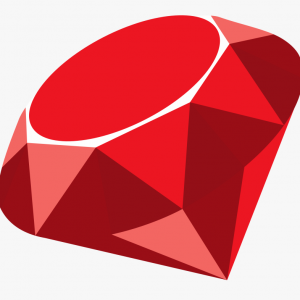
In Ruby, you can easily convert a string to either lower case or upper case using built-in methods.
Converting to Lower Case with downcase
What is downcase?
The downcase
method is used to convert a string to lower case. It returns a new string with all characters converted to their lowercase equivalent.
Syntax of downcase
lower_case_string = original_string.downcase
Example
original_string = "Hello, World!"
lower_case_string = original_string.downcase
puts lower_case_string
In this example, the downcase
method is applied to the original_string
, and the result is printed to the console. The output will be "hello, world!".
Converting to Upper Case with upcase
What is upcase?
The upcase
method is used to convert a string to upper case. It returns a new string with all characters converted to their uppercase equivalent.
Syntax of upcase
upper_case_string = original_string.upcase
Example
original_string = "Hello, World!"
upper_case_string = original_string.upcase
puts upper_case_string
In this example, the upcase
method is applied to the original_string
, and the result is printed to the console. The output will be "HELLO, WORLD!".
Modifying the Original String with downcase! and upcase!
Both downcase
and upcase
methods have destructive counterparts downcase!
and upcase!
, which modify the original string in place.
Example
original_string = "Hello, World!"
original_string.downcase!
puts original_string
In this example, the downcase!
method is applied to the original_string
, modifying it in place. The output will be "hello, world!".
original_string = "Hello, World!"
original_string.upcase!
puts original_string
In this example, the upcase!
method is applied to the original_string
, modifying it in place. The output will be "HELLO, WORLD!".
0 Comment