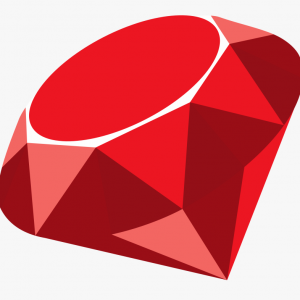
In Ruby, you can execute shell commands and interact with the command-line environment using various methods.
Using backticks (`) or %x
What are backticks?
Backticks or %x()
are a simple way to execute shell commands from within Ruby. They capture the standard output of the command and return it as a string.
Syntax of backticks
output = `command`
Example
result = `ls -l`
puts result
In this example, the ls -l
command is executed using backticks, and the result is printed to the console. The output will be a listing of files and directories in the current directory.
Using system() method
What is system()?
The system()
method allows you to execute shell commands and display their output directly in the terminal.
Syntax of system()
system('command')
Example
status = system('echo Hello, Ruby!')
puts "Command executed successfully!" if status
In this example, the echo Hello, Ruby!
command is executed using the system()
method, and the output is directly displayed in the terminal. The status of the command execution is stored in the status
variable, and if the command is executed successfully, the message "Command executed successfully!" will be printed.
Using backticks with interpolation
You can use string interpolation with backticks to execute dynamic shell commands.
Example
filename = 'example.txt'
result = `cat #{filename}`
puts result
In this example, the content of the file specified by the filename
variable is read using the cat
command and printed to the console.
Using IO.popen
The IO.popen
method allows you to execute shell commands and read their output as a stream.
Example
IO.popen('ls -l') do |io|
puts io.read
end
In this example, the ls -l
command is executed using IO.popen
, and the output is read as a stream and printed to the console.
0 Comment