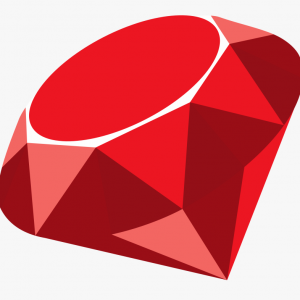
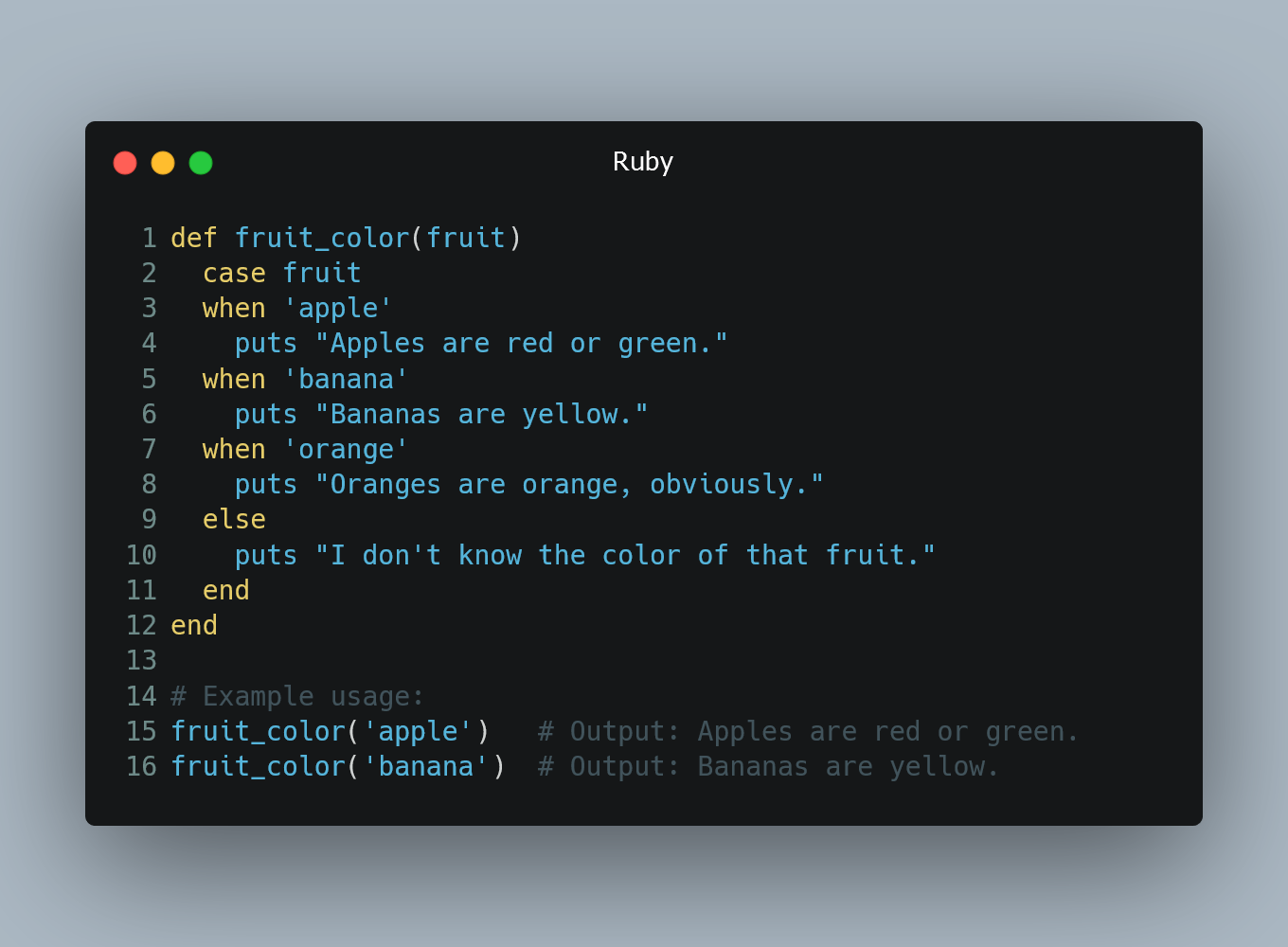
In Ruby, a switch statement is achieved using the case
statement. It allows you to compare the value of an expression against multiple conditions and execute code blocks based on matching conditions.
What is the case Statement?
The case
statement in Ruby is similar to the switch or select-case statements in other programming languages. It evaluates an expression and compares it against multiple when clauses to determine which code block to execute.
Syntax of the case Statement
The basic syntax of the case
statement is as follows:
case expression
when value1
# Code block to execute if expression matches value1
when value2
# Code block to execute if expression matches value2
when value3
# Code block to execute if expression matches value3
else
# Code block to execute if no previous when clause matches
end
The else
clause is optional and is executed when none of the previous when
clauses match the expression.
Example
def fruit_color(fruit)
case fruit
when 'apple'
puts "Apples are red or green."
when 'banana'
puts "Bananas are yellow."
when 'orange'
puts "Oranges are orange, obviously."
else
puts "I don't know the color of that fruit."
end
end
# Example usage:
fruit_color('apple') # Output: Apples are red or green.
fruit_color('banana') # Output: Bananas are yellow.
fruit_color('grape') # Output: I don't know the color of that fruit.
In this example, the fruit_color
method uses a case
statement to determine the color of a given fruit. Depending on the value of the fruit
parameter, the method prints the corresponding message about the color of the fruit. If none of the specified fruits match, the else
clause will be executed.
0 Comment