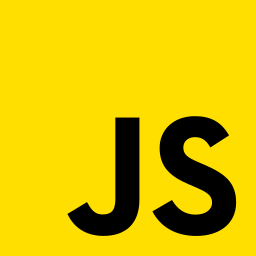
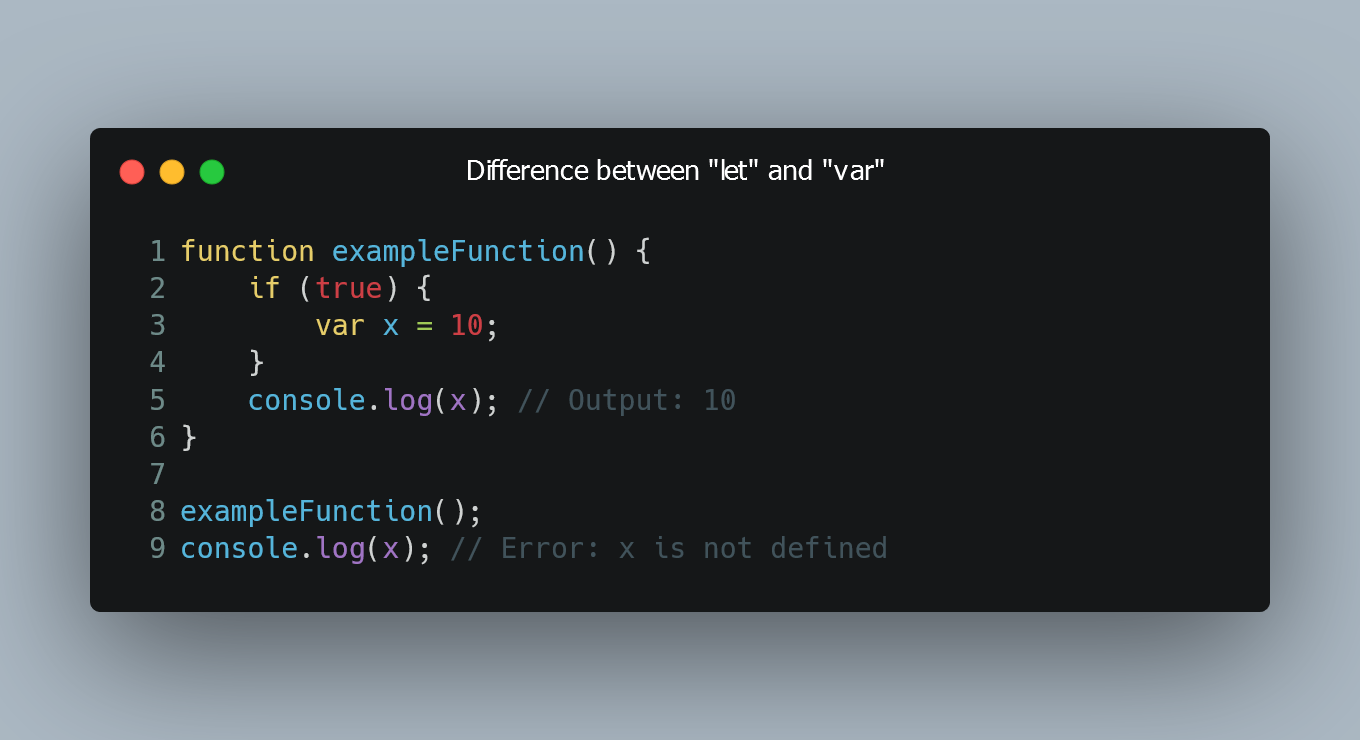
In JavaScript, both "let" and "var" are used for variable declaration, but they have some important differences in terms of scope and hoisting.
Declaration Scope
"var" Declaration Scope
Variables declared with "var" have function scope. This means that they are only accessible within the function where they are declared or at the global scope if declared outside any function.
function exampleFunction() {
if (true) {
var x = 10;
}
console.log(x); // Output: 10
}
exampleFunction();
console.log(x); // Error: x is not defined
"let" Declaration Scope
Variables declared with "let" have block scope. This means that they are only accessible within the block where they are declared, which can be a block inside a function, a loop, or an if statement.
function exampleFunction() {
if (true) {
let y = 20;
}
console.log(y); // Error: y is not defined
}
exampleFunction();
Hoisting
"var" Hoisting
Variables declared with "var" are hoisted to the top of their function or global scope. This means that the variable is effectively moved to the top of its scope during the execution phase, but its assignment is not hoisted.
function exampleFunction() {
console.log(a); // Output: undefined
var a = 5;
console.log(a); // Output: 5
}
"let" Hoisting
Variables declared with "let" are also hoisted, but they are not initialized. This means that you cannot access the variable before the actual declaration in the code.
function exampleFunction() {
console.log(b); // Error: Cannot access 'b' before initialization
let b = 10;
console.log(b); // Output: 10
}
Re-declaration
"var" Re-declaration
Variables declared with "var" can be re-declared within the same scope without any error.
var x = 5;
var x = 10;
console.log(x); // Output: 10
"let" Re-declaration
Variables declared with "let" cannot be re-declared within the same scope. Attempting to re-declare a "let" variable will result in an error.
let y = 15;
let y = 20; // Error: Identifier 'y' has already been declared
console.log(y);
0 Comment