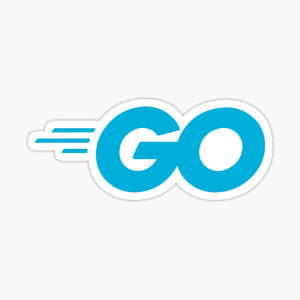
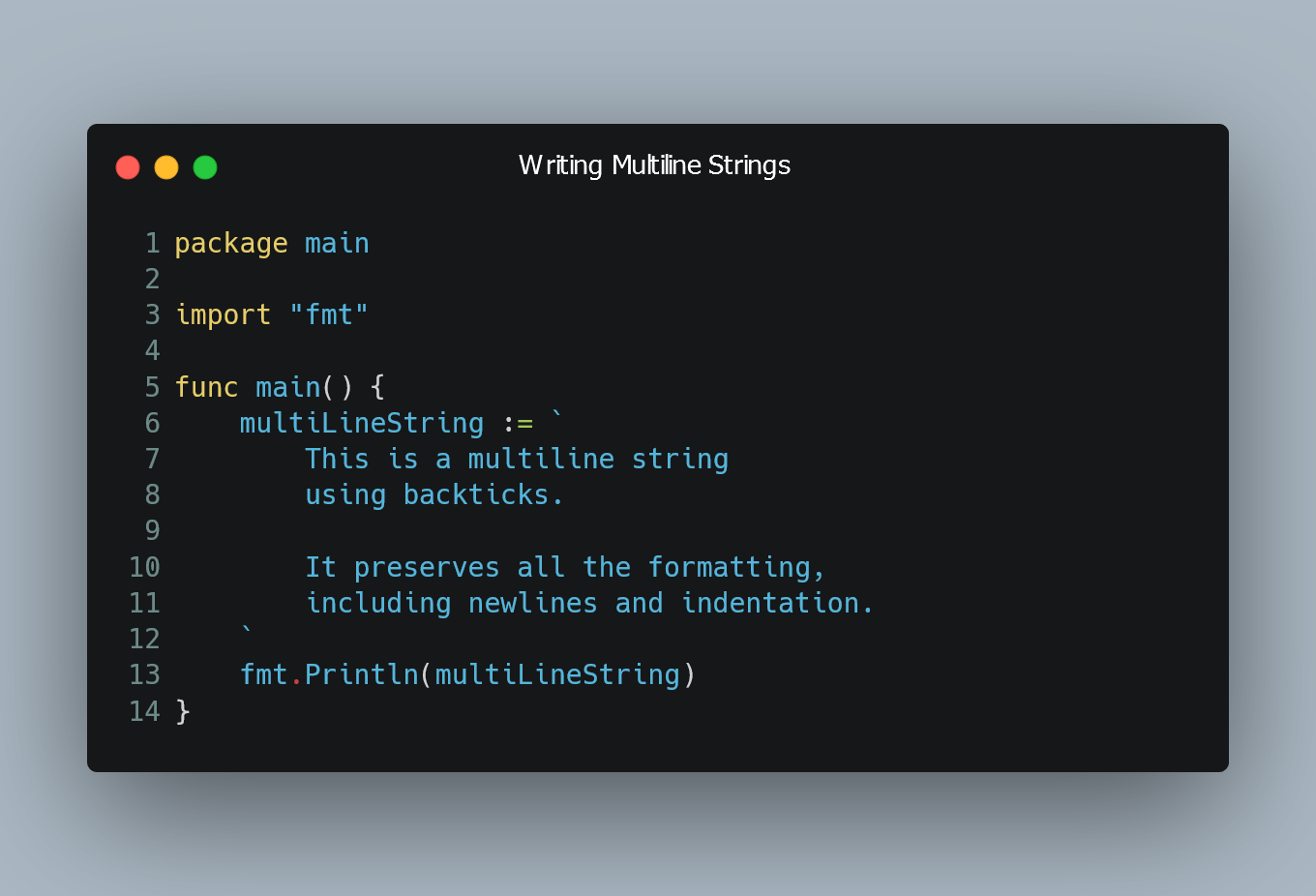
In Go, you can create multiline strings using backticks (``) or the newline escape character (\n).
Using Backticks (``)
Backticks (``) are a convenient way to define multiline strings in Go. When you use backticks to enclose a string, it can span multiple lines without requiring any special escape characters. The string will retain its original formatting, including newlines.
Example
package main
import "fmt"
func main() {
multiLineString := `
This is a multiline string
using backticks.
It preserves all the formatting,
including newlines and indentation.
`
fmt.Println(multiLineString)
}
Using Newline Escape Character (\n)
If you prefer to use double quotes to define strings, you can use the newline escape character (\n) to create multiline strings. The \n escape sequence represents a newline character, which allows you to split the string across multiple lines.
Example
package main
import "fmt"
func main() {
multiLineString := "This is a multiline string\nusing the newline escape character.\n\nIt requires explicit newline characters for line breaks."
fmt.Println(multiLineString)
}
Both methods will result in the same output, producing a multiline string.
0 Comment