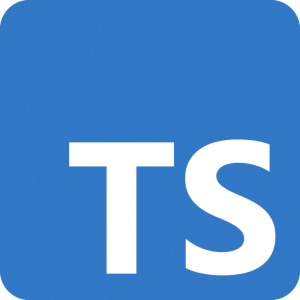
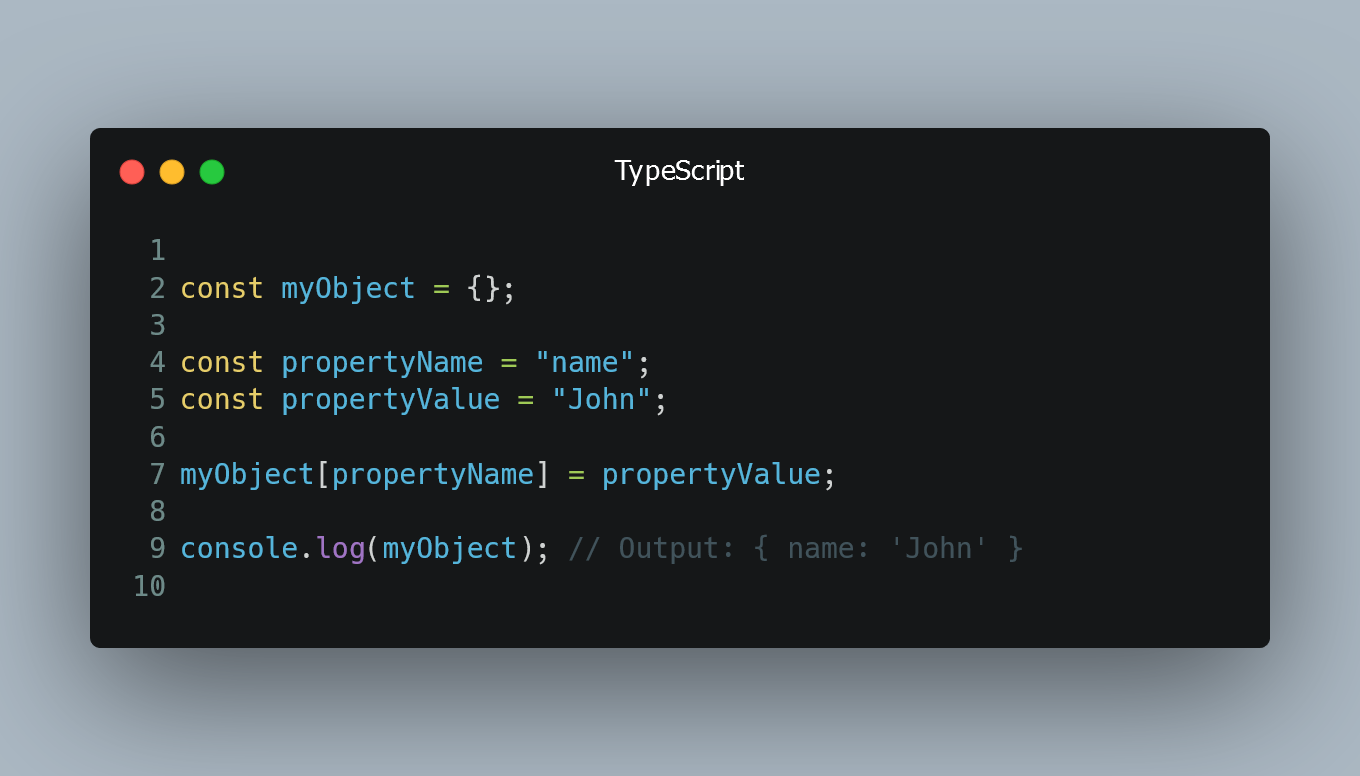
In TypeScript, you can dynamically assign properties to an object using various approaches. Dynamically adding properties allows you to create flexible and extensible data structures based on runtime conditions or user input.
#1. Dot Notation
The simplest way to dynamically add properties to an object is by using dot notation.
const myObject = {};
const propertyName = "name";
const propertyValue = "John";
myObject[propertyName] = propertyValue;
console.log(myObject); // Output: { name: 'John' }
In this example, we create an empty object myObject
. We then define propertyName
and propertyValue
as variables. By using square brackets with the variable propertyName
, we can dynamically assign the propertyValue
to the object.
#2. Square Bracket Notation
You can also use square bracket notation directly during object creation to assign properties dynamically.
const propertyName = "age";
const propertyValue = 30;
const myObject = {
[propertyName]: propertyValue,
};
console.log(myObject); // Output: { age: 30 }
In this example, we use square brackets during object creation to assign the property propertyName
with the value propertyValue
.
#3. Object Spread Operator
The object spread operator allows you to create a new object by merging an existing object with additional properties.
const baseObject = { a: 1, b: 2 };
const additionalProperties = { c: 3, d: 4 };
const myObject = { ...baseObject, ...additionalProperties };
console.log(myObject); // Output: { a: 1, b: 2, c: 3, d: 4 }
In this example, we have two objects: baseObject
and additionalProperties
. By using the object spread operator, we create a new object myObject
that combines properties from both objects.
#4. Object.assign()
The Object.assign()
method can also be used to merge objects and add properties dynamically.
const baseObject = { a: 1, b: 2 };
const additionalProperties = { c: 3, d: 4 };
const myObject = Object.assign({}, baseObject, additionalProperties);
console.log(myObject); // Output: { a: 1, b: 2, c: 3, d: 4 }
In this example, we use Object.assign()
to create a new object myObject
by merging baseObject
and additionalProperties
.
1 Comment
Maps