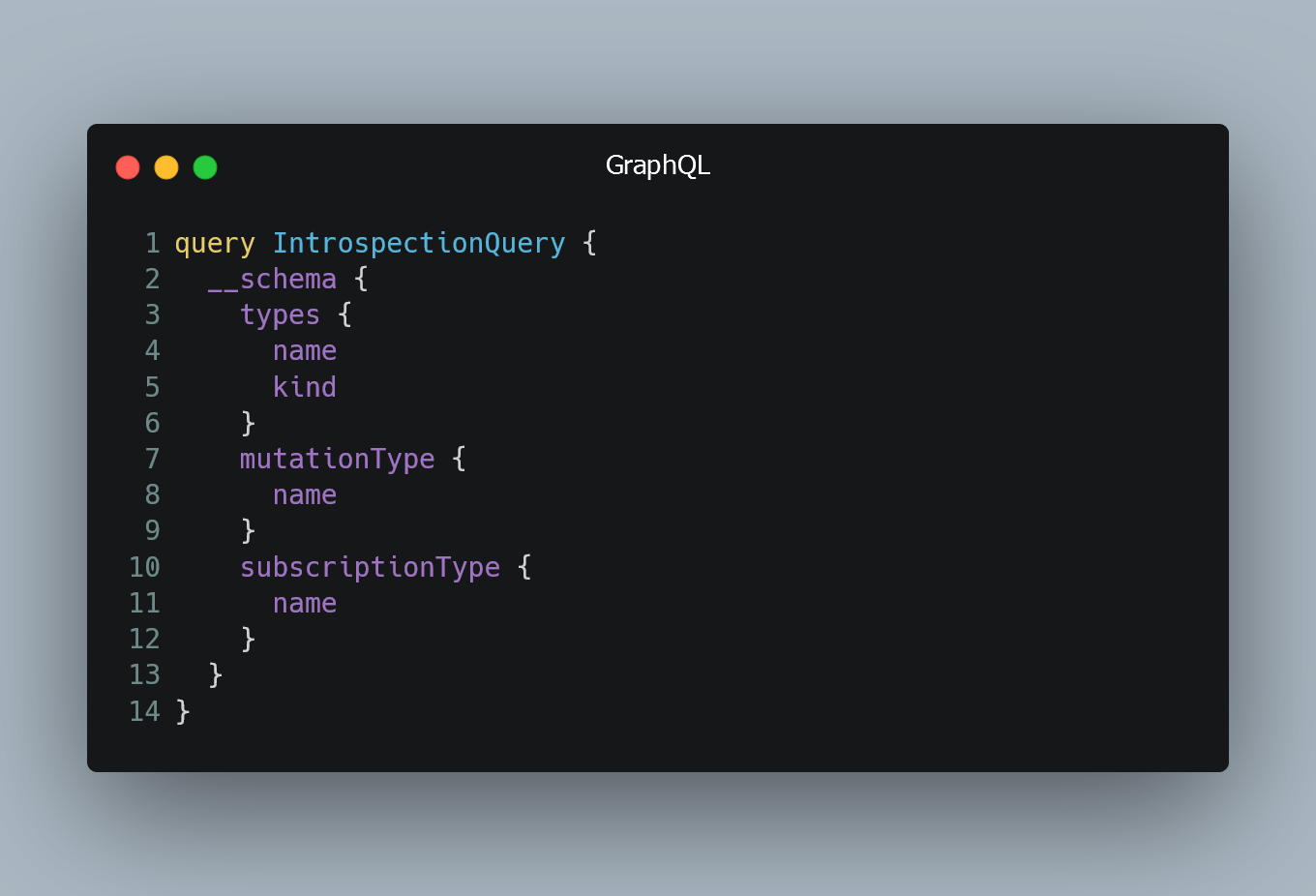
In GraphQL, you can retrieve the entire schema of your API using a special introspection query. This powerful feature allows you to explore and understand the available types, queries, mutations, and subscriptions supported by your GraphQL service.
Understanding Introspection
GraphQL provides a built-in introspection system that enables clients to query the schema itself. Introspection queries return metadata about the GraphQL schema, and this information can be used to build powerful tools like GraphQL clients, documentation generators, and more.
Writing the Introspection Query
To get the whole GraphQL schema, you need to execute an introspection query against your GraphQL endpoint. The introspection query is a special query that starts with the __schema
field, which serves as the entry point for the schema information.
query IntrospectionQuery {
__schema {
types {
name
kind
}
queryType {
name
}
mutationType {
name
}
subscriptionType {
name
}
}
}
Executing the Query
The introspection query can be executed just like any other GraphQL query. Send a POST request to your GraphQL endpoint with the introspection query as the request body.
For example, using the fetch
function in JavaScript:
const endpoint = 'https://your-graphql-api.com/graphql';
fetch(endpoint, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_ACCESS_TOKEN', // If required by your API
},
body: JSON.stringify({
query: `
query IntrospectionQuery {
__schema {
types {
name
kind
}
queryType {
name
}
mutationType {
name
}
subscriptionType {
name
}
}
}
`,
}),
})
.then(response => response.json())
.then(data => console.log(data));
0 Comment