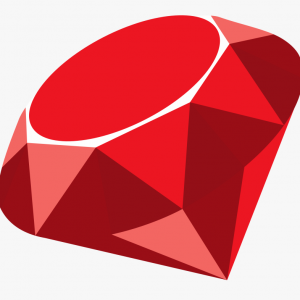
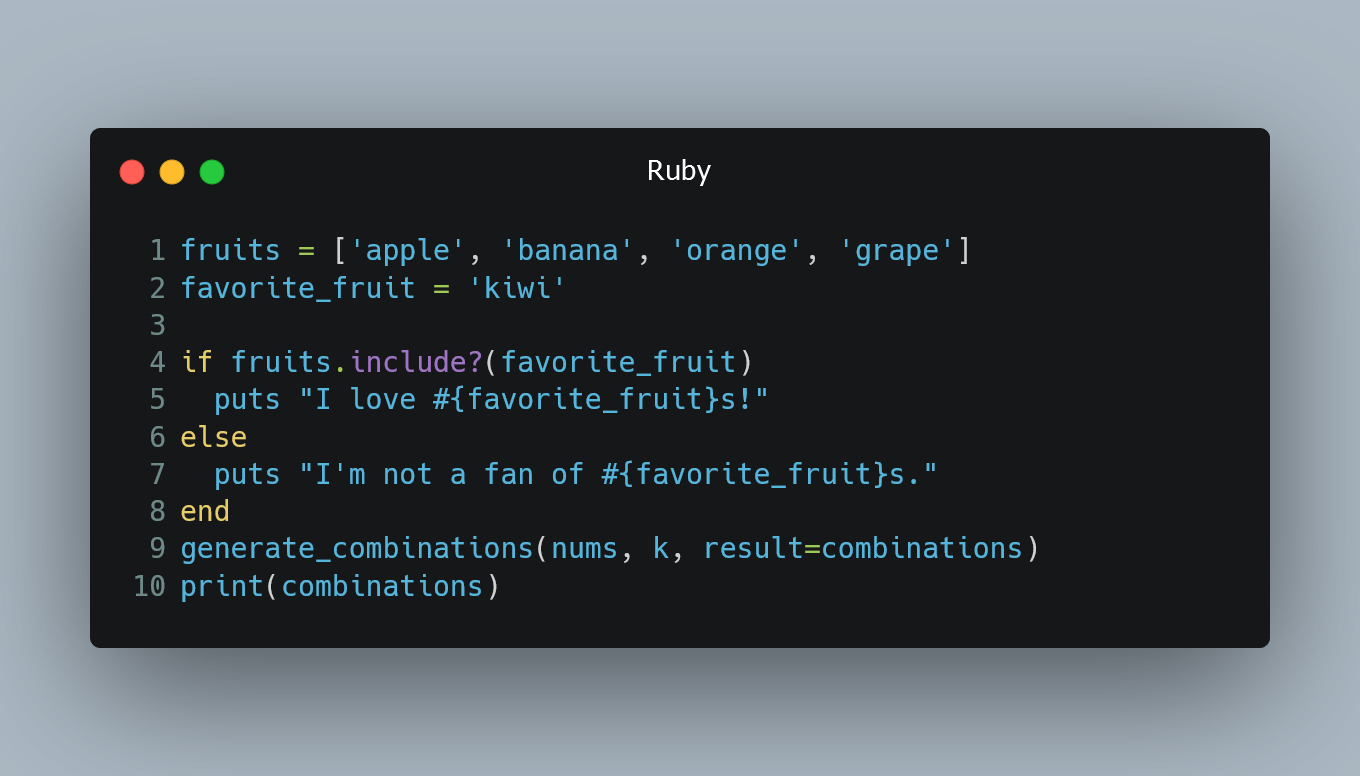
In Ruby, you can easily check if a value exists in an array using built-in methods and operators.
Using the include? Method
What is the include? Method?
The include?
method is a convenient way to check if a specific value exists in an array. It returns true
if the value is found and false
otherwise.
Syntax of include?
The syntax for using the include?
method is as follows:
array.include?(value)
Here, array
is the array you want to search, and value
is the value you want to check for.
Example
fruits = ['apple', 'banana', 'orange', 'grape']
puts fruits.include?('banana') # Output: true
puts fruits.include?('kiwi') # Output: false
In this example, we have an array fruits
containing several fruit names. We use the include?
method to check if 'banana' and 'kiwi' exist in the array. The method returns true
for 'banana' and false
for 'kiwi'.
Using the include? Method with if Statement
You can combine the include?
method with an if
statement to perform conditional checks based on the existence of a value in the array.
Example
fruits = ['apple', 'banana', 'orange', 'grape']
favorite_fruit = 'kiwi'
if fruits.include?(favorite_fruit)
puts "I love #{favorite_fruit}s!"
else
puts "I'm not a fan of #{favorite_fruit}s."
end
In this example, we have a variable favorite_fruit
set to 'kiwi'. We use the include?
method to check if 'kiwi' exists in the fruits
array. Based on the result, the program will print either "I love kiwis!" or "I'm not a fan of kiwis."
0 Comment