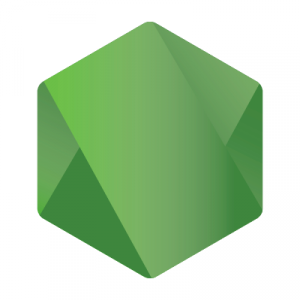
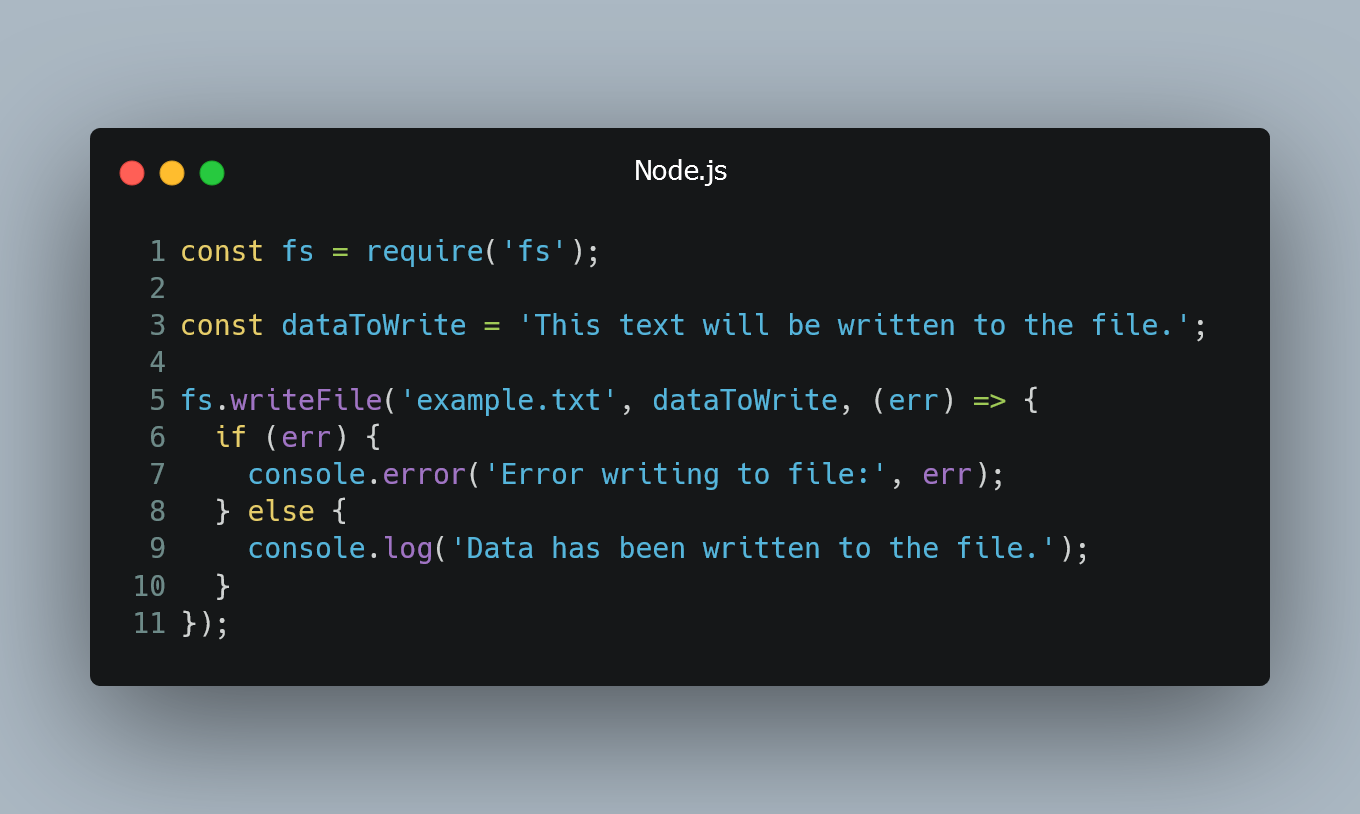
Writing to files in Node.js is a common task when working on server-side applications or handling data storage. Node.js provides built-in modules that make it easy to write data to files.
Using the fs.writeFile() Method
Node.js comes with the fs
module, which provides various methods for interacting with the file system. One of these methods is fs.writeFile()
, which allows you to write data to a file. Here's how you can use it:
const fs = require('fs');
const dataToWrite = 'This text will be written to the file.';
fs.writeFile('example.txt', dataToWrite, (err) => {
if (err) {
console.error('Error writing to file:', err);
} else {
console.log('Data has been written to the file.');
}
});
In this example, we first require the fs
module and create a string called dataToWrite
that contains the text we want to write to the file. Then, we use fs.writeFile()
and pass the file name ('example.txt'), the data to write, and a callback function. The callback function is executed after the write operation is completed, and it checks for any errors.
Using the fs.createWriteStream() Method
Another way to write to files in Node.js is by using the fs.createWriteStream()
method. This method is useful when you want to write a large amount of data or want more control over the writing process.
const fs = require('fs');
const dataToWrite = 'This text will be written using a write stream.';
const writeStream = fs.createWriteStream('example.txt');
writeStream.write(dataToWrite, 'utf8', (err) => {
if (err) {
console.error('Error writing to file:', err);
} else {
console.log('Data has been written to the file.');
writeStream.end();
}
});
In this example, we use fs.createWriteStream()
to create a writable stream to the file 'example.txt'. We then use the write()
method on the stream to write the data to the file. The 'utf8'
argument specifies the encoding of the data. After writing the data, we call end()
on the stream to signal that we have finished writing.
1 Comment
Can you help me