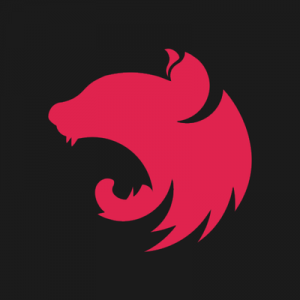
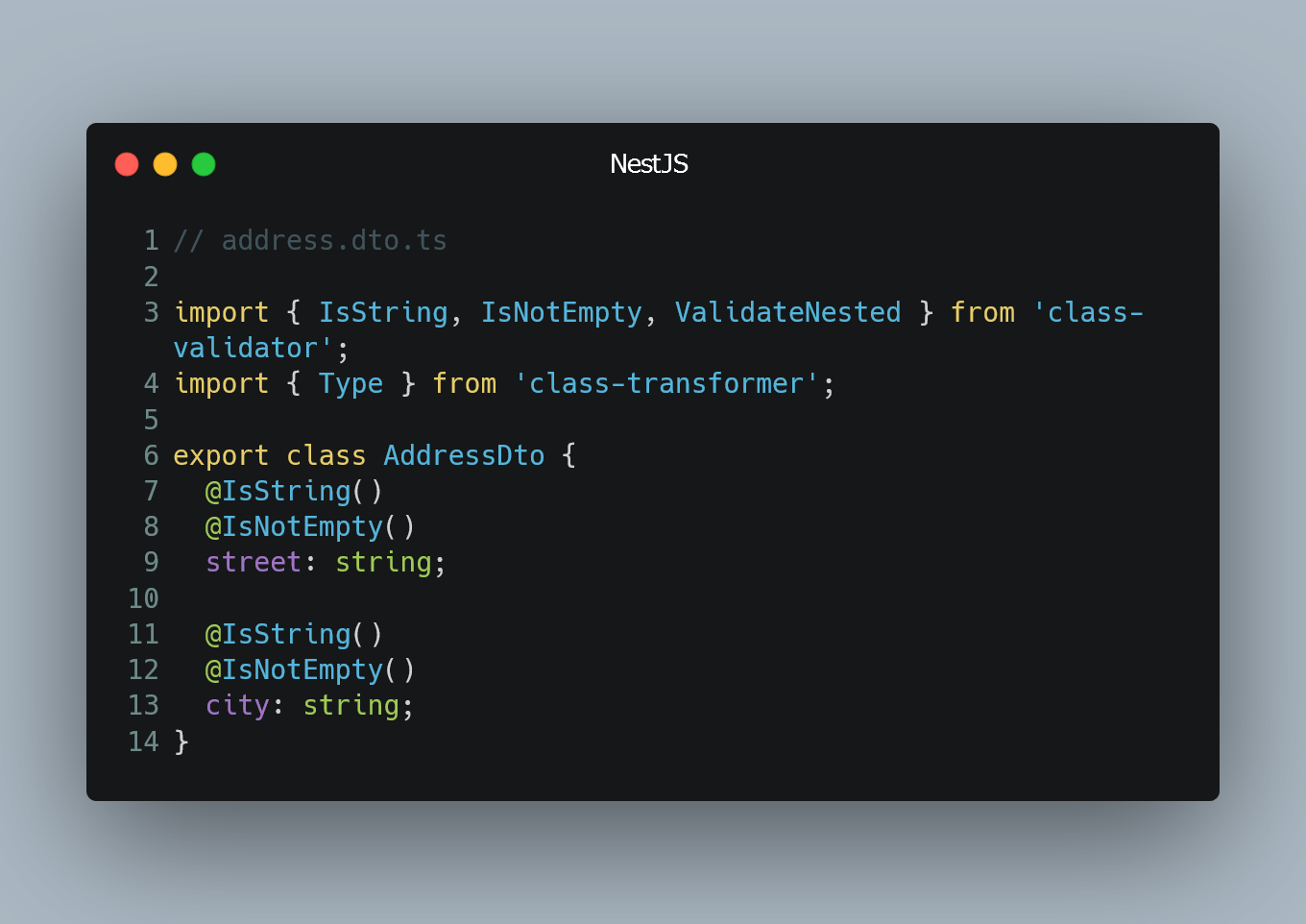
NestJS provides a powerful validation feature that integrates with class-validator to validate incoming data. You can easily validate nested objects in your DTO classes using class-validator decorators.
Setting Up Class Validator
Before we start validating nested objects, ensure that you have class-validator installed in your NestJS project.
npm install class-validator --save
Defining Nested DTO
To validate nested objects, create a DTO class that represents the nested object structure. Use class-validator decorators to add validation rules to the properties.
// address.dto.ts
import { IsString, IsNotEmpty, ValidateNested } from 'class-validator';
import { Type } from 'class-transformer';
export class AddressDto {
@IsString()
@IsNotEmpty()
street: string;
@IsString()
@IsNotEmpty()
city: string;
}
In this example, we create an AddressDto
class with two properties, street
and city
. We use IsString
and IsNotEmpty
decorators to enforce that both properties must be non-empty strings.
Using the Nested DTO in Main DTO
Now, use the nested DTO within the main DTO class and apply the ValidateNested
decorator from class-validator.
// user.dto.ts
import { IsString, IsNotEmpty, ValidateNested } from 'class-validator';
import { Type } from 'class-transformer';
import { AddressDto } from './address.dto';
export class UserDto {
@IsString()
@IsNotEmpty()
name: string;
@IsString()
@IsNotEmpty()
email: string;
@ValidateNested({ each: true })
@Type(() => AddressDto)
address: AddressDto;
}
In this example, we create a UserDto
class with properties name
, email
, and address
. We use the IsString
and IsNotEmpty
decorators for the name
and email
properties, as before.
For the address
property, we use ValidateNested
to indicate that it is a nested object that needs to be validated. The each: true
option is used when the property is an array of nested objects. We also use the Type
decorator to specify the type of the nested object.
Using the DTO in the Controller
Now, you can use the UserDto
in your controller to validate incoming requests with nested objects.
// users.controller.ts
import { Controller, Post, Body } from '@nestjs/common';
import { UserDto } from './user.dto';
@Controller('users')
export class UsersController {
@Post()
createUser(@Body() userDto: UserDto) {
// The userDto is already validated with nested objects
console.log(userDto);
// Your business logic here
}
}
In this example, we use the UserDto
as the @Body()
decorator in the createUser
method of the controller. When a request is made to this endpoint, NestJS automatically validates the incoming data based on the DTO structure.
0 Comment