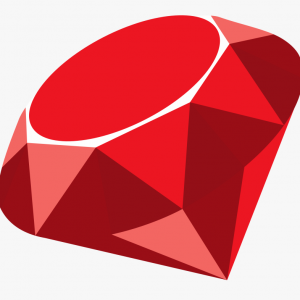
In Ruby, you can easily determine if a string contains a specific substring using built-in methods.
Using the include? Method
What is include?
The include?
method is a simple way to check if a substring exists within a string. It returns true
if the substring is found and false
otherwise.
Syntax of include?
string.include?(substring)
Here, string
is the original string you want to search in, and substring
is the string you want to check for.
Example
sentence = "The quick brown fox jumps over the lazy dog."
puts sentence.include?("fox") # Output: true
puts sentence.include?("cat") # Output: false
In this example, the include?
method is used to check if the substring "fox" and "cat" exist within the sentence
. The first check returns true
, while the second check returns false
.
Using the match Method with Regular Expression
What is match?
The match
method allows you to search for a substring using regular expressions. It returns a MatchData
object if a match is found or nil
if no match is found.
Syntax of match
result = string.match(/regex/)
Here, string
is the original string you want to search in, and /regex/
is the regular expression pattern for the substring you want to find.
Example
sentence = "The quick brown fox jumps over the lazy dog."
result = sentence.match(/fox/)
puts result # Output: #<MatchData "fox">
In this example, the match
method is used with the regular expression /fox/
to find the substring "fox" within the sentence
. The method returns a MatchData
object containing the matched substring.
0 Comment